JMS 2.0 Simplified: Navigating Different JMSContext Types
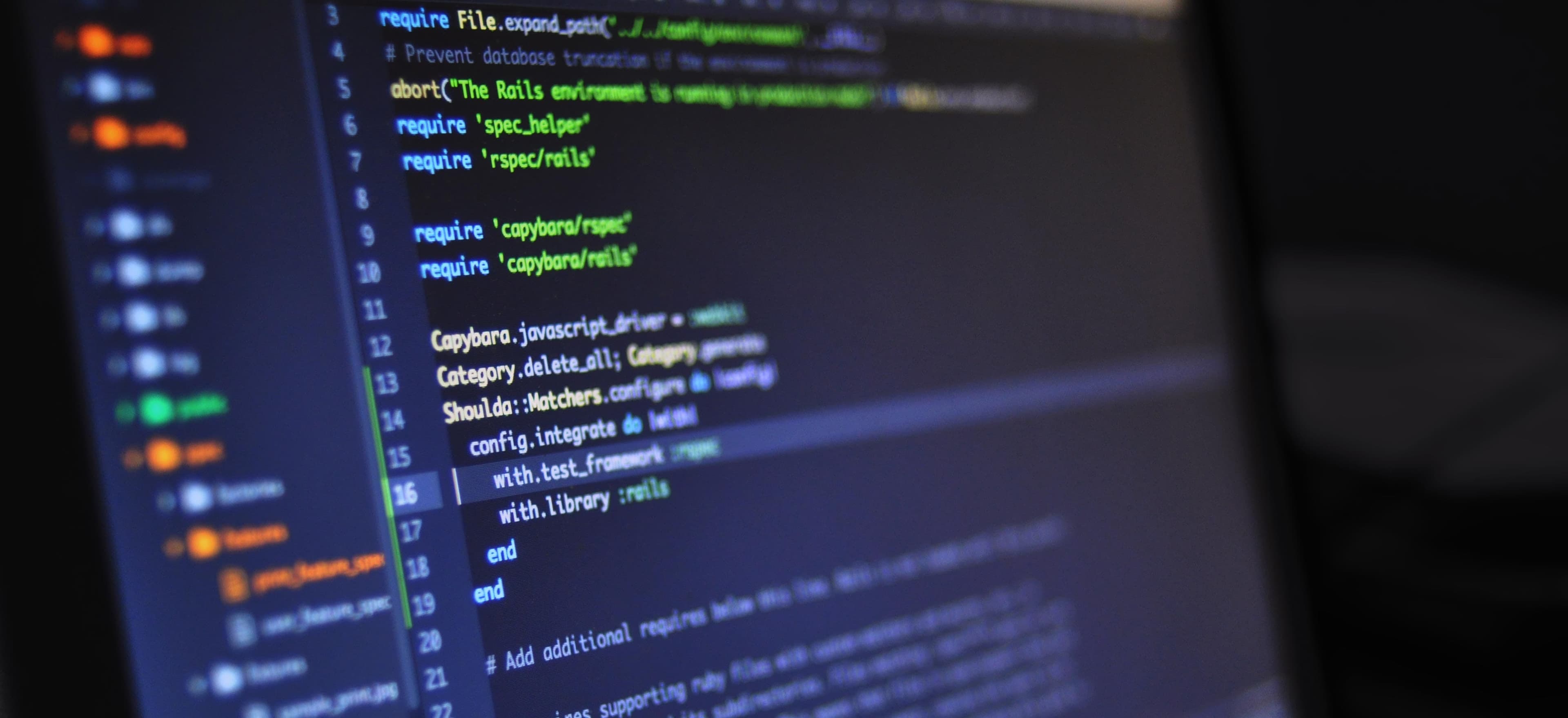
- Published on
JMS 2.0 Simplified: Navigating Different JMSContext Types
Java Message Service (JMS) is a widely used API that allows applications to create, send, receive, and read messages. With the release of JMS 2.0, the API has become even more developer-friendly, offering simplified methods for interacting with messaging systems.
In this post, we'll dive into one of the key features of JMS 2.0 - the JMSContext
class. We will explore the different types of JMSContext
and how they simplify the process of working with JMS.
Understanding JMSContext
In JMS 2.0, the JMSContext
class serves as the primary entry point for creating JMS resources and sending/receiving messages. It encapsulates the functionality of creating and managing the JMS objects, making the overall experience more streamlined and intuitive.
Creating a JMSContext
Creating a JMSContext
is straightforward and follows a simple pattern. Let's take a look at a basic example of creating a JMSContext
:
import javax.jms.ConnectionFactory;
import javax.jms.JMSContext;
import javax.naming.InitialContext;
import javax.naming.NamingException;
public class JMSContextExample {
public static void main(String[] args) {
try {
InitialContext initialContext = new InitialContext();
ConnectionFactory connectionFactory = (ConnectionFactory) initialContext.lookup("jms/MyConnectionFactory");
try (JMSContext jmsContext = connectionFactory.createContext()) {
// Use jmsContext to send/receive messages
}
} catch (NamingException e) {
e.printStackTrace();
}
}
}
In this example, we obtain a ConnectionFactory
through JNDI lookup and then create a JMSContext
using the createContext
method. The try-with-resources statement ensures that the JMSContext
is closed after its use.
Types of JMSContext
JMS 2.0 introduces two types of JMSContext
- the Auto-Closable JMSContext and the Transactional JMSContext. Understanding the differences between these types is essential for leveraging the full potential of JMS in your applications.
Auto-Closable JMSContext
The Auto-Closable JMSContext, as the name suggests, automatically closes the resources when it goes out of scope. It simplifies resource management by eliminating the need for explicit resource cleanup.
Let's see how the Auto-Closable JMSContext simplifies code:
try (JMSContext jmsContext = connectionFactory.createContext()) {
// Use jmsContext to send/receive messages
} // No need to explicitly call jmsContext.close()
In the above code, the try-with-resources statement ensures that the JMSContext
is automatically closed at the end of the block, leading to cleaner and more concise code.
Transactional JMSContext
The Transactional JMSContext, on the other hand, provides support for JMS transactions. It enables the grouping of messaging operations into atomic units, ensuring that either all operations within a transaction succeed or none of them take effect.
Let's examine a simple example of using Transactional JMSContext:
try (JMSContext jmsContext = connectionFactory.createContext(JMSContext.SESSION_TRANSACTED)) {
// Send/receive messages within a transaction
jmsContext.createProducer().send(destination, message);
// Commit the transaction
jmsContext.commit();
} // The transaction is committed or rolled back automatically
In this example, we create a JMSContext
with transactional support by passing JMSContext.SESSION_TRANSACTED
to the createContext
method. We then send a message and explicitly commit the transaction. If an exception occurs or the block finishes execution without committing, the transaction is automatically rolled back, ensuring data consistency.
Understanding when and how to use each type of JMSContext
is crucial for building robust and efficient messaging systems.
Closing the Chapter
In this post, we've explored the simplified approach of working with JMS 2.0, focusing specifically on the different types of JMSContext
and how they enhance the development experience. By mastering the nuances of JMSContext
, developers can leverage its capabilities to build scalable and reliable messaging solutions.
To delve deeper into JMS 2.0 and its features, take a look at the official Java EE JMS documentation and the JMS 2.0 specification.
Happy messaging with JMS 2.0!
Checkout our other articles