Secure or Skip? Navigating SSL Verification in Spring
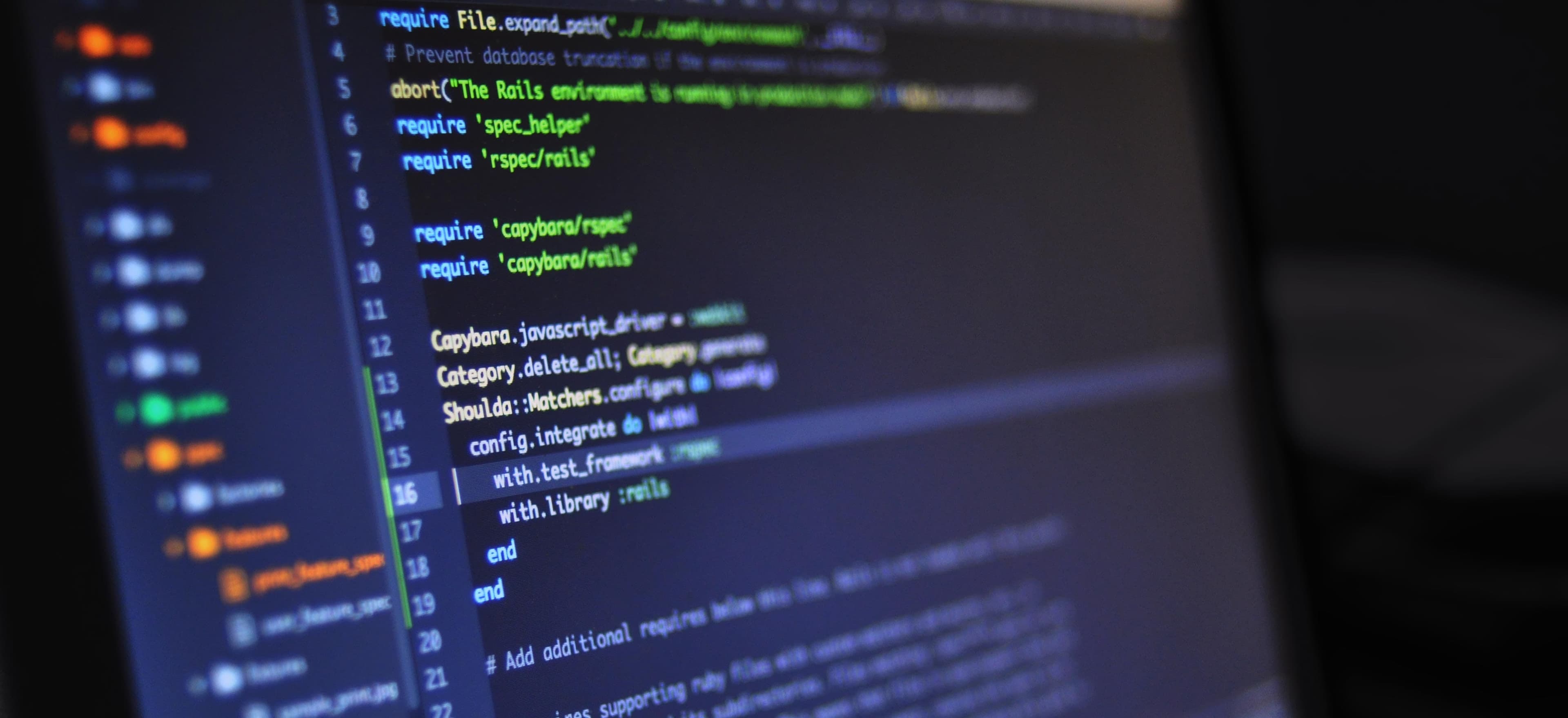
- Published on
Secure or Skip? Navigating SSL Verification in Spring
When it comes to developing secure applications, SSL (Secure Sockets Layer) plays a vital role in ensuring data confidentiality and integrity between the client and server. In the Java ecosystem, Spring is a widely-used framework for building enterprise-grade applications. In this article, we will explore the significance of SSL verification and how to handle it effectively in a Spring application.
Understanding SSL Verification
SSL verification is the process of ensuring that the server's SSL certificate is valid and trusted. When a client (such as a Spring application) communicates with a server over HTTPS, it needs to verify the server's SSL certificate to establish a secure connection.
If SSL verification is not properly handled, it can lead to security vulnerabilities such as man-in-the-middle attacks, where a malicious actor intercepts and potentially alters the communication between the client and server.
The Importance of SSL Verification in Spring Applications
In a Spring application, SSL verification is crucial for maintaining a secure channel for data exchange. By default, Spring Framework performs SSL verification to prevent unauthorized access and protect sensitive information.
However, there are scenarios where developers might need to adjust the SSL verification behavior based on specific requirements, such as dealing with self-signed certificates in a development environment or integrating with servers using custom or internal Certificate Authorities (CAs).
Managing SSL Verification in Spring
Default SSL Verification Behavior in Spring RestTemplate
In a typical Spring application, the RestTemplate
class is commonly used for making HTTP requests. When using RestTemplate
to communicate with a server over HTTPS, SSL verification is enabled by default. This means that the server's SSL certificate will be validated against the system's trusted CAs.
Handling Self-Signed Certificates
In some development or testing environments, using self-signed SSL certificates is common. When communicating with a server that uses a self-signed certificate, the default behavior of SSL verification in Spring will cause the connection to fail, as the certificate is not signed by a trusted CA.
To handle self-signed certificates in Spring, one approach is to customize the SSL verification behavior, either by trusting all certificates (insecure) or by explicitly configuring the RestTemplate
to trust specific self-signed certificates.
Trusting All Certificates (Insecure Approach)
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.HttpsURLConnection;
...
SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory();
requestFactory.setTrustAll(true);
RestTemplate restTemplate = new RestTemplate(requestFactory);
The above code snippet demonstrates an insecure approach by trusting all SSL certificates, including self-signed ones. However, it's important to note that this approach compromises the security of the communication, and it's not recommended for production use.
Trusting Specific Self-Signed Certificates
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.HttpsURLConnection;
...
SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory();
requestFactory.setHostnameVerifier((hostname, session) -> {
// Customize hostname verification if needed
return true;
});
requestFactory.setTrustManagers( /* custom trust managers for specific self-signed certificates */ );
RestTemplate restTemplate = new RestTemplate(requestFactory);
In the above code snippet, the RestTemplate
is configured to trust specific self-signed certificates by providing custom trust managers. This approach allows developers to explicitly define the trust logic for specific certificates while maintaining a higher level of security compared to the "trust all" approach.
Disabling SSL Verification
While it's generally not recommended for secure communication, there may be scenarios where developers need to disable SSL verification entirely, such as when integrating with legacy systems that do not have valid SSL certificates.
import org.springframework.http.client.SimpleClientHttpRequestFactory;
import org.springframework.web.client.RestTemplate;
import javax.net.ssl.HttpsURLConnection;
...
SimpleClientHttpRequestFactory requestFactory = new SimpleClientHttpRequestFactory();
requestFactory.setTrustAll(true); // Insecure approach to disable SSL verification
RestTemplate restTemplate = new RestTemplate(requestFactory);
By setting trustAll
to true, the SSL verification is effectively disabled. However, it's crucial to consider the security implications and use this approach judiciously, as it exposes the communication to potential security risks.
Wrapping Up
SSL verification is a critical aspect of secure communication in Spring applications. While the default behavior in Spring ensures secure connections, developers may need to customize SSL verification to accommodate specific use cases, such as dealing with self-signed certificates or legacy systems with invalid SSL certificates.
It's essential to carefully evaluate the risk and impact of modifying SSL verification behavior, and choose the most appropriate approach based on the security requirements of the application.
By understanding and effectively managing SSL verification in Spring applications, developers can uphold the integrity and confidentiality of data exchanged over secure channels, contributing to the overall security posture of their applications.
For more in-depth information on SSL verification in Spring, refer to the official Spring documentation.
Remember, security should never be compromised!