Master Type-Safe Hibernate Queries with Java Streams
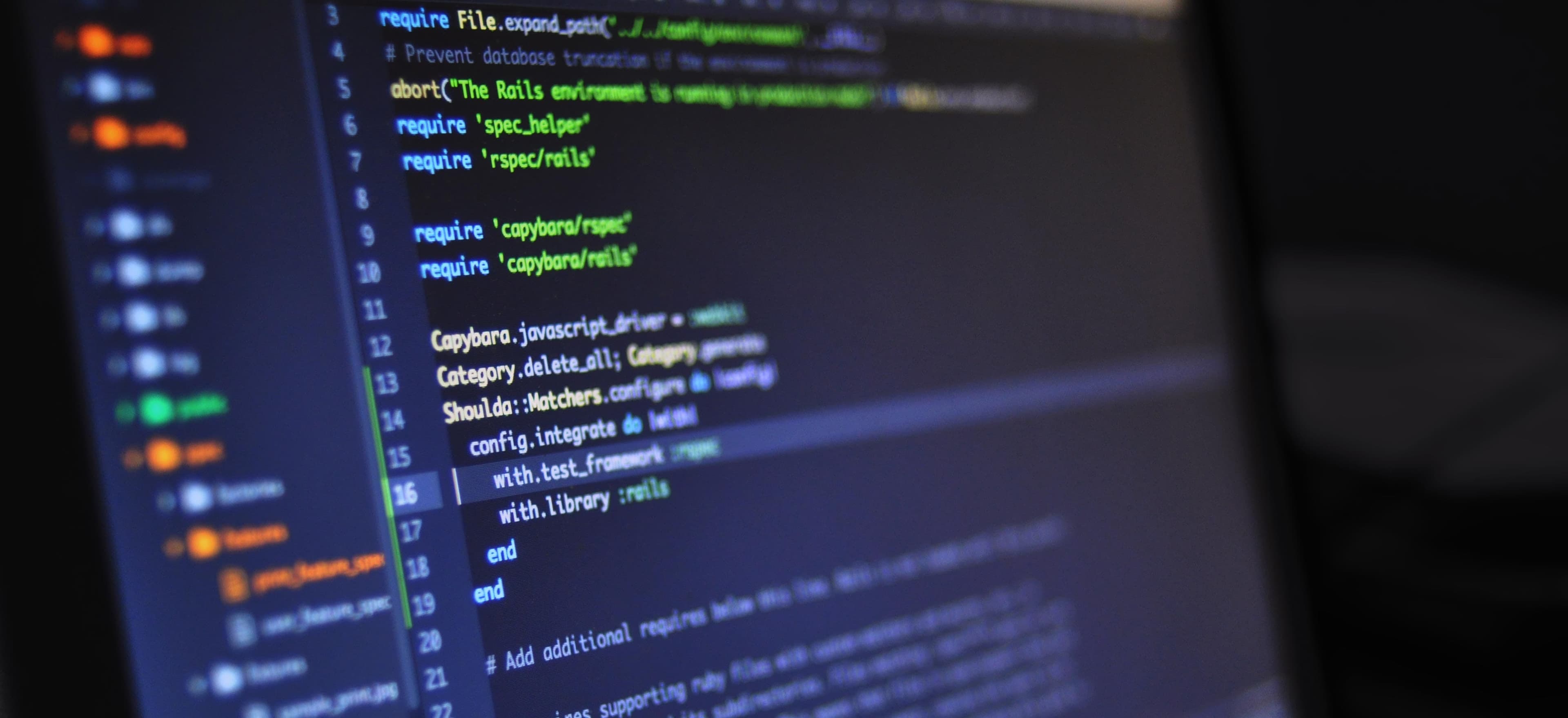
- Published on
Master Type-Safe Hibernate Queries with Java Streams
When it comes to data access in Java applications, Hibernate is a powerful tool widely utilized for object-relational mapping (ORM). It abstracts the database interaction and allows developers to work with data in a more intuitive way. However, crafting type-safe queries has often presented challenges, especially for developers transitioning from traditional SQL to the Hibernate Criteria API.
In this blog post, we'll delve deep into the intricacies of type-safe Hibernate queries using Java Streams. By the end, you’ll gain a clear understanding of how to leverage these technologies to write cleaner, safer, and more maintainable code.
Why Type-Safe Queries Matter
Type-safety in queries means that errors related to database fields, such as typos or mismatched data types, are caught at compile time rather than runtime. This can significantly reduce the debugging overhead and make your code more robust.
Imagine a scenario where your query references a nonexistent field in your entity. If you're using raw strings to define your queries, you won't discover this mistake until the code is executed, possibly leading to runtime exceptions. Type-safe alternatives allow the compiler to enforce correctness, safeguarding developer intentions.
Getting Started: Setting Up Hibernate
Before we proceed, ensure you have the following in your setup:
- Java Development Kit (JDK) 11 or higher.
- Dependency management tool like Maven or Gradle.
- Hibernate dependencies included in your project (along with a database connector).
Here is a sample Maven dependency for Hibernate 5.x with H2 Database:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.32.Final</version>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<version>1.4.200</version>
</dependency>
Defining Entities
For our example, let's define a simple User
entity. This class serves as an ORM entity that Hibernate will manage:
import javax.persistence.*;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String userName;
@Column(name = "email")
private String email;
// Constructors, getters, and setters
public User(String userName, String email) {
this.userName = userName;
this.email = email;
}
// Getters and setters omitted for brevity
}
Why use Annotations?
Annotations serve as metadata for the User
class. They instruct Hibernate on how to map the entity to the database, enabling smoother interactions.
Creating a Basic Hibernate Configuration
We'll use a configuration file or programmatic configuration to set up our Hibernate session factory. Here’s a simple example using a configuration file named hibernate.cfg.xml
:
<hibernate-configuration>
<session-factory>
<property name="hibernate.dialect">org.hibernate.dialect.H2Dialect</property>
<property name="hibernate.connection.driver_class">org.h2.Driver</property>
<property name="hibernate.connection.url">jdbc:h2:mem:testdb</property>
<property name="hibernate.hbm2ddl.auto">update</property>
<property name="hibernate.show_sql">true</property>
</session-factory>
</hibernate-configuration>
Starting a Session
Here’s how to boot up your Hibernate session:
import org.hibernate.SessionFactory;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
public class HibernateUtil {
private static final SessionFactory sessionFactory = buildSessionFactory();
private static SessionFactory buildSessionFactory() {
try {
return new Configuration().configure().buildSessionFactory();
} catch (Throwable ex) {
throw new ExceptionInInitializerError(ex);
}
}
public static Session getSession() {
return sessionFactory.openSession();
}
}
Type-Safe Queries with Criteria API and Java Streams
Now that our environment is ready, let’s explore how to write type-safe queries using the Hibernate Criteria API along with Java Streams.
Step 1: Constructing a Criteria Query
Let’s create a method that retrieves users by their username:
import org.hibernate.Session;
import org.hibernate.query.Query;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
import java.util.List;
public class UserDAO {
public List<User> getUsersByUserName(String userName) {
Session session = HibernateUtil.getSession();
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<User> cq = cb.createQuery(User.class);
Root<User> userRoot = cq.from(User.class);
cq.select(userRoot).where(cb.equal(userRoot.get("userName"), userName));
Query<User> query = session.createQuery(cq);
return query.getResultList();
}
}
Explanation:
- CriteriaBuilder: This is the starting point for criteria queries. It creates various criteria objects (like predicates).
- CriteriaQuery: Represents the query itself and allows you to specify the result type being queried for.
- Root: This represents the entity/table we are querying against.
- Predicate: The
where
clause uses a type-safe reference to theuserName
field while filtering.
Step 2: Using Java Streams
To further enhance our functionality, we will apply Java Streams to process results. Here’s how you could extend the getUsersByUserName
method to filter out inactive users from the result list:
import java.util.stream.Collectors;
public class UserDAO {
public List<User> getActiveUsersByUserName(String userName) {
List<User> users = getUsersByUserName(userName);
return users.stream()
.filter(this::isUserActive) // Assuming we have an isUserActive method
.collect(Collectors.toList());
}
private boolean isUserActive(User user) {
// Add logic to check if the user is active, considering some attribute in User.
return true; // Placeholder implementation
}
}
Why Use Streams?
Java Streams facilitate expressive and flexible operations over collections. They allow you to filter, map, and collect data elements in a clean and functional style, making your code easier to read and maintain.
Inserting a User into the Database
Handling Transactions
Ensuring data integrity requires you to handle your session and transactions properly. Here's an example method for adding a User:
public void addUser(User user) {
Session session = HibernateUtil.getSession();
session.beginTransaction();
session.save(user);
session.getTransaction().commit();
session.close();
}
Querying with Specifications
To extend our querying capabilities, we can also make use of well-defined specifications. This design pattern allows us to encapsulate the logic for different queries.
Let's define a specification for locating users based on their email:
import org.hibernate.Criteria;
import org.hibernate.Session;
public class UserSpecifications {
public Criteria getUserByEmail(Session session, String email) {
Criteria criteria = session.createCriteria(User.class);
criteria.add(Restrictions.eq("email", email));
return criteria;
}
}
Final Thoughts
Type-safe Hibernate queries using Java Streams significantly enhance the safety and maintainability of your data access layer. Not only do you minimize the risk of runtime errors, but over time, your codebase becomes more intuitive for both current and future developers.
As you advance further with Hibernate and Java, consider taking a look at more advanced topics like JPA Criteria Queries with Joins, pagination, and even integrating Spring Data JPA for seamless database operations.
For more information, check out the Hibernate User Guide and Stream API documentation.
Happy coding!
Checkout our other articles