Tackling HTTP Endpoint Polling Challenges with Spring
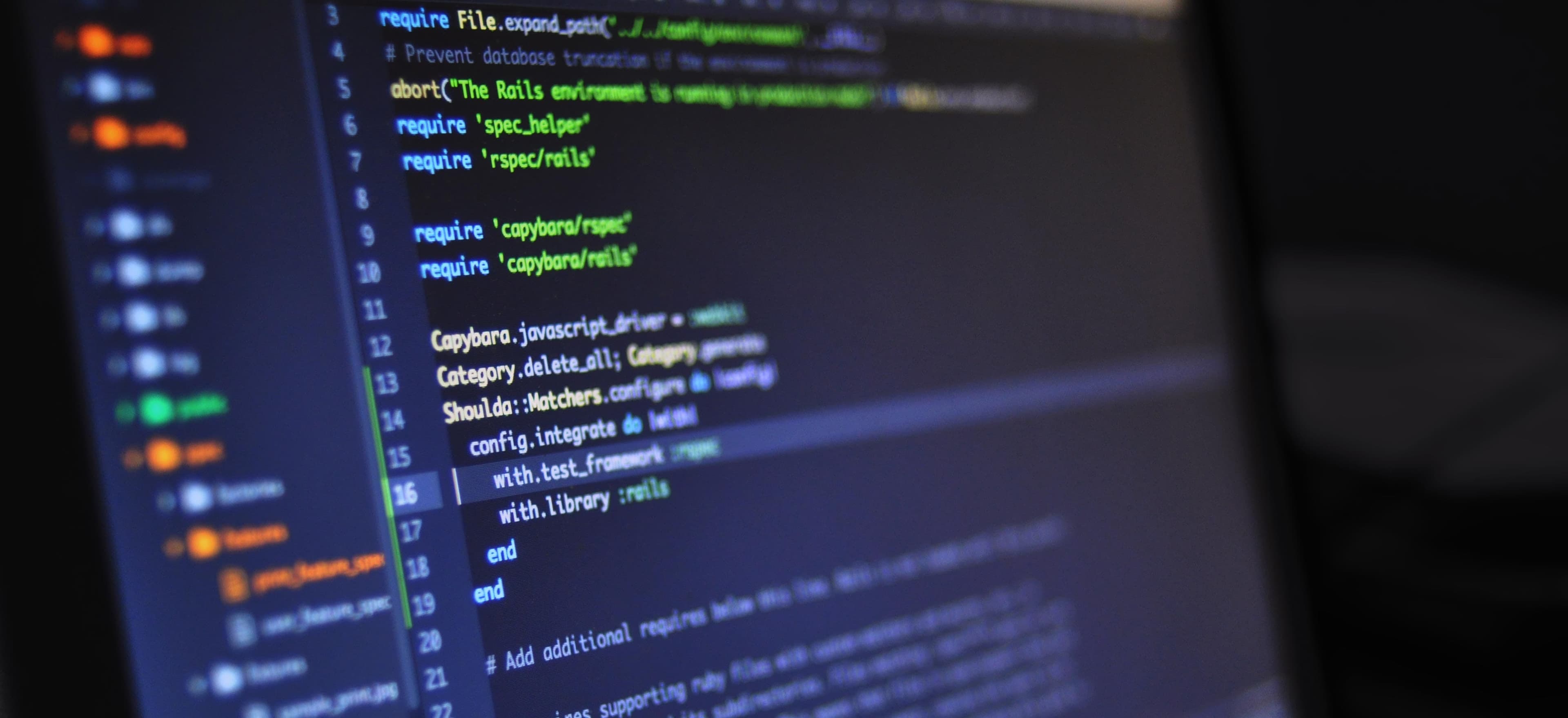
- Published on
Tackling HTTP Endpoint Polling Challenges with Spring
When working with asynchronous communication between microservices or systems, it's common to encounter the need to poll HTTP endpoints for data updates. In a real-time application, the client may need to fetch the latest information from a server at regular intervals, but the traditional polling approach can be inefficient and resource-consuming. In this blog post, we'll explore how to address these challenges using Spring, a popular Java framework for building robust, scalable applications.
The Pitfalls of Traditional Polling
Resource Consumption
Traditional polling involves repeatedly sending HTTP requests at fixed intervals, regardless of whether there are any updates available. This approach can lead to unnecessary consumption of network bandwidth and server resources.
Latency
In a high-latency environment, frequent polling can introduce significant delays in obtaining the latest data, impacting the responsiveness of the application.
Inefficient Data Transfer
Fetching the entire payload with every poll, even if only a small portion has changed, results in inefficient data transfer and increases the processing overhead on both the client and the server.
Introducing Reactive Polling with Spring WebFlux
To address the limitations of traditional polling, we can leverage the reactive capabilities of Spring WebFlux, which provides a non-blocking, event-driven architecture. By embracing reactive programming, we can build more efficient and scalable polling mechanisms.
Project Reactor
Spring WebFlux is built on top of Project Reactor, which offers a reactive library for composing asynchronous and event-driven programs. Project Reactor provides the Flux
and Mono
types, representing sequences of 0 or 1-n items, respectively, and supports backpressure to handle the flow of data efficiently.
WebClient
Spring WebFlux includes a WebClient
that offers a non-blocking and reactive way to consume RESTful web services. It allows us to make HTTP requests and handle the responses asynchronously, making it ideal for implementing reactive polling of HTTP endpoints.
Let's take a look at how we can use WebClient
in combination with Project Reactor to implement efficient HTTP endpoint polling.
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Flux;
import java.time.Duration;
public class HttpPollingService {
private final WebClient webClient;
public HttpPollingService(WebClient.Builder webClientBuilder) {
this.webClient = webClientBuilder.build();
}
public Flux<DataUpdate> pollEndpoint(String url, Duration interval) {
return Flux.interval(interval)
.flatMap(ignore -> webClient.get().uri(url).retrieve().bodyToMono(DataUpdate.class))
.distinctUntilChanged();
}
}
In the code snippet above, we create a HttpPollingService
that utilizes WebClient
to poll an HTTP endpoint at specified intervals. The Flux.interval
operator generates a sequence of increasing long values, which we then use to trigger the HTTP requests at regular intervals. We then use the flatMap
operator to perform the HTTP GET request and map the response to a DataUpdate
object using bodyToMono
. Finally, we use distinctUntilChanged
to ensure that we only emit distinct data updates to downstream subscribers.
Benefits of Reactive Polling
Efficient Resource Utilization
Reactive polling allows us to adaptively fetch data only when there are actual updates, leading to more efficient use of network bandwidth and server resources.
Reduced Latency
By fetching and transmitting only the changed data, reactive polling minimizes latency and improves the overall responsiveness of the application.
Backpressure Handling
Reactive programming with Project Reactor enables built-in backpressure handling, preventing overwhelming the downstream consumers with data, thus ensuring a more balanced flow and efficient resource usage.
Closing Remarks
In this blog post, we've explored the challenges of traditional HTTP endpoint polling and introduced a more efficient and responsive approach using Spring WebFlux's reactive capabilities. By leveraging Project Reactor and the WebClient
, we can implement a reactive polling mechanism that efficiently fetches and processes data updates from HTTP endpoints. This approach not only optimizes resource usage but also improves the overall responsiveness of real-time applications.
Reactive polling is just one of the many ways Spring empowers developers to build high-performance, scalable, and responsive applications. By embracing reactive programming and leveraging the capabilities of Spring WebFlux, developers can tackle the challenges of modern distributed systems with confidence.
If you're interested in diving deeper into reactive programming with Spring, take a look at the official Spring WebFlux documentation for comprehensive guides and tutorials.
Incorporate reactive polling into your applications and witness a significant boost in efficiency and responsiveness!
Checkout our other articles