Unlocking Step-Variant Models: Boost Your AI's Flexibility
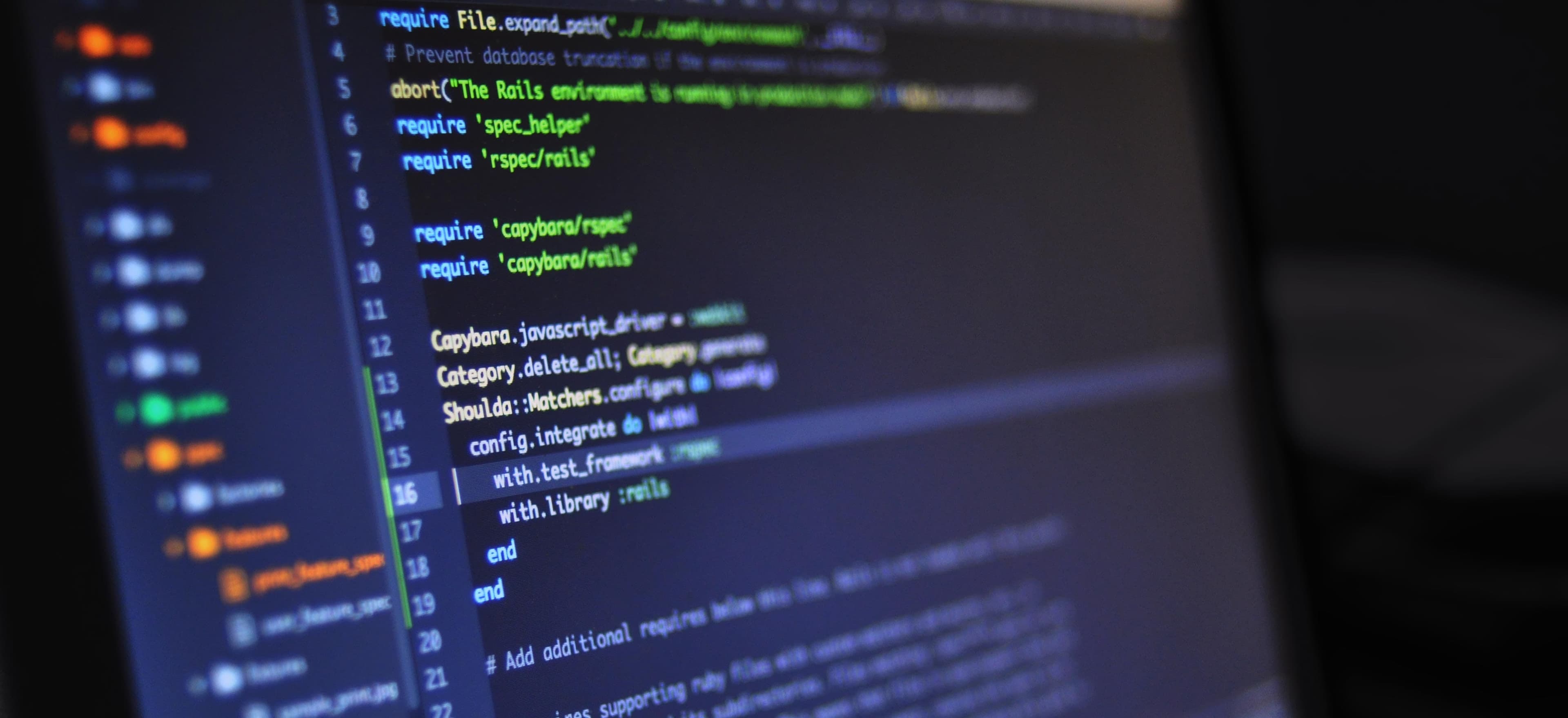
- Published on
Unlocking Step-Variant Models: Boost Your AI's Flexibility
In the world of artificial intelligence, flexibility and adaptability are key. One way to achieve this is through the implementation of step-variant models in your Java applications. Step-variant models allow for the creation of highly flexible and dynamic AI systems that can adapt to a wide range of inputs and scenarios. In this article, we will explore the concept of step-variant models, their implementation in Java, and the benefits they can bring to your AI applications.
Understanding Step-Variant Models
Step-variant models, also known as stepwise regression models, are a type of regression analysis where the relationship between the independent variables and the dependent variable is modeled as a series of steps or segments. This approach allows for greater flexibility in modeling complex relationships that may not be captured effectively by traditional linear or non-linear models.
Why Step-Variant Models?
Traditional regression models assume a fixed relationship between the independent and dependent variables, which may not hold true in many real-world scenarios. Step-variant models provide a more adaptable framework that can better capture the nuances and complexities of the data, leading to more accurate predictions and insights.
Implementing Step-Variant Models in Java
Now, let's dive into the practical implementation of step-variant models in Java. We'll be using the Apache Commons Math library, which provides comprehensive mathematical and statistical functionality for Java applications.
// Import necessary classes from Apache Commons Math
import org.apache.commons.math3.analysis.polynomials.PolynomialFunction;
import org.apache.commons.math3.fitting.WeightedObservedPoints;
import org.apache.commons.math3.fitting.WeightedObservedPolynomialCurveFitter;
import org.apache.commons.math3.fitting.WeightedObservedPoints;
public class StepVariantModel {
public static void main(String[] args) {
// Create a set of observed data points
WeightedObservedPoints obs = new WeightedObservedPoints();
// Add observed data points
obs.add(x1, y1);
obs.add(x2, y2);
// ...
// Create a polynomial curve fitter
WeightedObservedPolynomialCurveFitter fitter = WeightedObservedPolynomialCurveFitter.create(degree);
// Fit the observed data points with the polynomial curve fitter
double[] coefficients = fitter.fit(obs.toList());
// Create a polynomial function with the fitted coefficients
PolynomialFunction fittedFunction = new PolynomialFunction(coefficients);
// Use the fitted function for prediction or analysis
double predictedValue = fittedFunction.value(x);
}
}
In the above example, we use the Apache Commons Math library to create a step-variant model by fitting a polynomial curve to observed data points. The WeightedObservedPoints
class is used to store the observed data, and the WeightedObservedPolynomialCurveFitter
class is used to fit a polynomial curve to the data. The fitted polynomial function can then be used for making predictions or further analysis.
Benefits of Step-Variant Models in Java
By implementing step-variant models in Java, you can unlock a range of benefits for your AI applications:
-
Flexibility: Step-variant models allow your AI system to adapt to changing data patterns and relationships, leading to more robust and accurate predictions.
-
Complexity Handling: With step-variant models, you can effectively handle complex and non-linear relationships in your data, providing a more comprehensive analysis.
-
Customization: The flexibility of step-variant models allows for easy customization and adaptation to specific use cases and scenarios, making them highly versatile.
The Last Word
In conclusion, step-variant models offer a powerful approach to increasing the flexibility and adaptability of AI systems in Java. By leveraging the capabilities of step-variant models, you can build AI applications that are better equipped to handle diverse and dynamic data patterns, leading to improved accuracy and reliability. Incorporating step-variant models using libraries such as Apache Commons Math can elevate the capabilities of your AI systems, paving the way for more effective and efficient decision-making.
By embracing step-variant models, you are arming your AI with the agility it needs to navigate the complexities of real-world data, giving it the freedom to adjust and improve as it learns and evolves.
Start integrating step-variant models into your Java applications today and unleash the full potential of your AI systems!
For more in-depth information on step-variant models, you can refer to this article for further insights.
Remember, flexibility is the cornerstone of adaptability, and step-variant models are the key to unlocking your AI's potential.
Checkout our other articles