Shrink Your App's Memory Footprint with Flyweight Pattern!
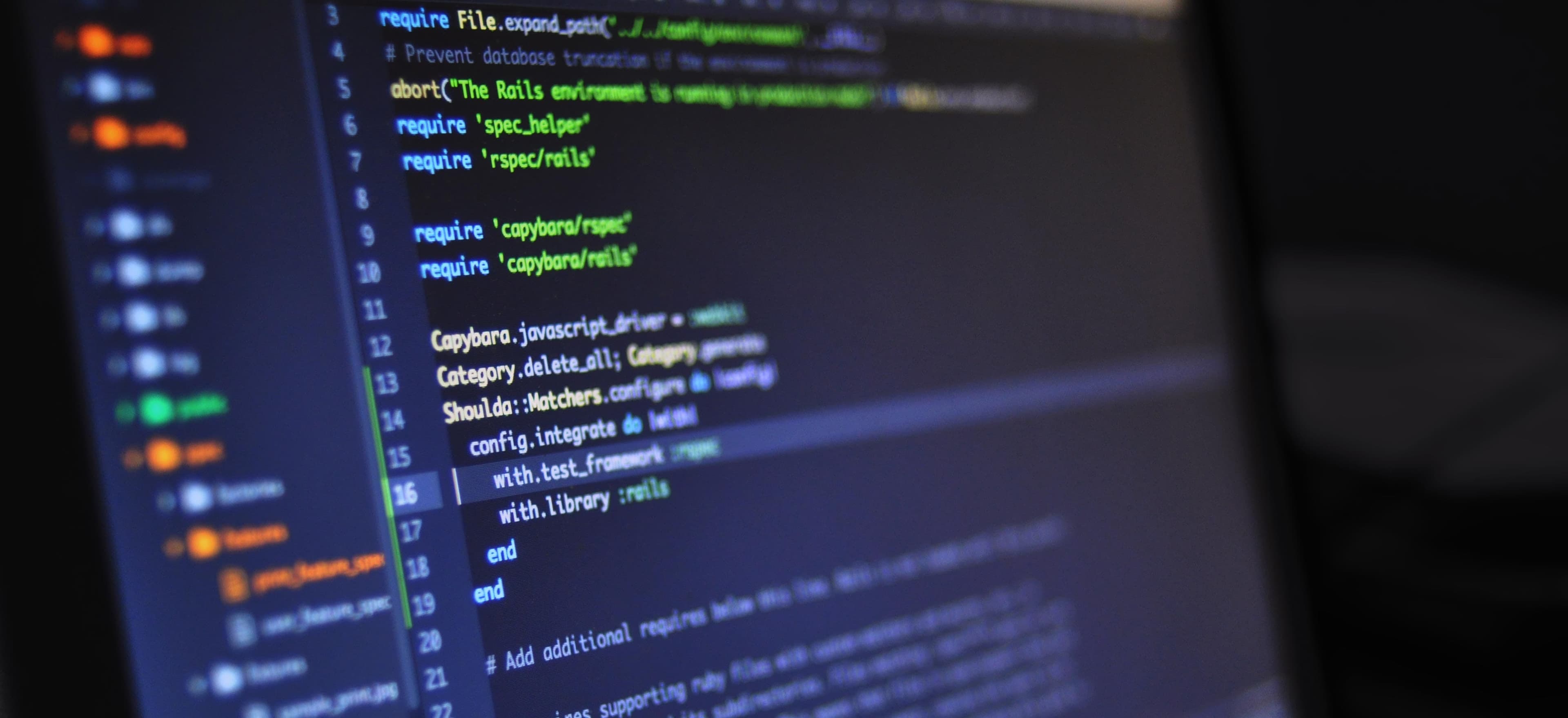
- Published on
Understanding the Flyweight Pattern in Java
In today's digital era, creating memory-efficient and high-performing applications is crucial for delivering a seamless user experience. As a Java developer, you might encounter scenarios where your application's memory footprint becomes a concern.
This is where the Flyweight pattern comes into play. The Flyweight pattern is a structural design pattern that focuses on minimizing memory usage by sharing data efficiently. By doing so, it reduces the overall memory footprint of an application, making it an excellent choice for optimizing performance-critical applications.
What is the Flyweight Pattern?
The Flyweight pattern is based on the concept of reusing objects to conserve memory. It achieves this by splitting the object properties into intrinsic and extrinsic state. The intrinsic state is shared and immutable, allowing multiple objects to refer to the same data. On the other hand, the extrinsic state is unique to each object and is managed externally.
Implementing the Flyweight Pattern
Let's explore how the Flyweight pattern can be implemented in Java to reduce memory overhead in applications.
Step 1: Identify the Intrinsic and Extrinsic State
When applying the Flyweight pattern, the first step involves identifying the intrinsic and extrinsic state of the objects. The intrinsic state represents shared data that can be reused, while the extrinsic state contains context-specific data unique to each object. By segregating the state in this manner, we can effectively reduce memory consumption.
Step 2: Create the Flyweight Interface
Next, we define a Flyweight interface that declares methods to manipulate the extrinsic state. This interface acts as the blueprint for the concrete flyweight objects.
public interface Flyweight {
void operation(String extrinsicState);
}
Step 3: Develop Concrete Flyweight Implementations
Concrete flyweight implementations encapsulate the intrinsic state of the object and provide the implementation for the Flyweight interface. These objects are shared and reused across multiple contexts, leading to memory savings.
public class ConcreteFlyweight implements Flyweight {
private String intrinsicState;
public ConcreteFlyweight(String intrinsicState) {
this.intrinsicState = intrinsicState;
}
@Override
public void operation(String extrinsicState) {
System.out.println("Intrinsic State: " + intrinsicState + ", Extrinsic State: " + extrinsicState);
}
}
Step 4: Utilize a Flyweight Factory
To manage the creation and retrieval of flyweight objects, a flyweight factory is employed. This factory ensures that flyweight objects are shared and reused efficiently.
import java.util.HashMap;
import java.util.Map;
public class FlyweightFactory {
private static final Map<String, Flyweight> flyweights = new HashMap<>();
public Flyweight getFlyweight(String key) {
if (!flyweights.containsKey(key)) {
flyweights.put(key, new ConcreteFlyweight(key));
}
return flyweights.get(key);
}
}
Step 5: Client Utilization
In the client code, we can now utilize the flyweight objects by requesting them from the flyweight factory. The extrinsic state unique to each context is passed to the flyweight objects, allowing them to fulfill their purpose without duplicating intrinsic data.
public class Client {
public static void main(String[] args) {
FlyweightFactory factory = new FlyweightFactory();
Flyweight flyweight1 = factory.getFlyweight("shared");
flyweight1.operation("context1");
Flyweight flyweight2 = factory.getFlyweight("shared");
flyweight2.operation("context2");
}
}
By following these steps and principles, the Flyweight pattern enables the efficient utilization of memory resources and optimizes the overall performance of Java applications.
Benefits of the Flyweight Pattern
- Memory Efficiency: By sharing intrinsic state, the Flyweight pattern significantly reduces memory consumption.
- Performance Optimization: With reduced memory overhead, the application's performance is enhanced, leading to improved responsiveness.
- Simplified Maintenance: The pattern promotes code reusability and maintainability, contributing to a more organized codebase.
My Closing Thoughts on the Matter
In the realm of Java development, understanding and leveraging design patterns such as the Flyweight pattern is instrumental in crafting high-quality, efficient applications. By adopting the Flyweight pattern, you can effectively shrink your application's memory footprint and elevate its performance, ultimately enriching the user experience.
Incorporating design patterns into your development arsenal not only enhances your technical acumen but also empowers you to tackle real-world challenges with finesse. Embrace the Flyweight pattern and embark on a journey towards crafting memory-conscious, high-performing Java applications.
So, why wait? Dive into the world of design patterns and propel your Java development expertise to new heights.
To delve deeper into the Flyweight pattern and its applications, check out the following resources:
- Design Patterns: Elements of Reusable Object-Oriented Software
- Java Design Patterns: A Hands-On Experience with Real-World Examples
Start optimizing your app's memory footprint today!