Boost Your API: Mastering Bulk Operations in REST
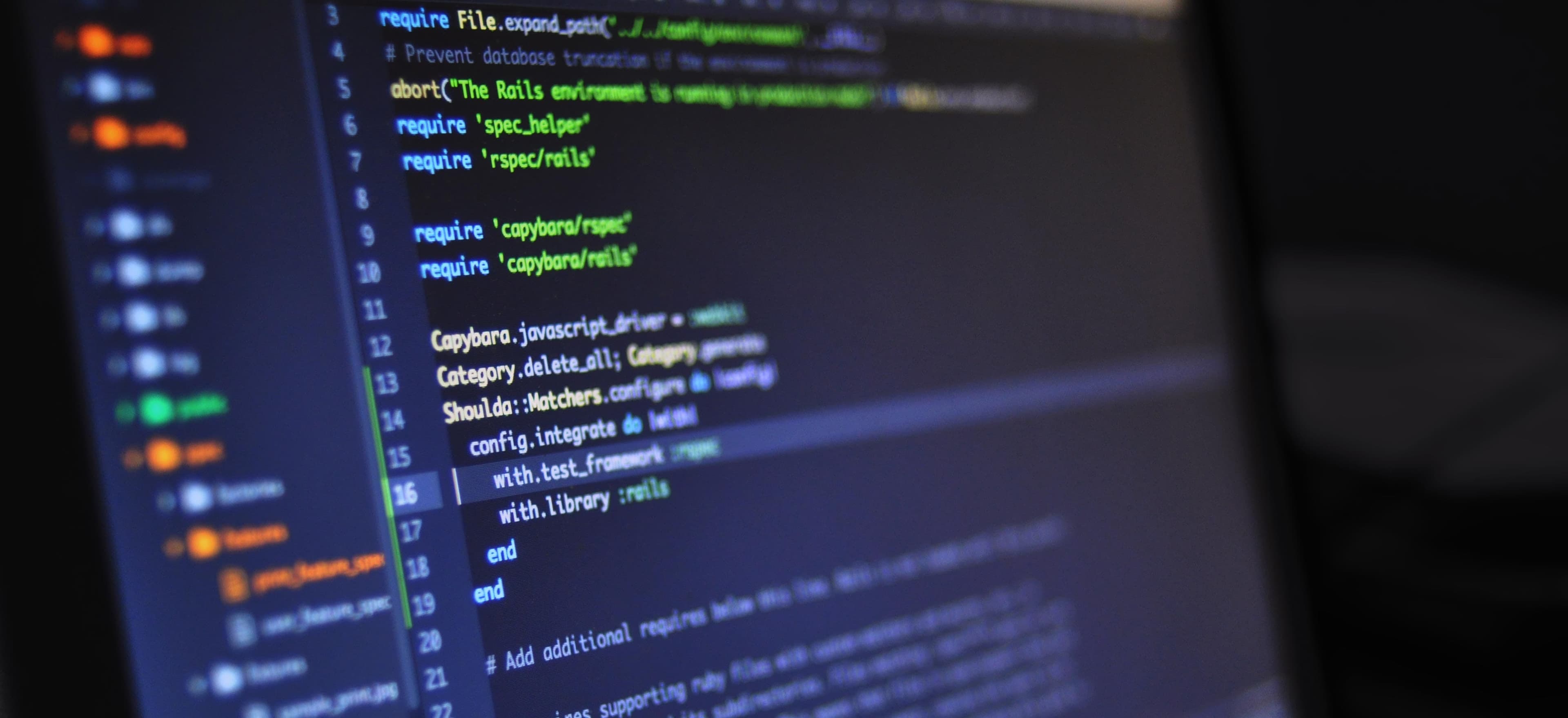
- Published on
Boost Your API: Mastering Bulk Operations in REST
As a Java developer, you constantly look for ways to optimize and improve the efficiency of your REST API. One of the key strategies for achieving this is by mastering bulk operations. In this article, we will delve into the concepts of bulk operations in REST APIs and explore how to implement them effectively in Java.
Understanding Bulk Operations
Bulk operations allow the client to perform multiple operations in a single request, reducing the overhead of making separate requests for each operation. This can lead to significant improvements in performance, especially when dealing with large datasets.
Benefits of Bulk Operations
- Reduced Overhead: By bundling multiple operations into a single request, you can minimize the overhead of initiating and managing individual requests.
- Improved Performance: Bulk operations can lead to faster processing of data, particularly when dealing with complex or large-scale operations.
- Enhanced Efficiency: Implementing bulk operations can streamline the overall workflow, making the API more efficient and responsive.
Implementing Bulk Operations in Java
Now, let's explore how we can implement bulk operations in a Java-based REST API. We'll take a step-by-step approach to understand the key components involved in facilitating bulk operations.
1. Designing the API
First and foremost, a well-designed API is crucial for supporting bulk operations. When defining endpoints for bulk operations, it's important to follow RESTful principles and adhere to standard HTTP methods such as POST
for creating new resources or performing operations on multiple resources.
@PostMapping("/bulk/operations")
public ResponseEntity<?> performBulkOperations(@RequestBody List<OperationRequest> operationRequests) {
// Implement bulk operations logic
}
In the above example, we use the POST
method to handle a bulk operations request and expect a list of operation requests in the request body.
2. Processing the Bulk Operations
Once the bulk operations request is received, the next step is to process the operations efficiently. This may involve iterating through the list of operation requests and performing the necessary actions for each request.
public ResponseEntity<?> performBulkOperations(@RequestBody List<OperationRequest> operationRequests) {
List<OperationResponse> operationResponses = new ArrayList<>();
for (OperationRequest request : operationRequests) {
// Process each operation request and build the responses
}
// Return the responses
return ResponseEntity.ok(operationResponses);
}
In the above code snippet, we iterate through the list of operation requests, process each request, and build a corresponding list of operation responses.
3. Handling Error Scenarios
It's essential to handle error scenarios gracefully when dealing with bulk operations. If any individual operation within the bulk request fails, the API should provide clear and meaningful error responses while continuing to process the remaining operations.
public ResponseEntity<?> performBulkOperations(@RequestBody List<OperationRequest> operationRequests) {
List<OperationResponse> operationResponses = new ArrayList<>();
List<OperationErrorResponse> errorResponses = new ArrayList<>();
for (OperationRequest request : operationRequests) {
try {
// Process each operation request and build the responses
} catch (Exception e) {
// Capture and log the error, then build the error responses
}
}
if (!errorResponses.isEmpty()) {
// Return error responses along with successful responses
return ResponseEntity.status(HttpStatus.MULTI_STATUS).body(errorResponses);
} else {
// Return only successful responses
return ResponseEntity.ok(operationResponses);
}
}
The above code demonstrates error handling within the bulk operations logic, where error responses are collected and returned separately if any operation encounters an error.
4. Optimizing Performance
To optimize the performance of bulk operations, consider leveraging asynchronous processing and parallel execution where applicable. Java provides various concurrency utilities, such as ExecutorService
and CompletableFuture
, to execute tasks concurrently and improve the overall throughput of bulk operations.
public ResponseEntity<?> performBulkOperations(@RequestBody List<OperationRequest> operationRequests) {
List<OperationResponse> operationResponses = new ArrayList<>();
List<CompletableFuture<Void>> futures = new ArrayList<>();
for (OperationRequest request : operationRequests) {
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Process each operation request and build the responses
});
futures.add(future);
}
CompletableFuture.allOf(futures.toArray(new CompletableFuture[0])).join();
// Return the responses
return ResponseEntity.ok(operationResponses);
}
In the above code, we utilize CompletableFuture
to process each operation request asynchronously, improving the execution time of bulk operations.
In Conclusion, Here is What Matters
Mastering bulk operations in REST APIs is an indispensable skill for enhancing the performance and efficiency of your Java-based API implementations. By optimizing the design, processing, error handling, and performance of bulk operations, you can significantly elevate the overall quality of your API.
In this article, we've explored the fundamental concepts of bulk operations, delved into the implementation of bulk operations in Java, and highlighted key strategies for effectively managing bulk operations within your REST API. By applying these principles, you can take your API to the next level by maximizing its scalability, responsiveness, and overall user experience.
Now it's your turn to incorporate these best practices and unleash the full potential of bulk operations in your Java-based REST API!
The article provides a comprehensive guide to mastering bulk operations in REST APIs using Java. It covers the fundamental concepts, implementation strategies, and best practices for optimal performance. By following this guide, Java developers can elevate the efficiency and scalability of their REST APIs.