Troubleshooting Transcending REST and RPC
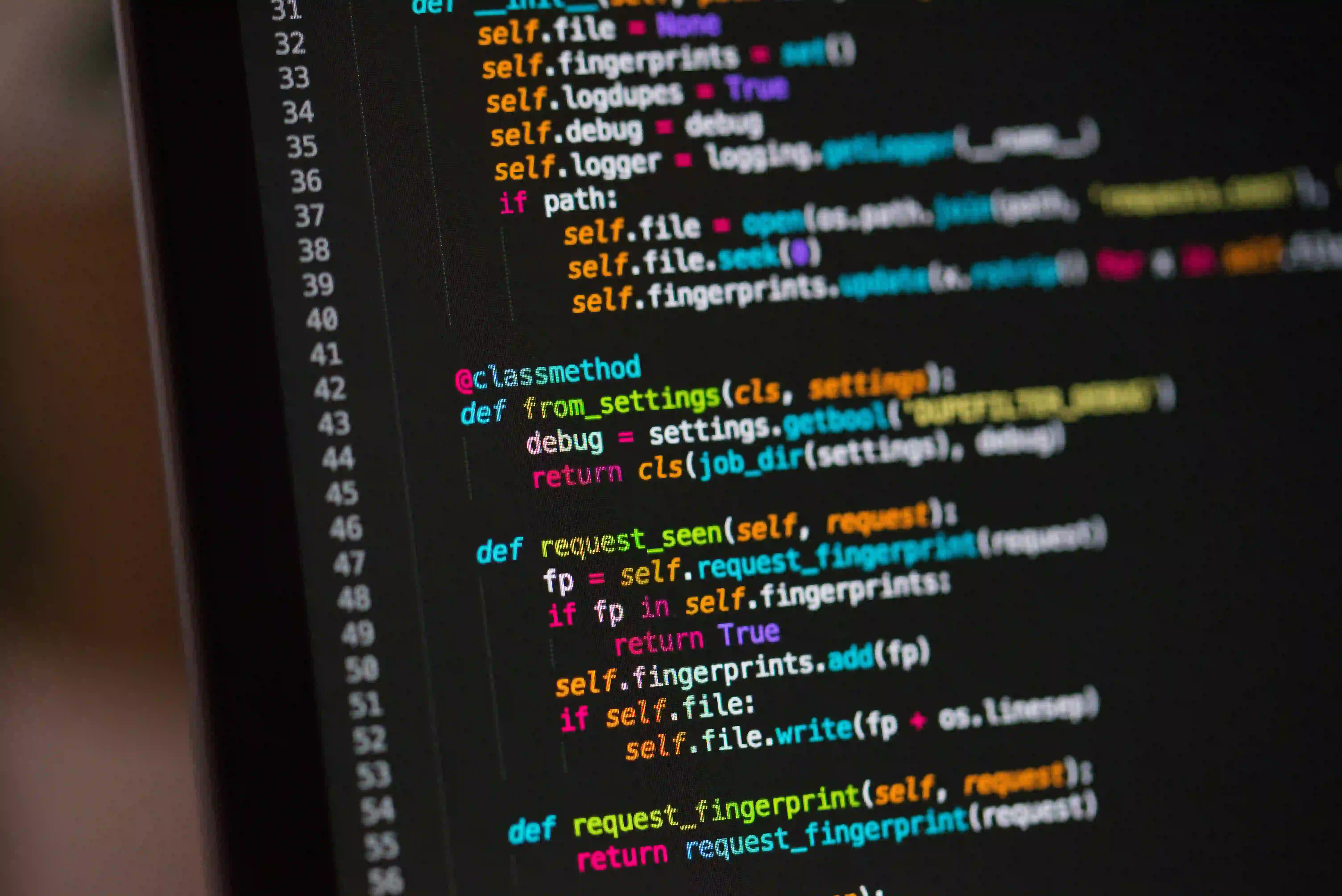
Understanding and Troubleshooting in Java
When it comes to building a robust and reliable Java application, understanding and troubleshooting different aspects of the system is crucial. In this blog post, we will delve into the concepts of REST and RPC (Remote Procedure Call) and explore some common troubleshooting techniques in Java.
Understanding REST and RPC
REST
REST, which stands for Representational State Transfer, is an architectural style for designing networked applications. It relies on a stateless, client-server communication protocol, most commonly HTTP. In a RESTful system, resources are identified by URIs, and interactions are performed using standard HTTP methods such as GET, POST, PUT, DELETE, etc.
When working with RESTful services in Java, it's essential to understand how to create and consume RESTful APIs using frameworks like Spring and Jersey. By understanding these frameworks and their underlying principles, developers can effectively troubleshoot issues related to REST API design, implementation, and consumption.
RPC
On the other hand, Remote Procedure Call (RPC) is a communication protocol that allows a program to execute code on a remote server. In the context of Java, RPC frameworks like Apache Thrift and gRPC are widely used for building distributed systems. Troubleshooting RPC-related issues involves understanding the underlying communication protocols, serialization, and deserialization mechanisms.
Troubleshooting Techniques in Java
Now that we have a basic understanding of REST and RPC, let's explore some common troubleshooting techniques in Java for dealing with issues related to these communication protocols.
1. Debugging and Logging
One of the first steps in troubleshooting any Java application is thorough logging and debugging. By using logging frameworks like Log4j or SLF4J, developers can instrument their code to capture relevant information about the execution flow, including HTTP requests/responses, RPC calls, and potential errors. This helps in identifying the root cause of issues and understanding the flow of data through the system.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyClass {
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public void myMethod() {
logger.debug("Inside myMethod");
// ... rest of the code
}
}
Here, the use of logging allows us to track the execution of myMethod
and any related issues, providing insights into the application's behavior.
2. Network Traffic Analysis
When troubleshooting communication-related issues in Java, analyzing network traffic can provide valuable insights. Tools like Wireshark and Fiddler can be used to capture and analyze HTTP requests/responses, RPC payloads, and network-level issues such as connection timeouts, DNS resolution problems, and packet loss.
By inspecting the network traffic, developers can identify potential bottlenecks, malformed requests/responses, or unexpected behavior in their RESTful or RPC communications.
3. Unit Testing and Mocking
Effective unit testing plays a crucial role in troubleshooting Java applications. When dealing with REST and RPC, writing unit tests for API endpoints and RPC services can help in validating the expected behavior and identifying edge cases or erroneous inputs. Mocking frameworks like Mockito can be used to simulate RESTful or RPC interactions and test various scenarios without relying on actual network communication.
import static org.mockito.Mockito.when;
@RunWith(MockitoJUnitRunner.class)
public class MyServiceTest {
@Mock
private RestClient client;
@InjectMocks
private MyService myService;
@Test
public void testApiCallSuccess() {
when(client.getData()).thenReturn("mockedData");
String result = myService.invokeApi();
assertEquals("ExpectedResult", result);
}
}
In this example, MyService
is tested by mocking the RestClient
to simulate API calls and assert the expected results, thus facilitating early detection of potential issues.
4. Exception Handling and Error Codes
Proper exception handling and consistent error code management are essential for troubleshooting REST and RPC issues. When designing RESTful APIs or RPC services, it's imperative to define clear error codes and messages for different failure scenarios, making it easier to diagnose and address issues when they occur.
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/api/data")
public ResponseEntity<String> getData() {
try {
String data = myService.getData();
return ResponseEntity.ok(data);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("Internal Server Error");
}
}
}
In this RESTful controller, any exceptions thrown by MyService
can be caught and translated into appropriate HTTP error responses, providing meaningful feedback to clients and aiding in troubleshooting.
Closing Remarks
Troubleshooting in Java, particularly in the context of REST and RPC, requires a combination of technical knowledge, practical skills, and the right set of tools. By incorporating logging, network analysis, unit testing, and robust error handling, developers can effectively identify and address issues related to communication protocols in their Java applications.
Understanding the intricacies of RESTful and RPC communications, along with the ability to troubleshoot and debug these interactions, is essential for ensuring reliable and efficient distributed systems in Java.
In conclusion, troubleshooting transcending REST and RPC involves not just identifying and resolving issues, but also gaining a deeper understanding of the underlying principles and best practices that govern these communication paradigms.
By mastering the art of troubleshooting in Java, developers can elevate the reliability and performance of their applications, while also honing their expertise in building robust distributed systems.
For further insights into REST and RPC, please refer to this comprehensive guide and this in-depth article.
Happy troubleshooting!