Sync Issues in 3 Java IDEs: Solving Common Method Glitches
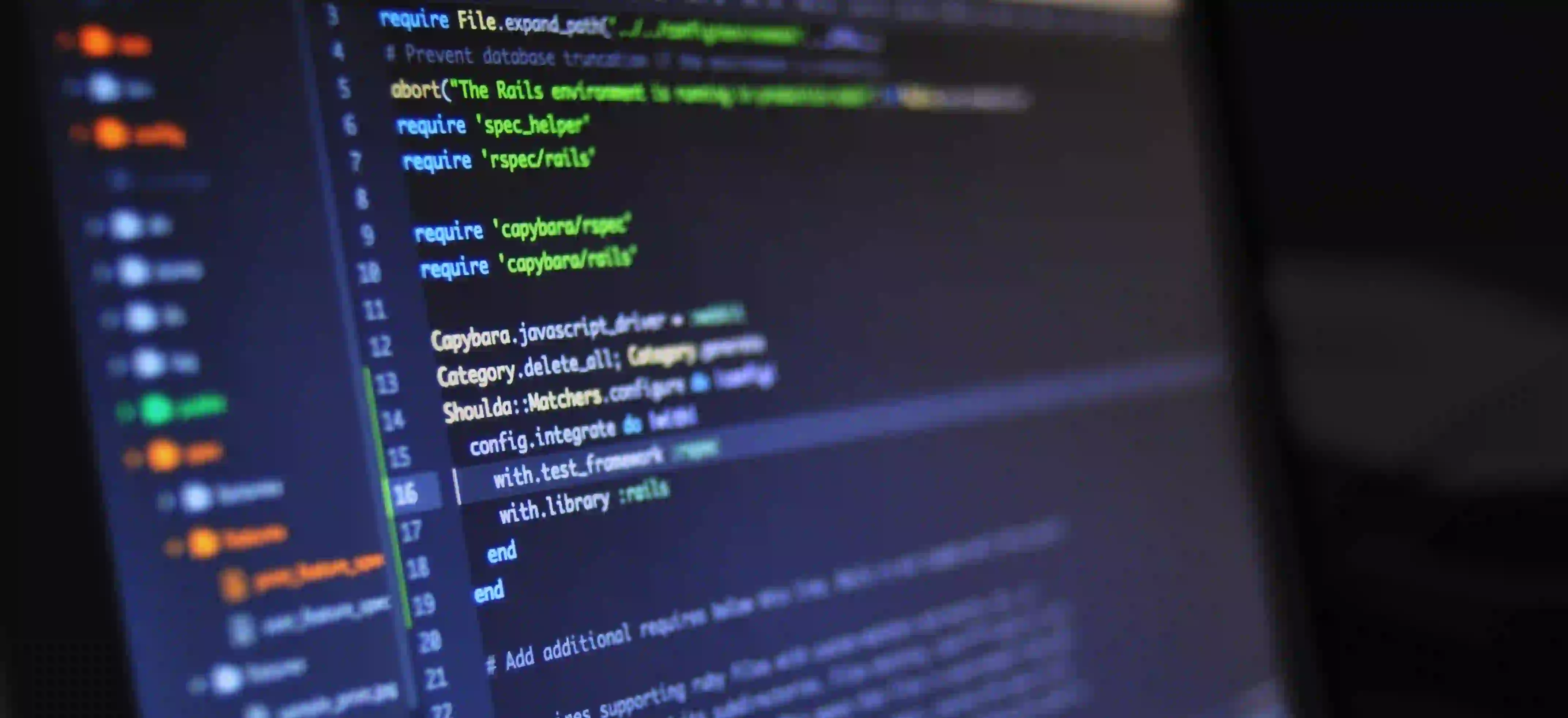
Diagnosing Method Glitches in Java IDEs: A Deep Dive into Sync Issues
Java Integrated Development Environments (IDEs) are potent tools that streamline the software development process. However, sometimes, we encounter glitches related to method synchronization. In this post, we will explore common sync issues in three popular Java IDEs - IntelliJ IDEA, Eclipse, and NetBeans - and delve into effective strategies for resolving these problems.
Understanding Method Synchronization
Before delving into the sync issues, let's understand the concept of method synchronization in Java. Method synchronization ensures that only one thread can execute a synchronized method at a time. This is vital for preventing concurrency issues in multi-threaded applications. However, sometimes IDEs can misinterpret or mishandle method synchronization, leading to unexpected behavior.
Sync Issues in IntelliJ IDEA
IntelliJ IDEA, a highly extensible IDE, is widely used for Java development. In some cases, users may encounter sync issues related to method locking and unlocking. One common scenario is when the IDE fails to recognize the correct lock on a method, leading to potential concurrency problems.
Consider the following code snippet:
public class SynchronizationExample {
private final Object lock = new Object();
public void synchronizedMethod() {
synchronized (lock) {
// Critical section
}
}
}
In IntelliJ IDEA, the IDE may fail to identify that lock
is used for synchronization, which could lead to potential race conditions. To address this, we can utilize the '@NotNull' annotation from the IntelliJ IDEA library to explicitly specify the lock object, ensuring that the IDE accurately recognizes the synchronization:
import org.jetbrains.annotations.NotNull;
public class SynchronizationExample {
private final Object lock = new Object();
public void synchronizedMethod() {
synchronized (lock) {
// Critical section
}
}
}
By incorporating the '@NotNull' annotation, we provide IntelliJ IDEA with explicit information about the synchronization lock, mitigating potential sync issues.
Tackling Sync Problems in Eclipse
Eclipse, another prominent Java IDE, is not immune to method synchronization glitches. A common issue in Eclipse involves the incorrect handling of synchronized methods within anonymous inner classes. Consider the following code snippet:
public class SynchronizationExample {
public void performAction() {
synchronized (this) {
Runnable runnable = new Runnable() {
@Override
public void run() {
// Critical section
}
};
new Thread(runnable).start();
}
}
}
In this scenario, Eclipse may not interpret the synchronization correctly, potentially leading to concurrency issues. To resolve this, we can explicitly specify the lock object by creating a separate variable:
public class SynchronizationExample {
private final Object lock = new Object();
public void performAction() {
synchronized (lock) {
Runnable runnable = new Runnable() {
@Override
public void run() {
// Critical section
}
};
new Thread(runnable).start();
}
}
}
By creating a separate lock object and synchronizing on it, we ensure that Eclipse accurately recognizes the synchronization, thus mitigating potential sync problems.
Overcoming Sync Hurdles in NetBeans
NetBeans, known for its simplicity and ease of use, may also encounter method synchronization challenges. One prevalent issue in NetBeans is related to the incorrect identification of synchronized methods when dealing with lambda expressions. Consider the following code snippet:
public class SynchronizationExample {
public void performAction() {
synchronized (this) {
new Thread(() -> {
// Critical section
}).start();
}
}
}
In this example, NetBeans may not interpret the synchronization correctly due to the lambda expression, potentially resulting in concurrency issues. To address this, we can utilize an explicit lock object as follows:
public class SynchronizationExample {
private final Object lock = new Object();
public void performAction() {
synchronized (lock) {
new Thread(() -> {
// Critical section
}).start();
}
}
}
By introducing an explicit lock object, we ensure that NetBeans accurately recognizes the synchronization, thereby overcoming potential sync hurdles.
Wrapping Up
In conclusion, method synchronization glitches can pose significant challenges during Java development in popular IDEs such as IntelliJ IDEA, Eclipse, and NetBeans. By understanding these sync issues and adopting targeted strategies, developers can effectively mitigate potential concurrency problems. Whether it involves leveraging annotations, restructuring code, or introducing explicit lock objects, addressing method synchronization glitches in Java IDEs is essential for ensuring the robustness and reliability of multi-threaded applications.
By applying the strategies outlined in this post, developers can effectively diagnose and resolve method synchronization issues, fostering a smoother and more reliable Java development experience.
For further reading on method synchronization and Java best practices, I recommend checking out the official Java documentation on Concurrency in Java. Additionally, you can explore advanced synchronization techniques in the book Java Concurrency in Practice.
Happy coding and may your methods synchronize flawlessly!