Why Mixing State with Code Spells Disaster for Your App
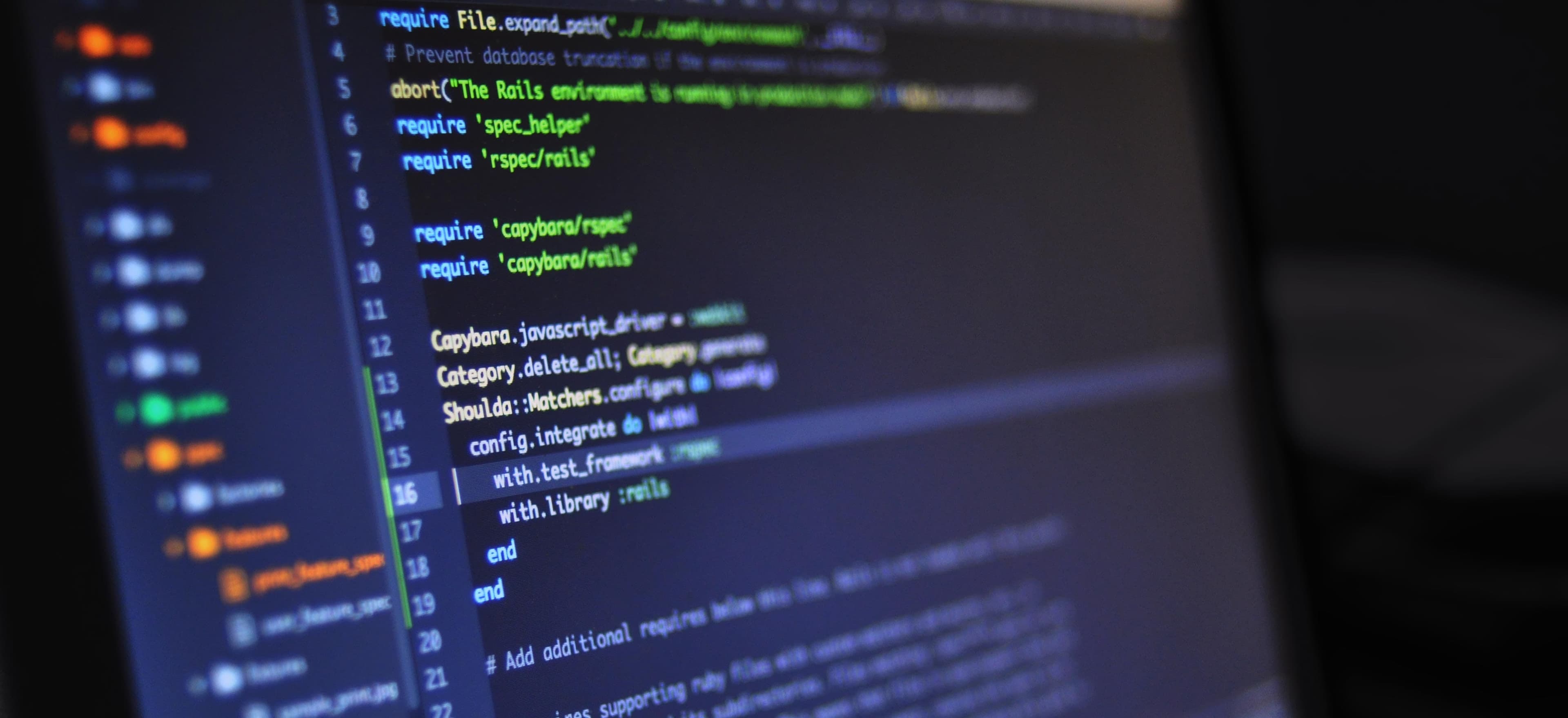
- Published on
Why Mixing State with Code Spells Disaster for Your App
Software development can be a complex task, especially when it comes to managing the state of an application. State management is a crucial aspect of building a robust and maintainable application. However, mixing state with code can lead to disastrous consequences for your app.
In this blog post, we'll explore the pitfalls of mixing state with code and why it's important to separate the two. We'll also discuss the best practices for managing state in your Java applications and how you can avoid the common pitfalls.
The Pitfalls of Mixing State with Code
When state and code are intertwined, it becomes challenging to reason about the behavior of your application. Here are some of the most common pitfalls of mixing state with code:
-
Increased Complexity: Mixing state with code can lead to increased complexity within your application. As the application grows, it becomes harder to manage and reason about the state, leading to maintenance challenges.
-
Code Duplication: When stateful logic is scattered throughout your codebase, it often leads to code duplication. Duplication makes your code harder to maintain and increases the likelihood of introducing bugs.
-
Scalability Issues: As your application scales, managing state becomes even more challenging. It's essential to have a clear separation between state and code to ensure scalability and maintainability.
-
Testing Challenges: Testing becomes more complicated when state and code are tightly coupled. It's difficult to write effective tests that cover all edge cases when the state is mixed with the code.
Separation of Concerns: Managing State and Code
The concept of separation of concerns is crucial in software development. It suggests that different aspects of a software system should be separated into distinct sections, each addressing a separate concern. When it comes to state and code, it's important to maintain a clear separation between the two.
Managing State
In Java applications, managing state can be achieved through various techniques such as using immutable objects, leveraging design patterns like the Observer pattern, or employing state management libraries like Redux or MobX.
By managing the state separately from the application logic, you can ensure that your code remains clean, maintainable, and easy to reason about. Additionally, separating state management allows for better testability and scalability of your application.
Separating Business Logic
The business logic of your application should be distinct from the state management. Business logic pertains to the rules and behaviors that define how your application operates. By isolating the business logic from the state, you can ensure that your code remains focused and easier to maintain.
Best Practices for State Management in Java
Now that we've explored the importance of separating state from code, let's delve into some best practices for state management in Java applications.
Immutability
One of the fundamental principles of effective state management is immutability. In Java, immutability can be achieved by creating immutable objects using the final
keyword for fields and ensuring that state cannot be modified once it's instantiated.
public class ImmutableState {
private final String name;
public ImmutableState(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
By enforcing immutability, you can prevent unexpected changes to the application state, leading to more predictable behavior and easier debugging.
Encapsulation
Encapsulation is another key aspect of effective state management. It involves restricting direct access to the internal state of an object and providing controlled access through methods.
public class EncapsulatedState {
private int count;
public int getCount() {
return count;
}
public void increment() {
count++;
}
}
By encapsulating the state and providing controlled access, you can ensure that the state remains consistent and prevent unintended modifications.
Observer Design Pattern
The Observer pattern is a useful design pattern for implementing event handling and communication between components in an application. It enables objects to subscribe and unsubscribe from changes in state, promoting loose coupling and flexibility.
public interface Observer {
void update();
}
public class Subject {
private List<Observer> observers = new ArrayList<>();
public void subscribe(Observer observer) {
observers.add(observer);
}
public void unsubscribe(Observer observer) {
observers.remove(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update();
}
}
}
By leveraging the Observer pattern, you can decouple state changes from the dependent components, making your code more modular and easier to maintain.
Avoiding the Common Pitfalls
To avoid the common pitfalls of mixing state with code, follow these best practices:
-
Use Immutable Objects: Embrace immutability to prevent unexpected state changes and promote predictability in your application.
-
Encapsulate State: Restrict direct access to the internal state of objects and provide controlled access through methods to maintain consistency.
-
Leverage Design Patterns: Utilize design patterns like the Observer pattern to decouple state changes from the dependent components, ensuring a more maintainable and scalable codebase.
Key Takeaways
In conclusion, mixing state with code can lead to disastrous consequences for your application, including increased complexity, code duplication, scalability issues, and testing challenges. To mitigate these risks, it's essential to embrace the separation of concerns and follow best practices for managing state in your Java applications. By maintaining a clear separation between state and code, you can ensure a clean, maintainable, and scalable codebase for your application.
Remember, the key to a robust and maintainable application lies in the thoughtful separation of state and code.
By adhering to the best practices for state management in Java, you can ensure a clean, maintainable, and scalable codebase for your application. If you're interested in adopting state management libraries like Redux or MobX, consider referring to the Redux documentation or the MobX official website for further insights.