Mastering Correlation IDs in Spring Boot for Seamless Tracing
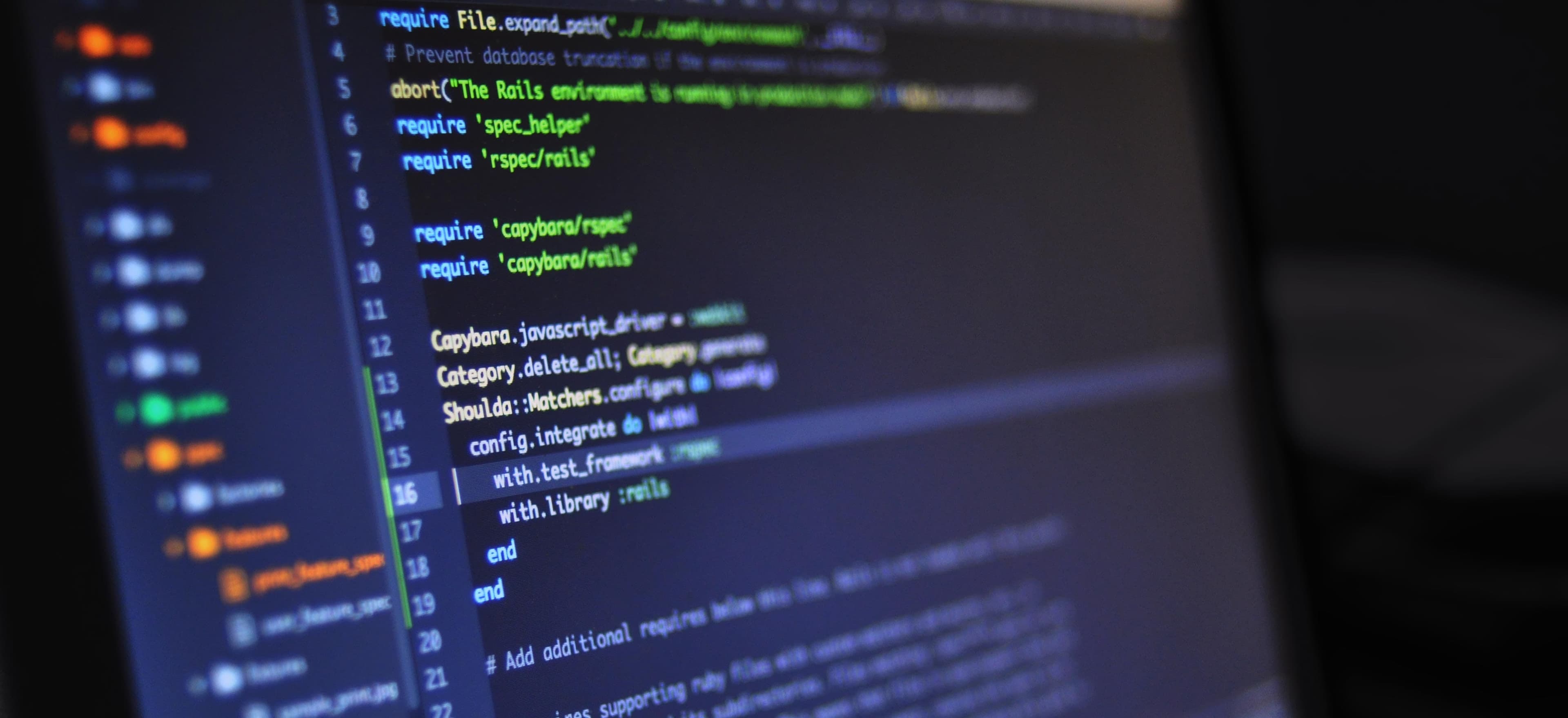
- Published on
Mastering Correlation IDs in Spring Boot for Seamless Tracing
In a microservices architecture, tracing and monitoring are essential for maintaining the health and performance of the system. When a single request fans out to multiple microservices, it becomes crucial to trace the flow of the request across these services. This is where correlation IDs come into play.
Correlation IDs are unique identifiers that are attached to every incoming request and passed along to all the subsequent services that get invoked. This allows for the aggregation of logs and traces across different services, providing a unified view of the entire request flow. In this article, we will explore how to implement correlation IDs in a Spring Boot application for seamless tracing.
Understanding the Need for Correlation IDs
Consider a scenario where a client makes a request to Service A, which in turn needs to interact with Service B and Service C to fulfill the request. Without correlation IDs, tracking the flow of the request across these services can become extremely challenging. Each service may generate its own logs and traces, making it cumbersome to correlate and analyze them collectively.
By incorporating correlation IDs, we can assign a unique identifier to each incoming request and propagate it across all the downstream services. This allows for easy tracking and aggregation of logs related to a specific request, simplifying the troubleshooting and debugging process.
Implementing Correlation IDs in Spring Boot
Using MDC (Mapped Diagnostic Context) for Correlation IDs
In a Spring Boot application, we can leverage the Mapped Diagnostic Context (MDC) provided by the SLF4J framework to manage correlation IDs. MDC allows us to enrich log messages with contextual information, which is particularly useful for tracing the flow of requests.
Let's consider an example of how we can implement correlation IDs using MDC in a Spring Boot application.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.MDC;
import org.springframework.web.bind.annotation.*;
@RestController
public class UserController {
private static final Logger logger = LoggerFactory.getLogger(UserController.class);
@GetMapping("/users/{id}")
public String getUserById(@PathVariable String id) {
String correlationId = generateCorrelationId();
MDC.put("Correlation-ID", correlationId);
logger.info("Received request to fetch user with ID: {}", id);
// Invoke service to fetch user details
MDC.clear();
return "User details for ID: " + id;
}
private String generateCorrelationId() {
// Logic to generate a unique correlation ID
return "CID-" + UUID.randomUUID().toString();
}
}
In this example, we have a UserController
with a getUserById
method. Upon receiving a request, we generate a unique correlation ID and store it in the MDC. This ensures that every log statement emitted during the processing of this request includes the correlation ID.
Propagating Correlation IDs to Downstream Services
Once we have set up the correlation ID in the MDC, we need to ensure that it gets propagated to any downstream services that are invoked as part of the request processing. This typically involves including the correlation ID in outgoing HTTP headers or message payloads.
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
public class UserClient {
private RestTemplate restTemplate;
// Constructor and other methods
public String getUserDetails(String userId) {
HttpHeaders headers = new HttpHeaders();
headers.set("Correlation-ID", MDC.get("Correlation-ID"));
// Include other necessary headers
// Make HTTP call to fetch user details from downstream service
}
}
In the UserClient
class, we set the "Correlation-ID" header in the outgoing HTTP request using the value retrieved from the MDC. This ensures that the downstream service receives the correlation ID and can also incorporate it into its logs and traces.
Centralized Logging and Tracing with Correlation IDs
With correlation IDs in place, we can now aggregate and correlate logs and traces across all the services involved in processing a request. Tools like Zipkin, Jaeger, or Elasticsearch can be used to visualize and analyze the request flow based on the correlation IDs.
By querying logs and traces using the correlation ID, it becomes much easier to track the journey of a request through various microservices, identify performance bottlenecks, and troubleshoot errors.
Best Practices for Correlation IDs
- Generate Unique IDs: Ensure that the correlation IDs generated are globally unique. UUIDs or Snowflake IDs are commonly used for this purpose.
- Propagate Consistently: Make sure the correlation IDs are consistently propagated to all downstream services, whether it's through HTTP headers, message headers, or any other communication mechanism.
- Clearing Context: Always remember to clear the correlation ID from the context once the request processing is complete, to avoid any accidental leakage of context to unrelated requests.
Final Considerations
In a microservices environment, implementing correlation IDs is a best practice for seamless tracing of requests across multiple services. By leveraging tools like MDC in conjunction with centralized logging and tracing systems, we can gain valuable insights into the flow of requests and effectively troubleshoot issues.
With this understanding of correlation IDs and their implementation in Spring Boot, you can now ensure that your microservices ecosystem is well-equipped for seamless tracing and monitoring. Happy tracing!
Checkout our other articles