Boosting Speed: Mastering Simple Binary Encoding
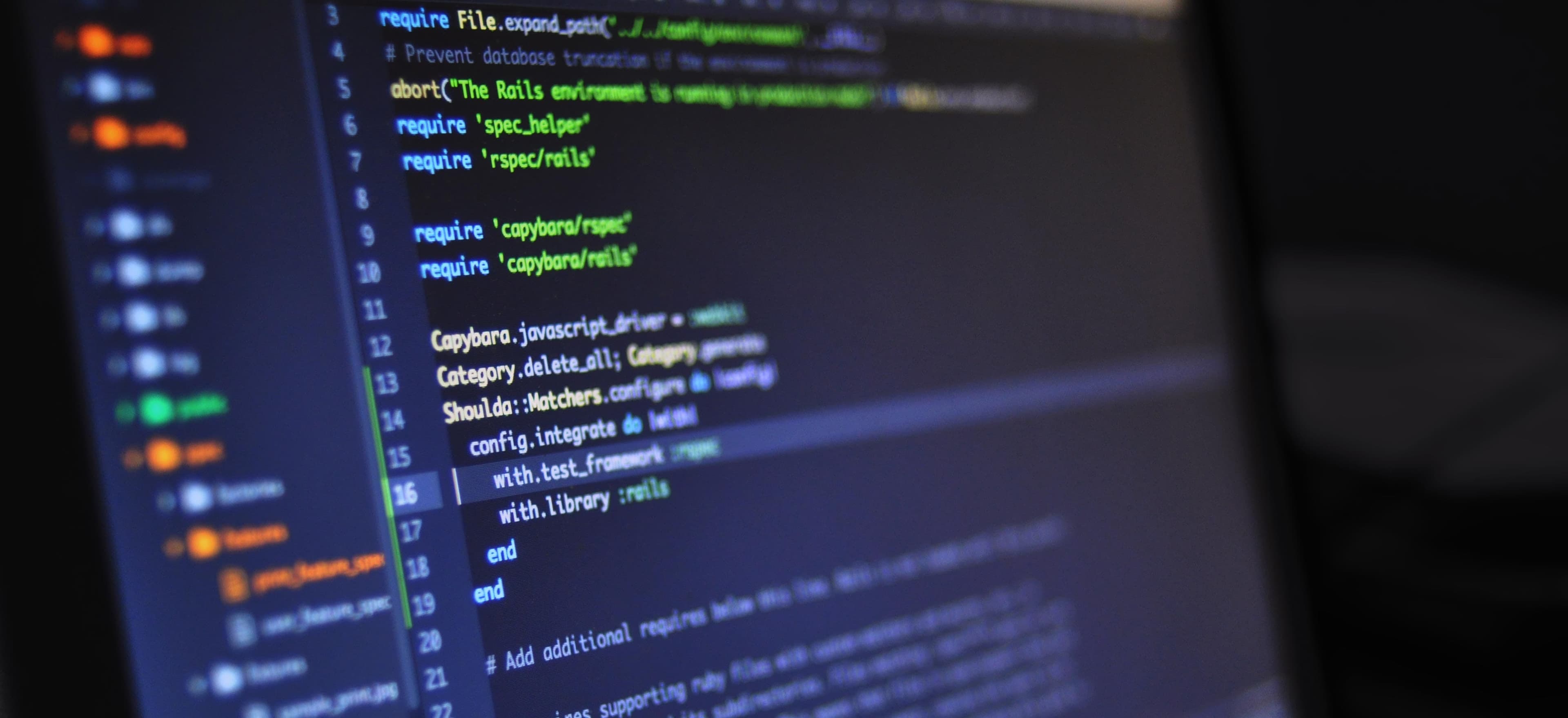
- Published on
Boosting Speed: Mastering Simple Binary Encoding
As a Java developer, you’re always looking for ways to optimize the performance of your code. One area where significant performance gains can be made is in the encoding and decoding of data. Simple Binary Encoding (SBE) is a high-performance encoding method that can be used to serialize and deserialize data in a way that is both efficient and easy to work with. In this blog post, we’ll explore how SBE works and how you can use it to boost the speed of your Java applications.
Understanding Simple Binary Encoding
At its core, Simple Binary Encoding is a method for encoding and decoding structured data in a way that is highly efficient in terms of both speed and space. It works by defining a schema that describes the structure of the data to be encoded, and then using that schema to generate Java classes that can efficiently serialize and deserialize instances of that data.
One of the key features of SBE is its use of fixed-width fields. By using fixed-width fields, SBE eliminates the need for delimiters or markers to separate different pieces of data, which can significantly reduce the overhead involved in both encoding and decoding.
Using SBE in Java
In order to use SBE in Java, you’ll need to start by defining a schema that describes the structure of the data you want to encode. This schema is written in a simple, human-readable format that defines the fields and their types, as well as any encoding rules that should be applied.
Once you have your schema defined, you can use the SBE tooling to generate Java classes that correspond to the schema. These generated classes provide an efficient way to work with instances of the encoded data, handling the serialization and deserialization details for you.
Let’s take a look at a simple example to illustrate how this works. Suppose we have a schema that describes a basic message format, including a message header, a message type, and a message body. Here’s how we could define that schema:
/*[
package com.example.sbe;
message MessageHeader
{
int16_t blockLength; // Length of the message body in bytes
int16_t templateId; // Template identifier for the message
int32_t schemaId; // Schema identifier for the message definition
int16_t version; // Version number of the schema
}
message MessageType
{
uint8_t type; // Type of the message
}
message MessageBody
{
int64_t timestamp; // Timestamp of the message
int32_t entityId; // ID of the entity
}
]*/
In this schema, we have defined three message types: MessageHeader
, MessageType
, and MessageBody
, each with its own set of fields and types. Once we have our schema defined, we can use the SBE tooling to generate Java classes that correspond to these message types.
Generating Java Classes
Using the SBE tooling, we can generate Java classes from our schema by running a simple command. This will produce Java classes that provide a convenient way to work with instances of the encoded data, abstracting away the low-level details of serialization and deserialization.
Here’s an example of the Java classes that the SBE tooling might generate based on our schema:
package com.example.sbe;
public class MessageHeader
{
// Fields and methods for working with the message header
}
public class MessageType
{
// Fields and methods for working with the message type
}
public class MessageBody
{
// Fields and methods for working with the message body
}
With these generated classes, we can easily create, encode, and decode instances of our message types without having to worry about the details of the underlying binary encoding.
The Performance Advantage
So, why go through the trouble of using SBE in Java? The answer lies in the performance advantages that SBE offers. By using fixed-width fields and eliminating the need for delimiters or markers, SBE can provide significant speed improvements over other encoding methods.
In addition to the speed advantages, SBE also offers a compact binary representation of the data, which can result in significant space savings, especially when working with large volumes of data.
Final Considerations
In this blog post, we’ve explored the basics of Simple Binary Encoding and how it can be used to boost the speed of Java applications. By defining a schema that describes the structure of the data, generating Java classes from that schema, and leveraging the performance advantages of fixed-width fields, SBE provides a powerful tool for optimizing the encoding and decoding of structured data.
If you’re interested in diving deeper into SBE, be sure to check out the official SBE documentation, where you can find more information and resources to help you master this powerful encoding method.
In conclusion, mastering Simple Binary Encoding can unlock new levels of performance in your Java applications, making it a valuable addition to any developer’s toolkit. So why not give it a try and see the speed improvements for yourself? Happy coding!