Seamlessly Call Plutus v2 Contracts with Java: A How-To Guide
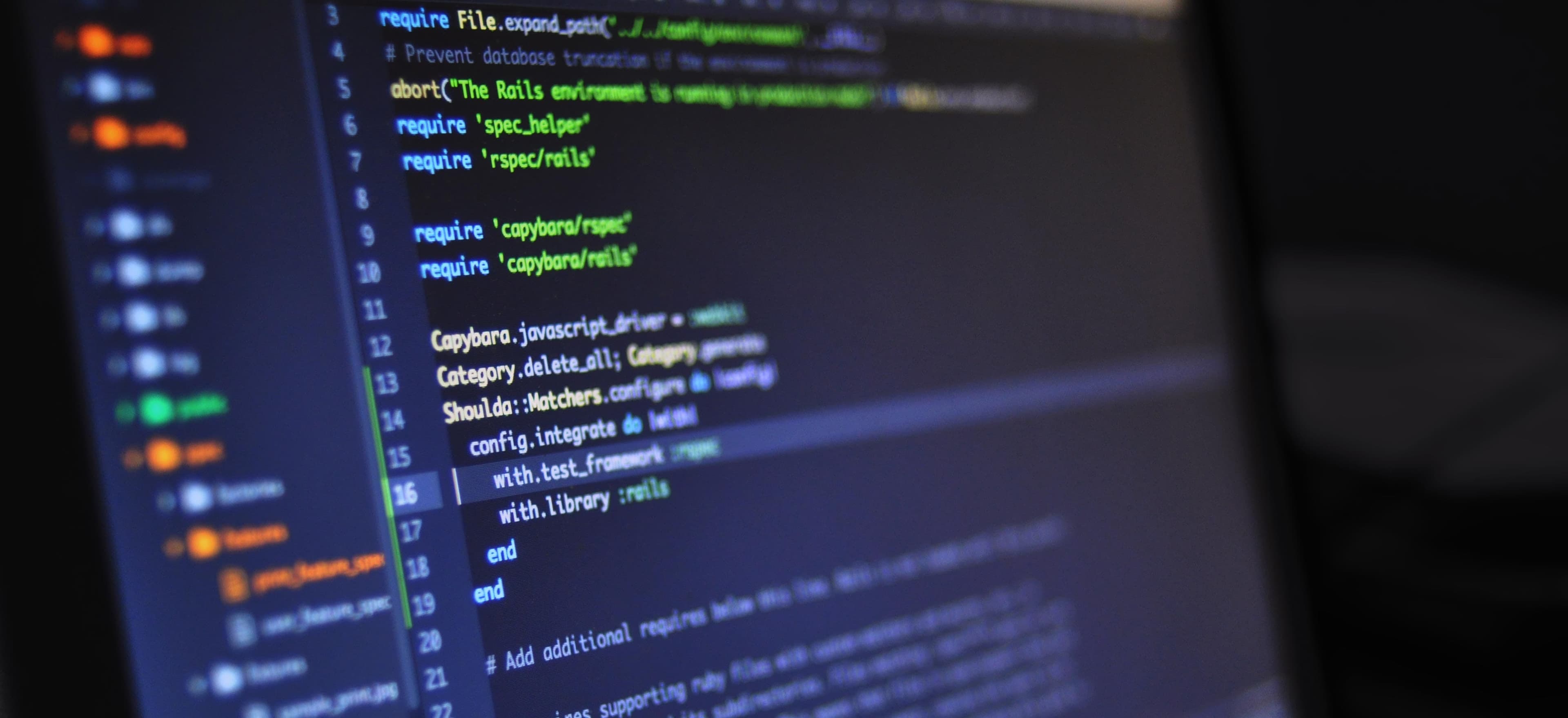
- Published on
Seamlessly Call Plutus v2 Contracts with Java: A How-To Guide
Blockchain development has gained significant attention in recent years, with smart contracts being a crucial element of decentralized applications. Cardano, a leading blockchain platform, has introduced Plutus, a smart contract platform that allows developers to write secure and composable contracts in Haskell. With the release of Plutus v2, interacting with Plutus contracts using Java has become more seamless. In this guide, we will walk through the process of calling Plutus v2 contracts using Java and explore the key concepts and code snippets to facilitate the understanding of this integration.
Prerequisites
Before diving into interacting with Plutus v2 contracts using Java, it is essential to have a basic understanding of blockchain technology, smart contracts, and Java programming. Additionally, familiarity with Cardano's Plutus platform and the basic concepts of Plutus scripting language would be beneficial. To follow along with the code examples, you will need to have Java Development Kit (JDK) and an Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse installed on your machine.
Setting Up the Project
To begin, let's create a new Java project in your chosen IDE. Once the project is set up, we need to add the necessary dependencies to interact with the Cardano blockchain and Plutus contracts. Maven is a popular build automation tool for Java projects, and we can use it to manage our project dependencies.
Add the following dependencies to your pom.xml
file:
<dependencies>
<dependency>
<groupId>io.github.cottontail</groupId>
<artifactId>cardano-client-lib</artifactId>
<version>4.0.0</version>
</dependency>
</dependencies>
This cardano-client-lib
dependency provides the necessary Java bindings to interact with the Cardano blockchain and Plutus contracts.
Interacting with Plutus v2 Contracts
Now that we have set up our project and added the required dependencies, let's delve into the process of interacting with Plutus v2 contracts using Java. The typical workflow involves creating transactions, composing the necessary inputs and outputs, and submitting the transaction to the blockchain.
1. Constructing a Transaction
In the Cardano blockchain, transactions are at the core of interacting with smart contracts. To create a transaction in Java, we utilize the TransactionBuilder
provided by the cardano-client-lib
. Here's an example of how you can construct a simple transaction:
TransactionBuilder transactionBuilder = new TransactionBuilder();
transactionBuilder.addInput(...); // Add input
transactionBuilder.addOutput(...); // Add output
In this code snippet, we create a new TransactionBuilder
instance and add inputs and outputs to the transaction. Inputs typically refer to the funds being spent, while outputs represent where the funds are being sent.
2. Signing the Transaction
Once the transaction is constructed, it needs to be signed to authorize the transfer of funds. We can use the Wallet
and PrivateKey
classes from the cardano-client-lib
to sign the transaction:
PrivateKey privateKey = Wallet.loadPrivateKey("path/to/private_key_file");
SignedTransaction signedTransaction = transactionBuilder.buildAndSign(privateKey);
Here, we load the private key from a file and use it to sign the transaction, resulting in a SignedTransaction
ready for submission to the blockchain.
3. Submitting the Transaction
The final step involves submitting the signed transaction to the Cardano blockchain. The CardanoClient
class from the cardano-client-lib
provides the necessary methods to submit the transaction:
CardanoClient cardanoClient = new CardanoClient();
cardanoClient.submitTransaction(signedTransaction);
By invoking the submitTransaction
method with the signed transaction, we broadcast the transaction to the Cardano network for processing.
Lessons Learned
In this guide, we have covered the process of seamlessly calling Plutus v2 contracts with Java. We set up a new Java project, added the required dependencies, and walked through the essential steps of interacting with Plutus contracts, including constructing a transaction, signing the transaction, and submitting it to the blockchain. As blockchain technology continues to evolve, the ability to interact with smart contracts using Java opens up new possibilities for developers seeking to integrate blockchain functionality into their applications.
Interacting with Plutus v2 contracts using Java can introduce a range of opportunities for developers, and mastering this integration can prove to be a valuable skill in the blockchain development landscape.
By following the steps outlined in this guide and experimenting with the provided code snippets, you can gain a solid understanding of calling Plutus v2 contracts with Java, setting the stage for further exploration and innovation in blockchain application development.
To further advance your knowledge, consider exploring advanced features, such as working with complex contract scripts, handling contract parameters, and integrating off-chain data into Plutus contracts.
With the continuous advancement of blockchain technology and the expanding capabilities of smart contract platforms like Plutus, developers equipped with the know-how of interacting with contracts using Java are well-positioned to contribute to the growth of decentralized applications and blockchain ecosystems.
In conclusion, the seamless integration of Java with Plutus v2 contracts paves the way for a new wave of blockchain-powered applications, and mastering this integration can unlock a myriad of possibilities for developers venturing into the realm of decentralized finance, non-fungible tokens, decentralized exchanges, and beyond.
Now that you have a solid understanding of the process, it's time to unleash your creativity and embark on your journey of leveraging Plutus v2 contracts with Java. Happy coding!