Mastering Many-to-Many Mapping in Hibernate: A Guide
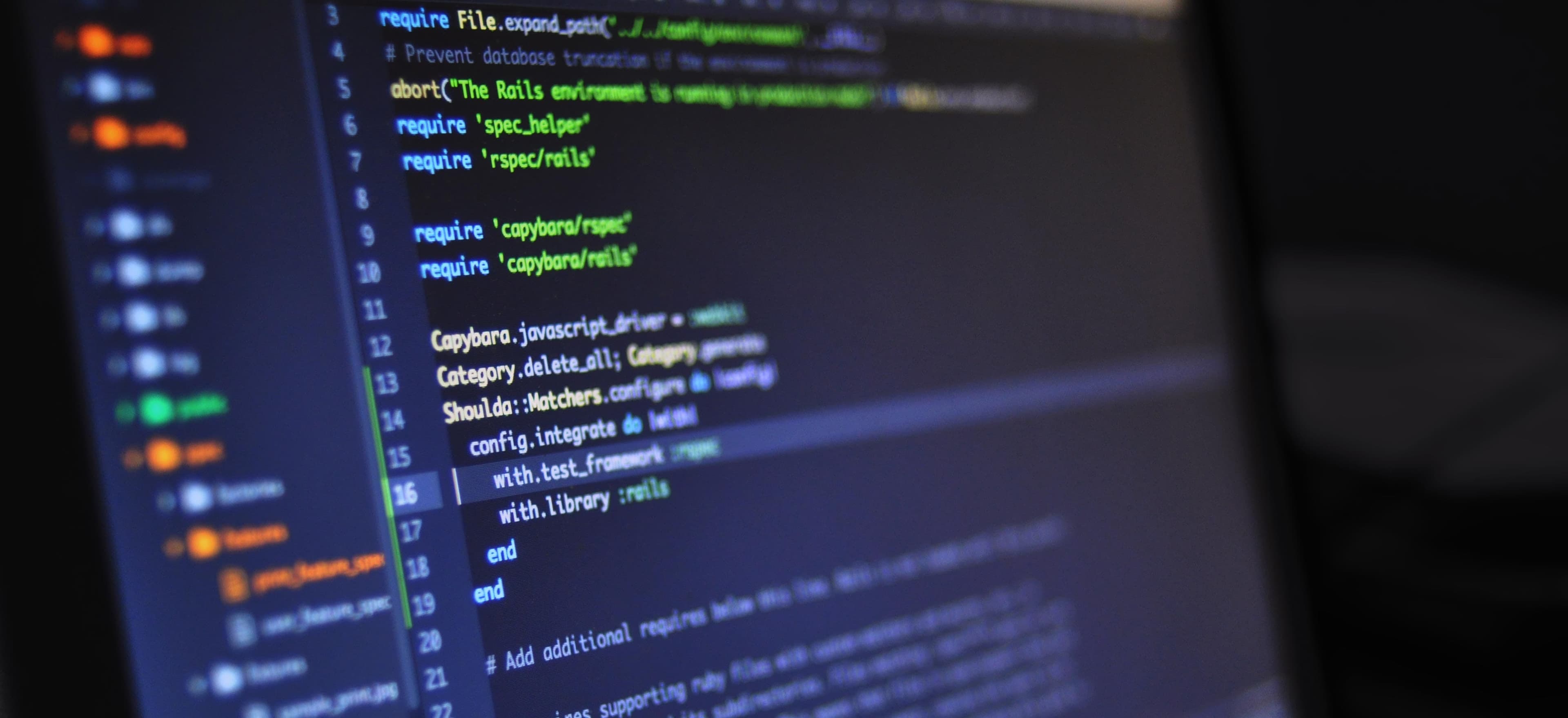
- Published on
Mastering Many-to-Many Mapping in Hibernate: A Guide
When working with relational databases, it's common to encounter scenarios where entities have a many-to-many relationship. In such cases, Hibernate, a popular ORM framework for Java, offers powerful features for handling these relationships. Understanding and mastering many-to-many mapping in Hibernate is essential for building efficient and maintainable applications.
In this guide, we will delve into the intricacies of many-to-many mapping in Hibernate, exploring the concepts, best practices, and implementation techniques.
Understanding Many-to-Many Mapping
In a relational database, a many-to-many relationship exists between two entities when each instance of one entity can be associated with multiple instances of the other entity, and vice versa. For example, consider a scenario where Student
entities can enroll in multiple Course
entities, and each Course
can have multiple enrolled Student
entities.
In a traditional relational database design, many-to-many relationships are implemented using a junction table, also known as an associative or link table. This junction table contains foreign key references to the primary keys of the two associated entities, effectively establishing the relationship between them.
Mapping Many-to-Many Relationships in Hibernate
The Entity Relationship
In Hibernate, the mapping of many-to-many relationships involves defining the association between the entities and specifying how this association is represented in the underlying database tables.
Let's consider the example of Student
and Course
entities. To establish a many-to-many relationship between these entities, we need to define the association in each entity class using appropriate annotations or XML mapping.
Annotation-Based Mapping
Student Entity
@Entity
@Table(name = "students")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "student_id")
private Long studentId;
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "student_course",
joinColumns = @JoinColumn(name = "student_id"),
inverseJoinColumns = @JoinColumn(name = "course_id"))
private Set<Course> courses = new HashSet<>();
// Other properties and methods
}
Course Entity
@Entity
@Table(name = "courses")
public class Course {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name = "course_id")
private Long courseId;
@ManyToMany(mappedBy = "courses")
private Set<Student> students = new HashSet<>();
// Other properties and methods
}
In the Student
entity, the @ManyToMany
annotation is used to establish the many-to-many relationship with the Course
entity. The @JoinTable
annotation specifies the name of the junction table and the columns used for joining.
In the Course
entity, the @ManyToMany
annotation is used with the mappedBy
attribute to indicate the inverse side of the relationship. This tells Hibernate to look for the mapping information in the Student
entity.
Benefits of Annotation-Based Mapping
- Simplified Configuration: Annotations provide a more concise and readable way to define the entity relationships, reducing the need for boilerplate XML configuration.
- Bi-Directional Navigation: With bidirectional mapping, you can navigate from
Student
toCourse
and vice versa, simplifying traversal of the many-to-many relationship.
Handling Many-to-Many Relationships in Code
Student student = new Student();
Course course = new Course();
student.getCourses().add(course);
course.getStudents().add(student);
// Persist the entities
session.save(student);
session.save(course);
In the code snippet above, we create a many-to-many association between a Student
and a Course
. We add the Course
to the set of courses associated with the Student
, and add the Student
to the set of students associated with the Course
.
Best Practices and Considerations
Efficient Fetching Strategies
When working with many-to-many associations, it's important to consider the fetching strategy to optimize database interactions. Hibernate offers various fetching strategies such as EAGER
and LAZY
. By default, many-to-many associations are fetched lazily, meaning that associated entities are loaded only when accessed for the first time.
Cascading Operations
Cascading operations, specified using the cascade
attribute in the mapping annotations, define how operations such as persist
, merge
, remove
, etc., are cascaded from one entity to the associated entities. Care should be taken when defining cascading behavior to avoid unintended side effects.
Junction Table Considerations
In some cases, the junction table may contain additional columns beyond the foreign keys. These additional columns represent attributes specific to the relationship itself. In such scenarios, Hibernate provides support for mapping these additional columns using an entity to represent the association.
Indexing and Constraints
Applying indexes to the foreign key columns in the junction table can improve query performance for many-to-many associations. Additionally, defining appropriate constraints ensures data integrity and consistency.
Lessons Learned
Mastering many-to-many mapping in Hibernate is crucial for building robust and performant applications with complex relationships between entities. By understanding the underlying concepts, leveraging the right mapping techniques, and adhering to best practices, developers can effectively model and manage many-to-many associations in their Hibernate-based applications.
In this guide, we've explored the fundamentals of many-to-many mapping in Hibernate, covering annotation-based mapping, best practices, and considerations for efficient handling of many-to-many relationships. Armed with this knowledge, developers can confidently navigate and master the intricacies of many-to-many mapping in Hibernate.
For further reading and exploration, refer to the official Hibernate documentation for comprehensive insights into entity associations and mapping strategies.
Mastering many-to-many mapping in Hibernate opens the doors to building scalable and maintainable applications that effectively model complex relationships between entities.
Happy mapping!