Master Java Exchanger: Avoid Common Synchronization Pitfalls
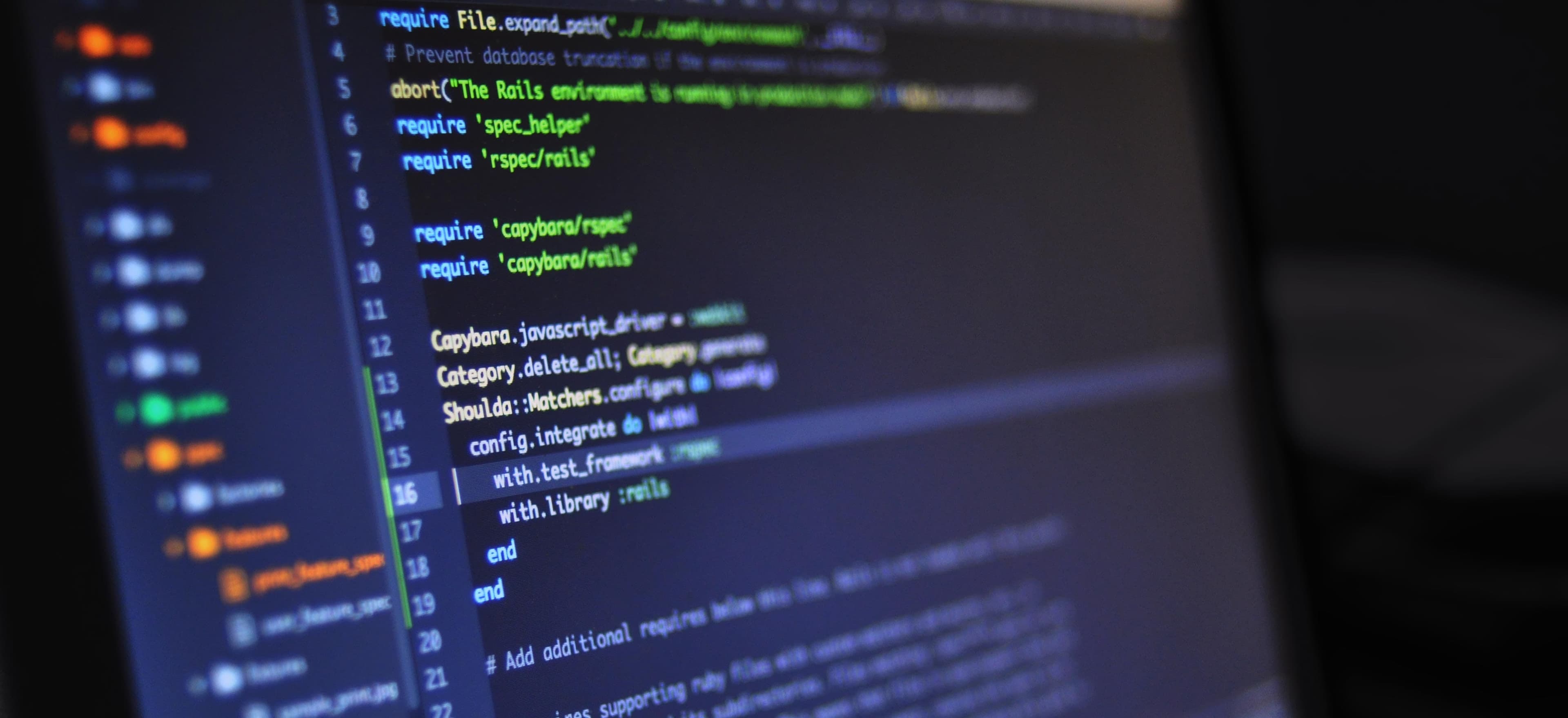
- Published on
Master Java Exchanger: Avoid Common Synchronization Pitfalls
Java's Exchanger
is a powerful synchronization aid that allows two threads to exchange objects at a rendezvous point. It provides a high-level mechanism for coordinating the exchange of data between threads. However, using Exchanger
efficiently and correctly requires a deep understanding of its intricacies. In this post, we'll explore the basics of Exchanger
and delve into common synchronization pitfalls to avoid when using this feature.
What is a Java Exchanger?
A java.util.concurrent.Exchanger
is a synchronization point at which threads can pair and swap elements within pairs. Each thread presents some object on entry to the exchange
method, matches with its partner, and receives its partner's object in return. This synchronization point is typically used when two threads need to coordinate and exchange data.
How to Use Exchanger in Java
Using an Exchanger
involves several steps:
-
Create an instance of
Exchanger
.Exchanger<String> exchanger = new Exchanger<>();
-
Create two threads that will exchange the data.
Thread thread1 = new Thread(() -> { try { String data1 = "Thread 1"; String exchangedData = exchanger.exchange(data1); System.out.println("Thread 1 received: " + exchangedData); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } }); Thread thread2 = new Thread(() -> { try { String data2 = "Thread 2"; String exchangedData = exchanger.exchange(data2); System.out.println("Thread 2 received: " + exchangedData); } catch (InterruptedException e) { Thread.currentThread().interrupt(); } });
-
Start the threads.
thread1.start(); thread2.start();
The exchange
method will block until both threads have reached the exchange point. Once both threads have arrived, they swap their data and continue execution.
Common Pitfalls When Using Exchanger
1. Deadlock
Using Exchanger
can lead to deadlock if the exchange operation is not properly handled. Deadlock can occur if one thread calls exchange
while the other thread is not yet ready to perform the exchange. To avoid this, ensure that both threads reach the exchange point before calling the exchange
method.
2. Unequal Exchange
It's crucial to ensure that both threads exchange the same type of data. If one thread provides a different type of data than expected, it can lead to unexpected behavior in the program. Always validate the exchanged data to avoid runtime issues.
3. Handling Interrupts
Properly handling interrupts is essential when using Exchanger
. If a thread gets interrupted while waiting at the exchange point, it's crucial to handle the InterruptedException
to avoid leaving the Exchanger
in an inconsistent state.
4. Resource Management
Ensure that any resources associated with the exchanged data are properly managed. Improper resource management can lead to resource leaks and potential application instability.
Synchronization Best Practices
Use a Timeout
Consider using a timeout when calling the exchange
method to prevent indefinite blocking. By specifying a timeout value, you can gracefully handle situations where the exchange operation takes longer than expected.
String exchangedData = exchanger.exchange(data, timeout, timeUnit);
Error Handling
Always handle potential exceptions thrown by the exchange
method. Failing to handle exceptions can lead to unanticipated behavior and potential application crashes.
try {
String exchangedData = exchanger.exchange(data);
// Handle exchanged data
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
// Handle interrupt
}
Data Validation
Before using the exchanged data, validate its integrity and type to avoid runtime errors.
String exchangedData = exchanger.exchange(data);
// Validate exchanged data
if (isValid(exchangedData)) {
// Process data
}
Resource Cleanup
If the exchanged data involves resources such as file handles or database connections, ensure proper cleanup to prevent resource leaks.
try {
String exchangedData = exchanger.exchange(data);
// Process data
} finally {
// Clean up resources
}
The Closing Argument
In conclusion, the Exchanger
in Java is a powerful synchronization aid that facilitates data exchange between threads. However, using Exchanger
effectively requires careful consideration of potential pitfalls such as deadlock, unequal exchange, interrupt handling, and resource management. By following the best practices outlined in this post, you can leverage the Exchanger
effectively while avoiding common synchronization pitfalls.
By mastering the use of Exchanger
, you can enhance the coordination and communication between threads in your Java applications, leading to more robust and efficient concurrent programming.
To delve deeper into Java concurrency and synchronization, consider exploring the official Java documentation on Concurrency.
Checkout our other articles