Product vs. Purpose: Crafting Strategy Beyond Tactics
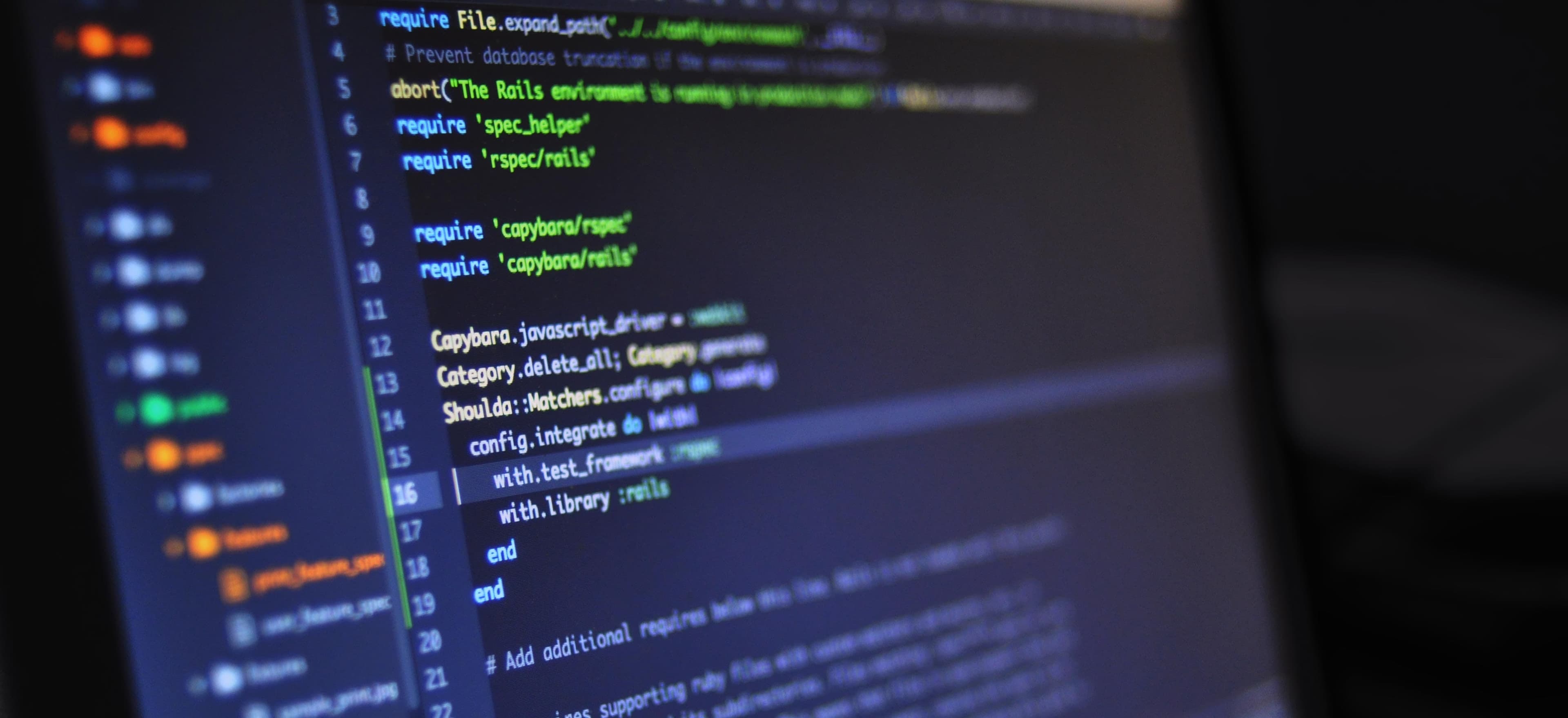
- Published on
Product vs. Purpose: Crafting Strategy Beyond Tactics
In the world of Java development, it's easy to get caught up in the details of writing code and forget the bigger picture. While it's important to have a solid understanding of the technical aspects of Java, it's equally crucial to approach software development with a strategic mindset. This involves looking beyond the immediate tasks at hand and considering the broader purpose and impact of the software being built.
The Role of Strategy in Java Development
When it comes to Java development, having a strategic approach means understanding the underlying purpose of the software being built and aligning every technical decision with that purpose. This requires developers to think beyond writing efficient code and delve into the larger goals of the project.
Understanding the Product
Before writing a single line of code, it's essential to have a clear understanding of the product being developed. What is its purpose? Who are the end users? What problems does it solve? By answering these questions, developers can gain valuable insight into the overall objectives of the project.
In Java development, having a deep understanding of the product helps in designing the software architecture in a way that aligns with the product's purpose. For instance, if the product is a web application that needs to handle a large number of concurrent user requests, the choice of framework and design patterns can significantly impact its performance and scalability.
Aligning Technical Decisions with Purpose
Once the purpose of the product is understood, every technical decision should be evaluated based on how well it serves that purpose. This means that developers should resist the temptation to adopt the latest technologies or implement complex solutions simply for the sake of it. Instead, they should focus on choosing tools and techniques that directly contribute to the product's purpose.
For example, when deciding between different Java frameworks, such as Spring and Micronaut, the choice should be made based on which framework best aligns with the requirements and purpose of the product. This strategic approach ensures that technical decisions are not made in isolation but are instead driven by the overarching purpose of the software.
Balancing Tactical Implementation with Strategic Vision
While the strategic aspect of Java development is crucial, it's equally important to balance it with effective tactical implementation. This involves writing clean, maintainable code that aligns with the strategic vision of the project.
Writing Clean and Maintainable Code
Clean code is essential for the long-term success of any software project. It not only makes the codebase easier to understand and maintain but also aligns with the strategic goal of building a reliable and efficient product. In Java development, adhering to coding best practices and design principles such as SOLID principles, DRY (Don't Repeat Yourself), and KISS (Keep It Simple, Stupid) not only improves the quality of the code but also supports the strategic purpose of the software.
// Example of adhering to SOLID principles
public interface Shape {
double calculateArea();
}
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
In the above example, the Shape
interface adheres to the Single Responsibility Principle (SRP) by defining a single responsibility, i.e., calculating the area. The Circle
class then implements this interface to perform the specific task of calculating the area of a circle.
Embracing Agile Methodologies
Agile methodologies such as Scrum or Kanban provide a tactical framework for iterative and incremental development. By breaking down the strategic vision into smaller, manageable tasks, Agile methodologies allow Java developers to focus on delivering value in small increments while continuously aligning their work with the strategic purpose of the software. This ensures that the development process remains adaptable to changing requirements without losing sight of the bigger strategic goals.
Final Considerations
In the world of Java development, it's crucial to strike a balance between tactical implementation and strategic vision. By understanding the product's purpose and aligning technical decisions with that purpose, Java developers can ensure that their code serves a larger strategic goal. Additionally, by embracing clean coding practices and Agile methodologies, developers can effectively translate the strategic vision into a reliable and maintainable software product.
Ultimately, by crafting a strategy beyond tactics, Java developers can elevate their role from mere code writers to strategic contributors, actively shaping the success of the software they build.
Remember, always keep the purpose in mind, and let it drive your every technical decision.