Efficiently Stream Large JSON Files with RxJava and Jackson
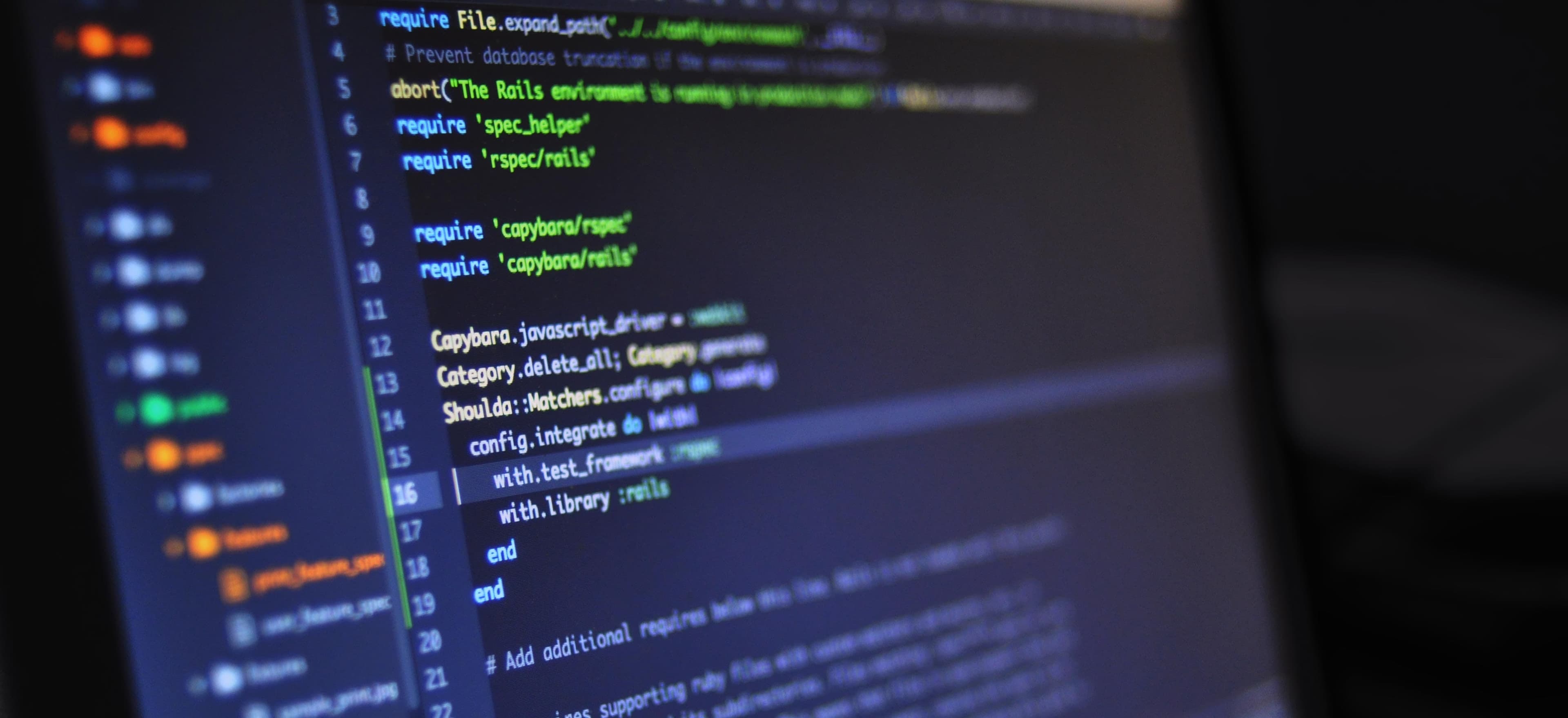
- Published on
Efficiently Stream Large JSON Files with RxJava and Jackson
In the world of software development, managing large datasets is a common challenge. JSON, being one of the most popular data interchange formats, is often used for APIs and configuration files. However, large JSON files can cause memory overflow errors or slow performance if not handled properly. In this blog post, we will explore how to efficiently stream large JSON files using two powerful libraries: RxJava and Jackson.
Why Use RxJava and Jackson?
RxJava
RxJava is a Java VM implementation of Reactive Extensions. It provides a powerful and flexible way to work with asynchronous data streams. One of the major benefits of using RxJava is that it lets you compose asynchronous and event-based programs using observables, allowing for better management of concurrency and a more responsive application.
Jackson
Jackson is a high-performance JSON processor for Java. It is widely used for parsing and generating JSON, making it a natural choice for handling JSON data in Java applications. It offers streaming capabilities that are memory efficient. This means that we can read very large JSON files without loading the entire file into memory, helping to maintain the performance and responsiveness of our applications.
Streaming Large JSON Files
When dealing with large JSON files, traditionally, the entire file is read into memory and then processed. This approach is unsustainable for larger datasets, as it can lead to out-of-memory errors. With the combination of RxJava and Jackson, we can read the JSON file efficiently in a streaming manner.
Setup Your Project
To begin, ensure that your project includes the necessary dependencies in your pom.xml
(for Maven users) or build.gradle
(for Gradle users).
Maven
<dependency>
<groupId>io.reactivex.rxjava2</groupId>
<artifactId>rxjava</artifactId>
<version>2.2.19</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.12.3</version>
</dependency>
Gradle
implementation 'io.reactivex.rxjava2:rxjava:2.2.19'
implementation 'com.fasterxml.jackson.core:jackson-databind:2.12.3'
implementation 'com.fasterxml.jackson.core:jackson-core:2.12.3'
Example: Streaming a Large JSON Array
In this example, we will demonstrate how to stream a large JSON array of objects from a file in a memory-efficient manner.
Step 1: Define the JSON Structure
Let's say we have a JSON file (data.json
) structured as follows:
[
{"id": 1, "name": "John Doe"},
{"id": 2, "name": "Jane Smith"},
...
]
Step 2: Create a Class to Represent the Data
We need a class to match the structure of our JSON objects. Here’s a simple model class named Person
:
public class Person {
private int id;
private String name;
// Getters and Setters
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
Step 3: Implement Streaming Logic
Now, let’s create a method to read and process the JSON file using RxJava and Jackson:
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.reactivex.Observable;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
public class JsonStreamExample {
public static Observable<Person> streamJsonFile(String filePath) {
ObjectMapper objectMapper = new ObjectMapper(new JsonFactory());
File file = new File(filePath);
return Observable.create(emitter -> {
try {
JsonNode rootNode = objectMapper.readTree(file);
Iterator<JsonNode> iterator = rootNode.elements();
while (iterator.hasNext()) {
JsonNode node = iterator.next();
Person person = objectMapper.treeToValue(node, Person.class);
emitter.onNext(person);
}
emitter.onComplete();
} catch (IOException e) {
emitter.onError(e);
}
});
}
}
Explanation of the Code
1. Observable Creation
Observable.create()
: We utilize this method to create an observable that emits items. It takes a lambda expression to define how we want to push items.
2. Reading the File
objectMapper.readTree(file)
: This reads the JSON file into a JsonNode. Using Jackson's streaming capabilities allows us to handle large files without exhausting memory.
3. Emitting Items
-
emitter.onNext(person)
: Each instance of thePerson
object is emitted. -
emitter.onComplete()
: Signals that all items have been emitted.
Step 4: Consume the Stream
Now that we have our observable set up, let's see how to consume it:
public static void main(String[] args) {
JsonStreamExample.streamJsonFile("data.json")
.subscribe(
person -> System.out.println("Person ID: " + person.getId() + ", Name: " + person.getName()),
throwable -> System.err.println("Error: " + throwable.getMessage()),
() -> System.out.println("All data processed.")
);
}
Explanation of Subscription
subscribe()
: We attach observers to our observable. It takes three parameters:- A consumer for the emitted
Person
objects. - An error handler for potential exceptions.
- A completion handler once all items are processed.
- A consumer for the emitted
Final Thoughts
By combining RxJava with Jackson, we have created a robust mechanism for processing large JSON files efficiently. Adopting this streaming approach can significantly reduce memory consumption and enhance performance in your applications.
For more information, you might find these links helpful:
Utilize these libraries wisely, and you'll be able to handle large datasets with ease. Happy coding!