Mastering Chain of Responsibility with Spring Autowired Lists
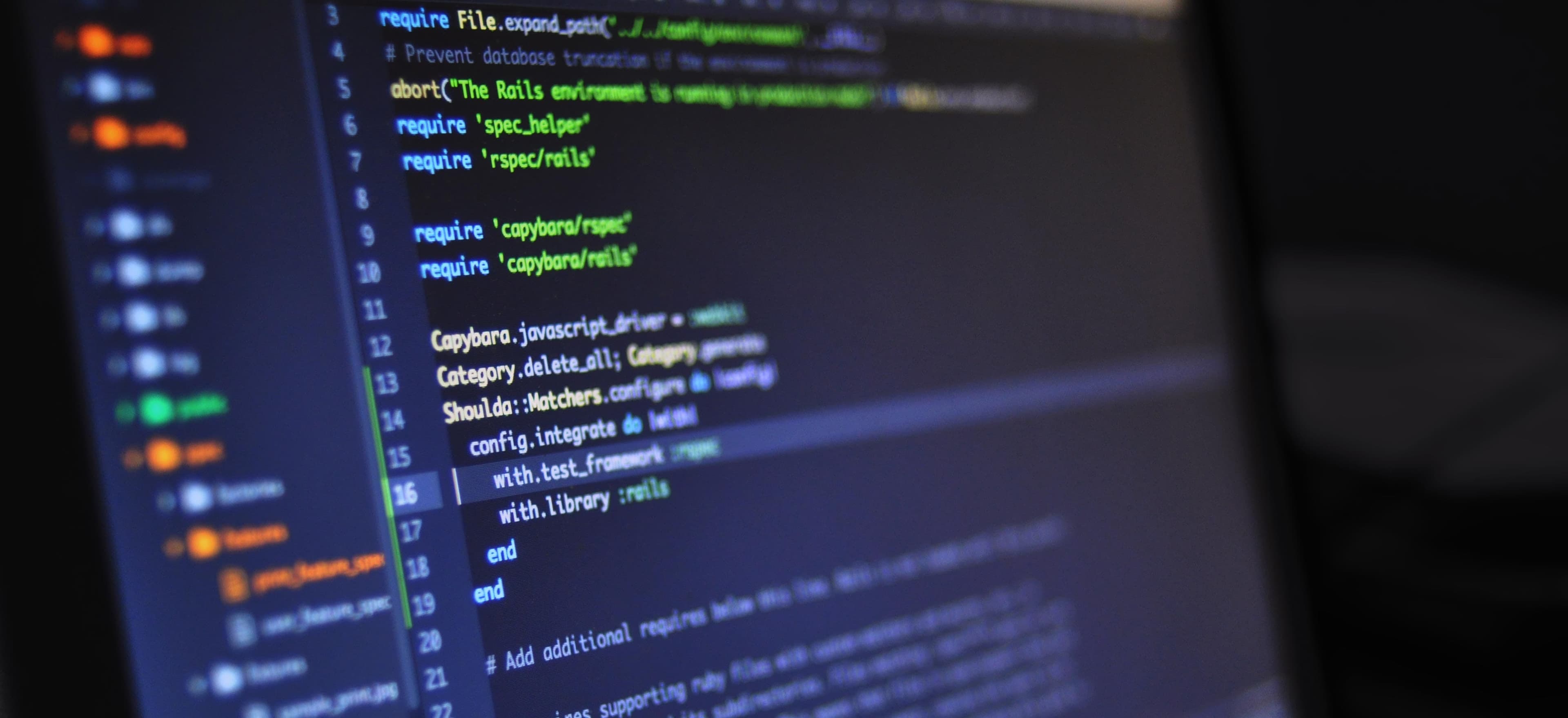
- Published on
Mastering Chain of Responsibility with Spring Autowired Lists
The Chain of Responsibility design pattern is a powerful tool in a developer's arsenal when it comes to handling requests in a flexible and decoupled manner. When combined with Spring Autowired Lists, it becomes even more potent. In this post, we'll explore how to master the Chain of Responsibility pattern using Spring Autowired Lists in Java.
Understanding the Chain of Responsibility Pattern
The Chain of Responsibility pattern is a behavioral design pattern that allows an object to pass a request along a chain of handlers, with each handler deciding whether to process the request or pass it on to the next handler in the chain. This pattern promotes loose coupling and flexibility by allowing handlers to be added or removed without affecting the client that initiates the request.
Implementing the Chain of Responsibility Pattern in Java
Let's start by creating the foundation for our Chain of Responsibility implementation. We'll define an interface for the handler and a base abstract class that implements the handler interface.
// Handler interface
public interface Handler {
void handleRequest(Request request);
}
// Abstract base handler
public abstract class AbstractHandler implements Handler {
protected Handler nextHandler;
public void setNextHandler(Handler nextHandler) {
this.nextHandler = nextHandler;
}
}
In the above code, we've defined the Handler
interface with a method handleRequest
to process the request. We've also created an abstract class AbstractHandler
that implements the Handler
interface and provides a mechanism to set the next handler in the chain.
Next, let's create concrete handler implementations.
// Concrete handler 1
@Component
public class ConcreteHandler1 extends AbstractHandler {
@Override
public void handleRequest(Request request) {
// Process the request or pass it to the next handler
if (/* condition to handle request */) {
// handle the request
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
// Concrete handler 2
@Component
public class ConcreteHandler2 extends AbstractHandler {
@Override
public void handleRequest(Request request) {
// Process the request or pass it to the next handler
if (/* condition to handle request */) {
// handle the request
} else if (nextHandler != null) {
nextHandler.handleRequest(request);
}
}
}
In the above code, we've created two concrete handler implementations ConcreteHandler1
and ConcreteHandler2
by extending the AbstractHandler
class. We've also annotated these classes with @Component
to make them Spring-managed beans.
Leveraging Spring Autowired Lists
Now that we have our handler implementations, let's leverage Spring Autowired Lists to automatically inject all the handler beans into a list.
// Chain executor
@Component
public class ChainExecutor {
@Autowired
private List<Handler> handlers;
public void execute(Request request) {
for (Handler handler : handlers) {
handler.handleRequest(request);
}
}
}
In the above code, we've created a ChainExecutor
class that is annotated with @Component
to make it a Spring-managed bean. The ChainExecutor
class has a field handlers
annotated with @Autowired
, which will be automatically populated with all the beans of type Handler
by Spring.
Putting it All Together
Now, let's see how we can put it all together and invoke the chain of responsibility.
// Client code
@Component
public class Client {
@Autowired
private ChainExecutor chainExecutor;
public void sendRequest(Request request) {
chainExecutor.execute(request);
}
}
In the above code, we've created a Client
class that is annotated with @Component
to make it a Spring-managed bean. The Client
class has a field chainExecutor
annotated with @Autowired
, which will be automatically populated with the ChainExecutor
bean by Spring. The sendRequest
method initiates the chain of responsibility by calling the execute
method on the chainExecutor
.
Advantages of Using Spring Autowired Lists
By leveraging Spring Autowired Lists, we've achieved a seamless integration of the Chain of Responsibility pattern with Spring's inversion of control. This approach offers several advantages:
-
Flexible Configuration: Handlers can be added or removed from the chain simply by creating or removing the respective Spring-managed beans. There's no need to modify the client code, thus promoting flexibility and ease of maintenance.
-
Loose Coupling: The client code is decoupled from the specific handlers in the chain, as it only interacts with the
ChainExecutor
and does not need to be aware of the individual handlers.
Lessons Learned
In this post, we've delved into the powerful combination of the Chain of Responsibility pattern and Spring Autowired Lists in Java. We've learned how to implement the Chain of Responsibility pattern using Spring-managed beans and leverage Spring Autowired Lists to dynamically inject the handler beans into a list. This approach not only simplifies the configuration and management of the chain but also promotes loose coupling and flexibility in handling requests.
By mastering the Chain of Responsibility with Spring Autowired Lists, you're equipped to build robust and maintainable systems that gracefully handle requests in a decoupled and flexible manner.
Start harnessing the power of Chain of Responsibility with Spring Autowired Lists today for a more efficient and adaptable request handling in your Java applications!
For more in-depth insights into Spring Autowired Lists, check out the official Spring documentation and Baeldung's guide.
Checkout our other articles