Avoiding Test Failures: Mastering Byteman & Junit
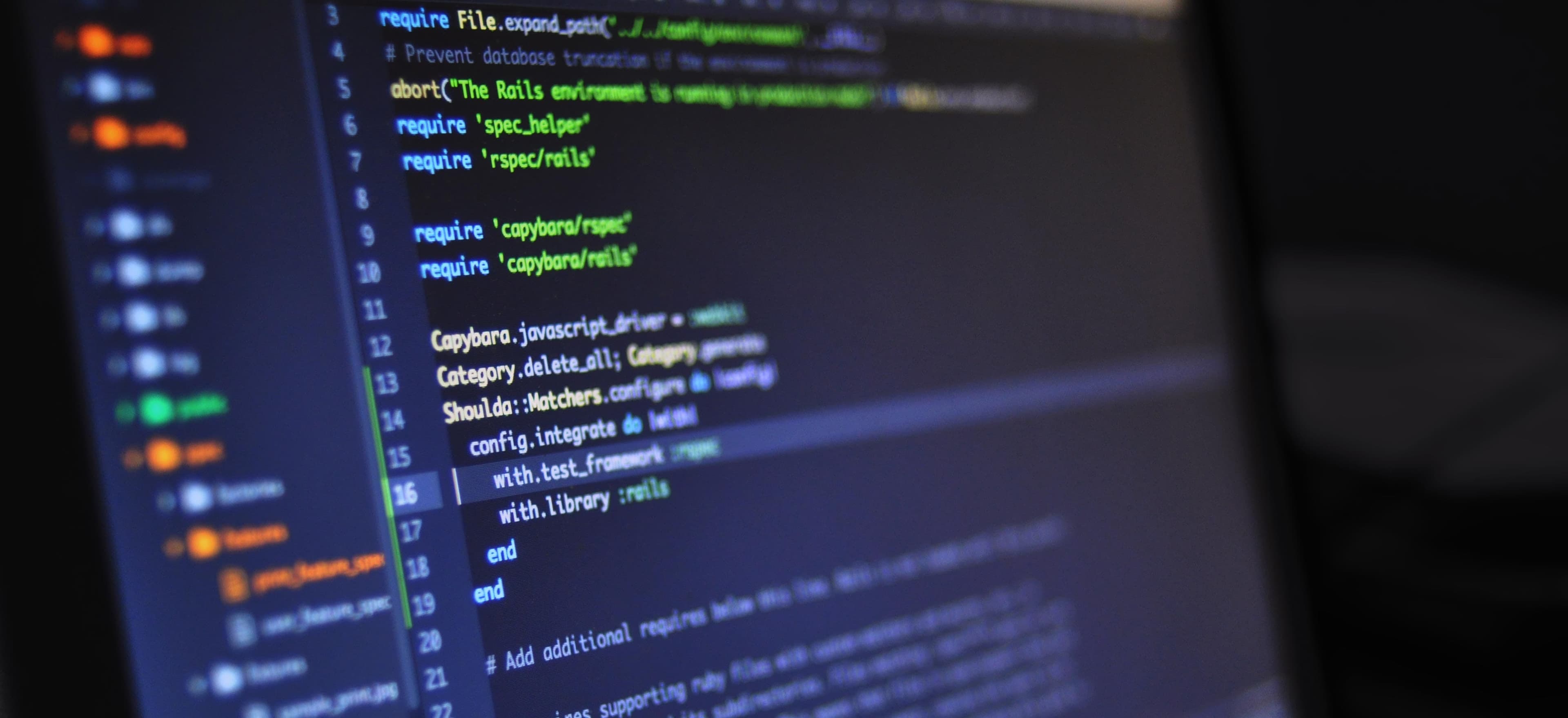
- Published on
Avoiding Test Failures: Mastering Byteman & Junit
When it comes to writing robust and reliable Java applications, testing is a crucial aspect of the development process. Unit testing, in particular, plays a significant role in ensuring that each component of the application works as expected. However, writing effective unit tests can be challenging, and even with a comprehensive test suite, identifying and debugging failures can be time-consuming. This is where tools like Byteman and JUnit come into play, offering developers powerful capabilities to not only write comprehensive tests but also to troubleshoot and address test failures effectively.
In this blog post, we will explore how to harness the power of Byteman and JUnit to prevent, identify, and troubleshoot test failures in Java applications. We will dive into practical examples and best practices to demonstrate how these tools can significantly improve the testing process and enhance the overall reliability of your Java codebase.
Understanding Byteman
Byteman is a versatile and powerful Java agent that allows developers to inject Java code into existing applications at runtime. This capability is particularly useful for testing, as it enables dynamic instrumentation of classes and methods, effectively providing a way to alter their behavior temporarily for testing purposes. Byteman operates by injecting bytecode into the target application, making it possible to modify method behavior, inject exceptions, and gather diagnostic information during runtime.
Installing Byteman
To get started with Byteman, you can download the latest release from the official website. Once downloaded, you can extract the Byteman distribution to a suitable location on your system.
Getting Started with Byteman Rules
Byteman rules define the actions to be performed when specific conditions are met at runtime. These rules are written in a simple scripting language and can be used to inject code into the target application's execution flow. Let's take a look at a basic Byteman rule to gain an understanding of its structure and usage:
RULE example_rule
CLASS MyClass
METHOD myMethod
AT ENTRY
IF TRUE
DO traceln("Entering myMethod")
ENDRULE
In this example, we define a rule named example_rule
that targets the myMethod
within the MyClass
class. The AT ENTRY
clause specifies that the action should be triggered at the entry point of the method. The IF TRUE
condition ensures that the action is always executed. Finally, the DO
block contains the action to be performed, which, in this case, is to print a trace message indicating the entry into the method.
By defining and applying such rules, developers can gain valuable insights into the runtime behavior of their applications, making it easier to identify and address potential issues.
Leveraging Byteman for Test Resilience
One of the common challenges in writing unit tests is dealing with external dependencies, such as network services or databases. Byteman provides a compelling solution to this problem by enabling developers to inject fault scenarios and test failure conditions without modifying the application code itself. Let's consider an example where a unit test needs to simulate a network timeout scenario to ensure that the application handles such failures gracefully.
RULE simulate_network_timeout
CLASS MyNetworkService
METHOD sendData
AT ENTRY
IF TRUE
DO throw new IOException("Simulating network timeout")
ENDRULE
In this rule, we inject code into the sendData
method of the MyNetworkService
class to throw an IOException
, simulating a network timeout scenario. By applying such rules, developers can comprehensively test the resilience of their code against various failure scenarios without having to rely on the actual occurrence of such events during testing.
Enhancing Unit Tests with JUnit
JUnit is a widely used framework for writing and executing unit tests in Java. With its rich set of assertion methods and flexible test execution capabilities, JUnit empowers developers to create comprehensive test suites that validate the behavior of their codebase. While JUnit excels at writing and running tests, integrating Byteman into the testing process can significantly enhance the depth and effectiveness of unit testing.
Setting up JUnit with Byteman
To integrate Byteman with JUnit tests, we can leverage Byteman's support for running with the -javaagent
option. This allows us to specify the Byteman agent to be loaded when running our test cases. Additionally, we can utilize Byteman's rule file to define the specific rules to be applied during test execution.
@Rule
public BytemanRule byteman = BytemanRule.create();
In this JUnit test class, we use the @Rule
annotation to create a Byteman rule instance, allowing us to define and apply Byteman rules within our test methods. By incorporating Byteman into our test setup, we can dynamically inject fault scenarios and capture diagnostic information during the execution of our unit tests, offering a powerful mechanism to validate the resilience and robustness of our code.
Addressing Test Failures with Byteman
In addition to enhancing the scope and resilience of unit tests, Byteman provides valuable capabilities for diagnosing and troubleshooting test failures. By injecting tracing statements and logging information at critical points in the application's execution flow, developers can gain visibility into the internal behavior of the code, making it easier to identify the root causes of test failures.
RULE trace_method_execution
INTERFACE MyClass
METHOD .* # This matches any method in the MyClass interface
AT ENTRY
IF TRUE
DO traceln("Executing method: " + $0.getClass().getName() + "." + $0.getName())
ENDRULE
In this example, we define a rule that traces the execution of all methods within the MyClass
interface, providing valuable insights into the sequence and context of method invocations during test execution. By leveraging such tracing capabilities, developers can effectively pinpoint the source of test failures and take targeted actions to address them.
Best Practices for Test-Driven Development with Byteman & JUnit
As we delve into harnessing the combined power of Byteman and JUnit for effective test-driven development, it's essential to adhere to best practices to maximize the benefits of these tools:
-
Targeted Injection: When defining Byteman rules, focus on injecting code at specific points in the application's execution flow relevant to the test scenarios being validated.
-
Isolation and Cleanup: Ensure that Byteman rules are applied in a controlled and isolated manner to prevent interference with other test cases. Additionally, clean up any injected changes to the application state after test execution to maintain consistency.
-
Diagnostic Tracing: Leverage Byteman's tracing capabilities to gain comprehensive insight into the internal behavior of the application during test execution, facilitating efficient debugging and troubleshooting.
-
Collaborative Integration: Foster seamless collaboration between Byteman and JUnit by integrating fault injection and diagnostic tracing seamlessly into the test setup, enabling a unified and enhanced testing process.
By embracing these best practices, developers can leverage Byteman and JUnit to craft resilient tests that robustly validate the functionality, error handling, and performance characteristics of their Java applications.
In Conclusion, Here is What Matters
In the realm of Java development, test failures can be a significant source of frustration and impediment to progress. However, with the strategic adoption of tools like Byteman and JUnit, developers can effectively preempt, diagnose, and address test failures, empowering them to create more resilient and reliable code. By embracing the dynamic bytecode manipulation capabilities of Byteman and the comprehensive testing framework offered by JUnit, developers can elevate their testing practices and fortify their code against potential failure scenarios.
Through the examples and best practices outlined in this post, we have shed light on how Byteman and JUnit can work in tandem to bolster the testing process, paving the way for enhanced test-driven development and the cultivation of more robust Java applications. As the landscape of Java testing continues to evolve, the synergy between Byteman and JUnit presents a compelling opportunity for developers to master the art of test resilience and mitigate the impact of test failures with precision and finesse.