Troubleshooting Spring JMS Configuration in Spring Boot
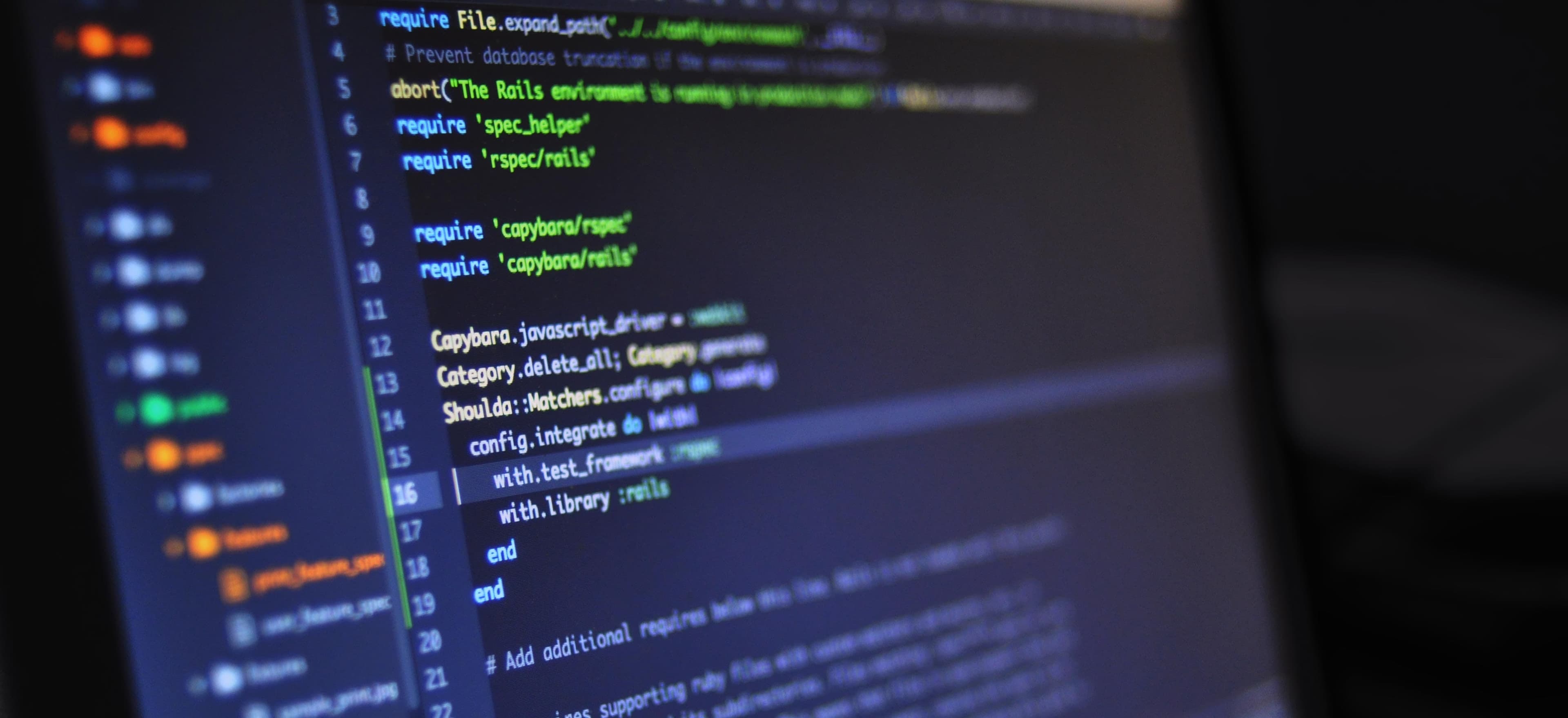
- Published on
Troubleshooting Spring JMS Configuration in Spring Boot
When working with Spring Boot applications that involve messaging, it's common to utilize the Java Message Service (JMS) to handle asynchronous communication between distributed systems. Spring provides seamless integration with JMS through its spring-jms
module, allowing for the creation of message-driven Pojo-based components. However, configuring and troubleshooting Spring JMS in a Spring Boot application can sometimes pose challenges. In this article, we'll explore common issues encountered when setting up Spring JMS in Spring Boot and how to troubleshoot them effectively.
Checking Dependencies
A crucial step in troubleshooting any issue in a Spring Boot application is to ensure that the necessary dependencies are included in the project. When working with Spring JMS, make sure the following dependencies are present in your pom.xml
(for Maven) or build.gradle
(for Gradle) file:
Maven dependencies
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jms</artifactId>
</dependency>
Gradle dependencies
implementation 'org.springframework.boot:spring-boot-starter-activemq'
implementation 'org.springframework.boot:spring-boot-starter-jms'
If any of these dependencies are missing, it can lead to various runtime issues when working with Spring JMS.
Connection Factory Configuration
An essential part of setting up Spring JMS is configuring the JMS connection factory. The connection factory is responsible for creating connections to the messaging broker. In a typical Spring Boot application, you can configure the connection factory properties in the application.properties
or application.yml
file. Here's an example configuration for Apache ActiveMQ:
spring.activemq.broker-url=tcp://localhost:61616
spring.activemq.user=admin
spring.activemq.password=secret
When troubleshooting issues related to the connection factory, ensure that the connection parameters such as broker URL, username, and password are correctly configured. Additionally, verify that the messaging broker is running and accessible from the application.
Destination Configuration
In a JMS environment, a destination represents the target location for sending and receiving messages. Destinations can be of two types: queues (point-to-point messaging) and topics (publish-subscribe messaging). When encountering issues with message consumption or production, it's essential to verify the correctness of destination configuration.
In Spring JMS, you can define destinations using JmsTemplate
or @JmsListener
annotations. Here's an example of configuring a queue destination using JmsTemplate
:
@Configuration
public class JmsConfig {
@Bean
public Queue queue() {
return new ActiveMQQueue("myQueue");
}
}
When debugging issues related to destinations, check that the destination names match between the producer and the consumer. Misconfigured or non-existent destinations can result in message delivery failures.
Message Conversion
Another aspect to consider when troubleshooting Spring JMS is message conversion. By default, Spring uses a SimpleMessageConverter
for converting between Java objects and JMS messages. However, you may encounter situations where custom message conversion logic is required to handle specific message types.
In such cases, you can create custom message converters by implementing the MessageConverter
interface. For example:
public class CustomMessageConverter implements MessageConverter {
@Override
public Message toMessage(Object object, Session session) throws JMSException {
// Custom conversion logic from object to JMS message
}
@Override
public Object fromMessage(Message message) throws JMSException {
// Custom conversion logic from JMS message to object
}
}
When experiencing issues with message conversion, ensure that the message payload and the Java object being sent or received are compatible. Verify that the message converter is properly configured and handling the conversion logic as expected.
Transaction Management
Transactional behavior is crucial in messaging systems to ensure message delivery and processing reliability. When working with Spring JMS, it's essential to understand and effectively troubleshoot transaction management.
In a Spring Boot application, you can annotate the message listener method with @Transactional
to enable transactional behavior. For example:
@Component
public class MessageListenerService {
@JmsListener(destination = "myQueue")
@Transactional
public void onMessage(Message message) {
// Process the message within a transaction
}
}
When encountering issues with transaction management, verify that the transactional behavior is appropriately applied to message-consuming components. Additionally, ensure that the transaction boundaries are correctly set to encompass the message processing logic.
Error Handling and Logging
Effective error handling and logging are indispensable for troubleshooting issues in Spring JMS. When dealing with message reception or processing failures, it's essential to log pertinent information and handle exceptions gracefully.
You can implement error handling and logging strategies using Spring's exception handling mechanisms, such as @JmsListener
's errorHandler
attribute or utilizing a logging framework like Logback or Log4j.
Ensure that appropriate error handling mechanisms are in place to capture and handle exceptions that may occur during message processing. Logging detailed information such as message contents, processing stages, and exception stack traces can aid in diagnosing and resolving issues efficiently.
Testing with Embedded Brokers
During troubleshooting, employing an embedded message broker for testing can be advantageous. Embedded brokers, such as the Apache ActiveMQ Artemis or ActiveMQ broker, can facilitate local testing without the need for an external messaging server.
By including the embedded broker dependencies and configurations in the test scope, you can simulate messaging interactions within the test environment. This approach allows for comprehensive testing and debugging of Spring JMS components in isolation.
Key Takeaways
Troubleshooting Spring JMS configuration in Spring Boot applications involves addressing various aspects such as dependencies, connection factory setup, destination configuration, message conversion, transaction management, error handling, and testing strategies. By systematically examining these components and their interactions, you can effectively diagnose and resolve issues encountered when working with Spring JMS.
Remember, thorough familiarity with the messaging concepts, coupled with vigilant testing and proactive error handling, is key to ensuring robust and reliable messaging behavior in Spring Boot applications.
In conclusion, troubleshooting Spring JMS in Spring Boot necessitates a comprehensive understanding of the underlying messaging infrastructure, diligent configuration management, and meticulous attention to error handling and testing practices.