Solving JDBC Realm Authentication in GlassFish & PrimeFaces
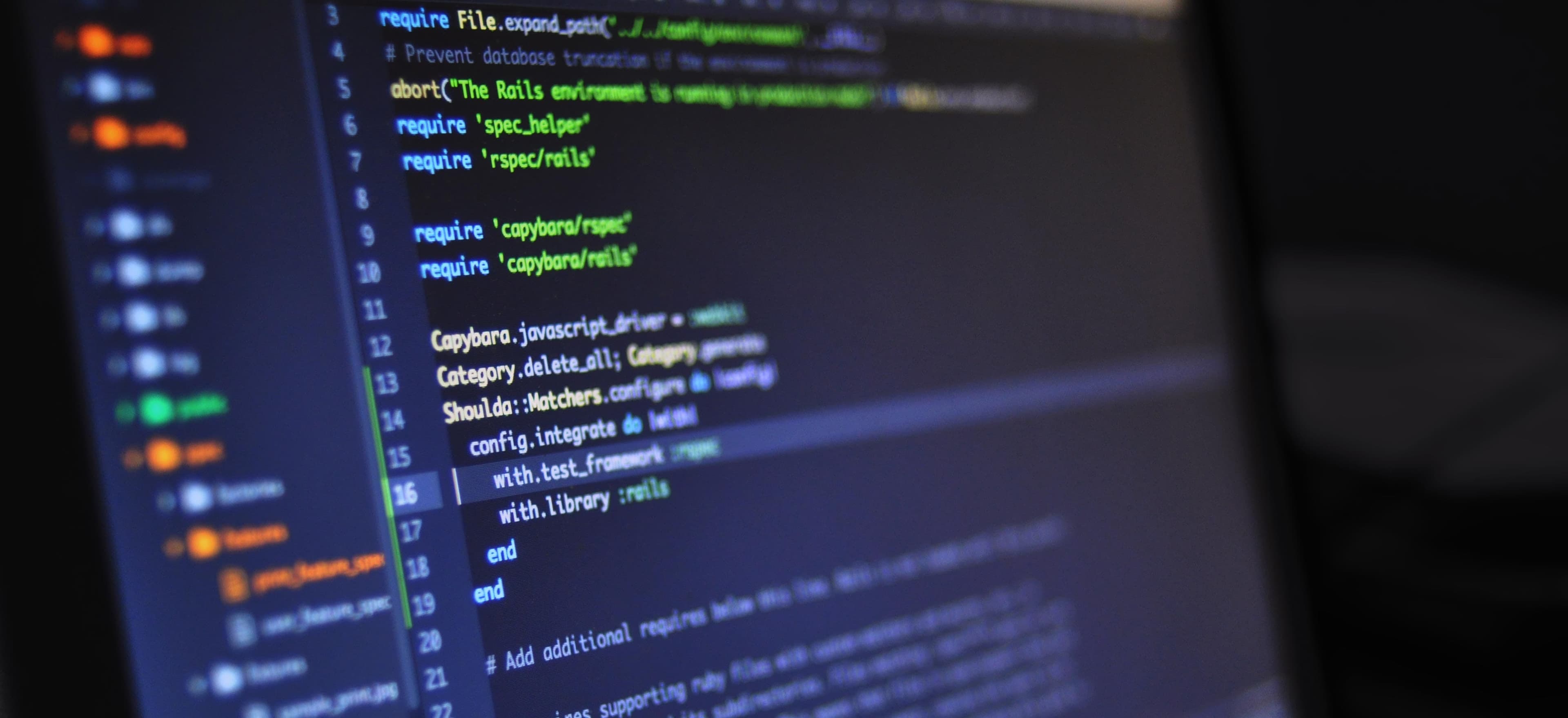
- Published on
Solving JDBC Realm Authentication in GlassFish & PrimeFaces
JDBC Realm authentication is a popular and widely used method for securing Java EE applications. GlassFish, an open-source application server, and PrimeFaces, a lightweight UI component suite, are commonly used in combination to build robust web applications. In this blog post, we will explore the process of integrating JDBC Realm authentication in a web application using GlassFish as the application server and PrimeFaces for the front-end. We will cover the necessary configuration steps, implementation of custom login forms, and the underlying Java code. By the end of this post, you will have a solid understanding of how to implement JDBC Realm authentication in your own Java web applications.
Setting Up GlassFish Server
Before we delve into the implementation, let's make sure that GlassFish Server is set up and running. If you haven't installed GlassFish yet, you can download it from the official website. Once installed, start the GlassFish Server by navigating to the installation directory and running the following command:
./bin/asadmin start-domain
After starting the server, you can access the GlassFish Admin Console by navigating to http://localhost:4848 in your web browser. Here, you can perform administrative tasks and configure resources for your applications.
Configuring JDBC Realm in GlassFish
GlassFish Server supports JDBC Realm for user authentication. To configure and set up a JDBC Realm, follow these steps:
- Create a Database for Authentication: Before configuring the JDBC Realm, you need to have a database with a table for storing user credentials. Here is an example of a table schema for user authentication:
CREATE TABLE users (
username VARCHAR(50) PRIMARY KEY,
password VARCHAR(100)
);
-
Setting up JDBC Connection Pool: In the GlassFish Admin Console, navigate to Resources > JDBC > JDBC Connection Pools. Create a new JDBC Connection Pool for your authentication database.
-
Creating JDBC Resource: After creating the connection pool, navigate to Resources > JDBC > JDBC Resources. Create a new JDBC Resource for the authentication database, using the previously created connection pool.
-
Configuring JDBC Realm: Finally, navigate to Configurations > server-config > Security > Realms and click on the "New" button. Choose "Database Realm" as the type and configure the realm to use the JDBC Resource created in the previous step. Specify the SQL queries for retrieving user credentials.
Implementing Login Functionality with PrimeFaces
With GlassFish configured with JDBC Realm, let's proceed with implementing the login functionality using PrimeFaces.
- Add PrimeFaces Dependency: Include the PrimeFaces dependency in your project's
pom.xml
if you are using Maven:
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>{latest version}</version>
</dependency>
- Create Login Form: Design a login form using PrimeFaces components in your JSF page. Here's an example of a simple login form with input fields for username and password:
<h:form>
<p:panelGrid columns="2">
<h:outputLabel for="username" value="Username:" />
<p:inputText id="username" value="#{loginBean.username}" required="true" />
<h:outputLabel for="password" value="Password:" />
<p:password id="password" value="#{loginBean.password}" required="true" />
</p:panelGrid>
<p:commandButton value="Login" action="#{loginBean.login}" />
</h:form>
- Implementing the LoginBean: Create a managed bean in your application to handle the login functionality. This bean will interact with the GlassFish JDBC Realm for user authentication. Here's a simplified example of the
LoginBean
:
@ManagedBean
@SessionScoped
public class LoginBean implements Serializable {
private String username;
private String password;
public String login() {
FacesContext context = FacesContext.getCurrentInstance();
HttpServletRequest request = (HttpServletRequest) context.getExternalContext().getRequest();
try {
request.login(username, password);
return "home?faces-redirect=true";
} catch (ServletException e) {
context.addMessage(null, new FacesMessage("Login failed: Invalid username or password"));
return "login";
}
}
// Other methods such as logout, etc.
}
In the login
method, we use the HttpServletRequest
to invoke the GlassFish JDBC Realm's authentication. Upon successful authentication, the user is redirected to the home page. If authentication fails, an error message is displayed, and the user stays on the login page.
To Wrap Things Up
In this blog post, we walked through the process of configuring JDBC Realm authentication in GlassFish and implementing the login functionality using PrimeFaces. By following these steps, you can create a robust and secure authentication mechanism for your Java web applications. JDBC Realm authentication provides a convenient way to handle user authentication with the support of database-backed credentials. When used in conjunction with a powerful UI component library like PrimeFaces, the result is a seamless and user-friendly authentication experience.
With the wealth of resources and tutorials available, such as the official documentation on GlassFish and PrimeFaces user guide, you can further explore and enhance the authentication process to meet your specific application requirements. Embracing best practices and staying informed about security considerations will contribute to the creation of a robust and secure web application.