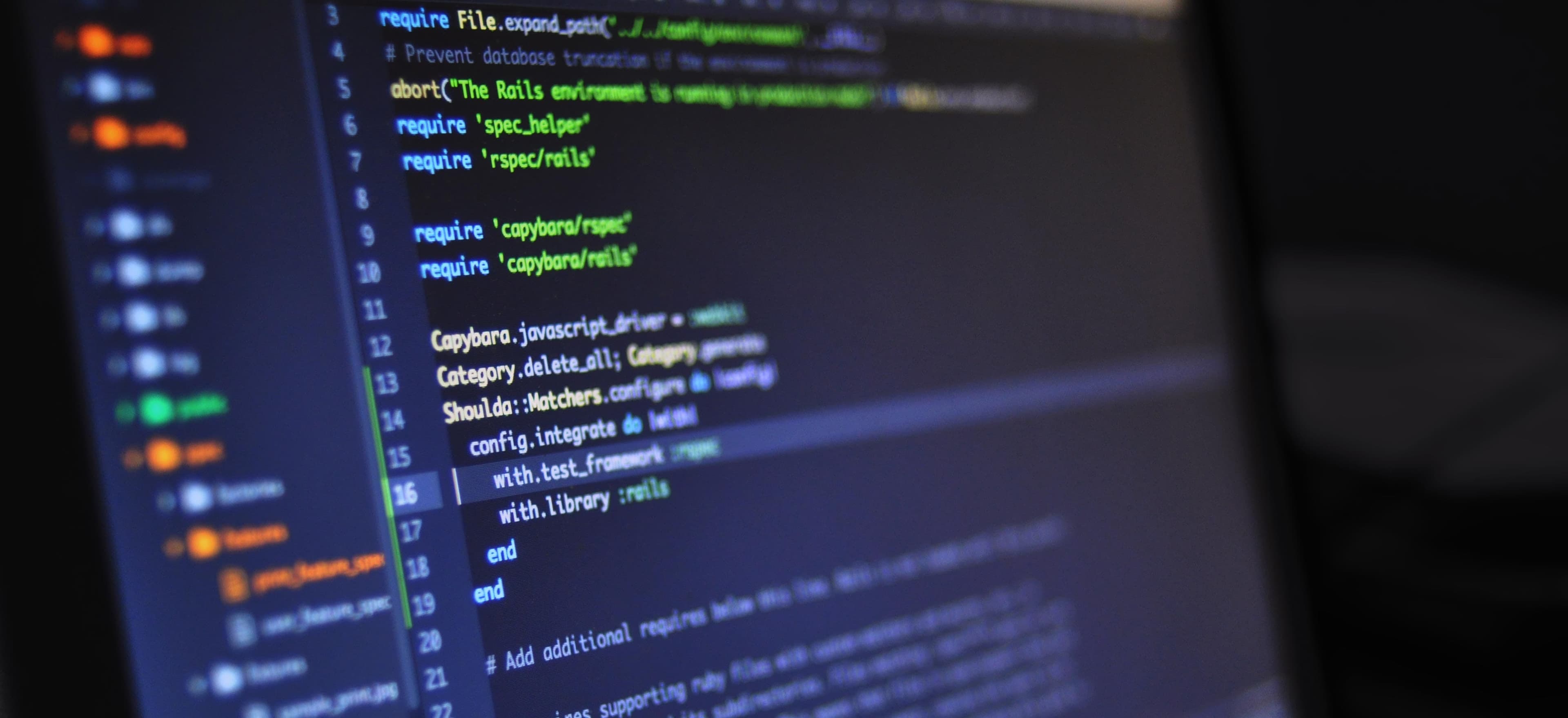
- Published on
Thwarting Cyber Threats: Secure JavaServer Faces (JSF) Apps on JBoss AS 7.x
In today's digital landscape, the security of web applications is a paramount concern for organizations and developers. JavaServer Faces (JSF) is a popular framework for building user interfaces for Java web applications. When deploying JSF apps on JBoss AS 7.x, it's crucial to implement robust security measures to protect against cyber threats and vulnerabilities.
In this comprehensive guide, we'll delve into essential security practices for JSF applications running on JBoss AS 7.x. We'll cover a range of security considerations, from preventing common web application vulnerabilities to configuring secure communication protocols.
Securing JSF Applications
Cross-Site Scripting (XSS) Mitigation
Cross-site scripting (XSS) is a prevalent security vulnerability that can compromise the integrity of JSF applications. Mitigating XSS involves validating and sanitizing user input to prevent malicious script injection.
<h:outputText value="#{userInput}" escape="false" />
In the above code snippet, the escape="false"
attribute is used to disable HTML escaping for the userInput
variable. While this may be necessary in specific scenarios, it introduces XSS vulnerabilities. To mitigate XSS, use the following:
<h:outputText value="#{userInput}" escape="true" />
By setting escape="true"
, the JSF framework automatically escapes the user input, rendering it safe from XSS attacks.
Input Validation and Data Sanitization
JSF provides built-in validation and conversion features that help enforce strict input requirements and sanitize user data. When defining input fields, utilize JSF components such as <h:inputText>
and <f:validateLength>
to enforce length constraints:
<h:inputText id="username" value="#{userBean.username}" required="true">
<f:validateLength maximum="50" />
</h:inputText>
In the above example, the <f:validateLength>
tag ensures that the length of the username
input does not exceed 50 characters, mitigating potential input-based attacks.
Protecting Against SQL Injection
SQL injection is a serious threat to the security of web applications. When interacting with databases in JSF applications, it's crucial to use parameterized queries and prepared statements to prevent SQL injection attacks.
String query = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement statement = connection.prepareStatement(query);
statement.setString(1, userInputUsername);
statement.setString(2, userInputPassword);
ResultSet resultSet = statement.executeQuery();
In the above code snippet, connection.prepareStatement()
and the subsequent setString()
calls introduce parameterized queries, safeguarding the application against SQL injection.
Configuring Secure Communication
Enforcing HTTPS
Securing data transmission between clients and the server is imperative for safeguarding sensitive information. To enforce HTTPS in JSF applications on JBoss AS 7.x, modify the web.xml
deployment descriptor to include the following security constraints:
<web-app>
...
<security-constraint>
<web-resource-collection>
<web-resource-name>Secure Pages</web-resource-name>
<url-pattern>/*</url-pattern>
</web-resource-collection>
<user-data-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</user-data-constraint>
</security-constraint>
...
</web-app>
With the above configuration, all web requests are constrained to use the CONFIDENTIAL
transport guarantee, ensuring that data is transmitted over HTTPS.
Securing JBoss AS 7.x
Restricting Access to Administration Console
The JBoss AS 7.x administration console provides powerful management capabilities for the application server. However, it's imperative to restrict access to the console to prevent unauthorized configuration changes and potential security breaches.
To restrict access to the administration console, configure the mgmt-users.properties
and mgmt-groups.properties
files in the JBOSS_HOME/standalone/configuration
directory. Create user accounts with specific roles to control access privileges.
Implementing Role-Based Access Control
Role-based access control (RBAC) is essential for governing user permissions within JSF applications. JBoss AS 7.x supports RBAC through the use of security realms and role mappings.
By defining security realms in the standalone.xml
configuration file and mapping roles to specific users or groups, developers can enforce granular access control policies within their JSF applications.
Final Thoughts
Securing JavaServer Faces applications on JBoss AS 7.x demands a multifaceted approach encompassing input validation, data sanitization, secure communication, and server-level security configurations. By adhering to best practices and leveraging the robust security features of the JSF framework and JBoss AS 7.x, developers can fortify their applications against an array of cyber threats.
In an era of escalating cyber threats, the imperative of securing JSF applications on JBoss AS 7.x cannot be overstated. Embracing a proactive security stance will fortify web applications and contribute to overall cybersecurity resilience.
For further insights into security practices for Java applications, refer to OWASP, the Open Web Application Security Project, and Java Secure Coding Guidelines from the MITRE Corporation.
Remember, safeguarding JSF applications on JBoss AS 7.x is an ongoing endeavor that demands vigilance and adaptability in the face of evolving cybersecurity challenges.
Checkout our other articles