Optimizing Performance for Bitmap Visualization
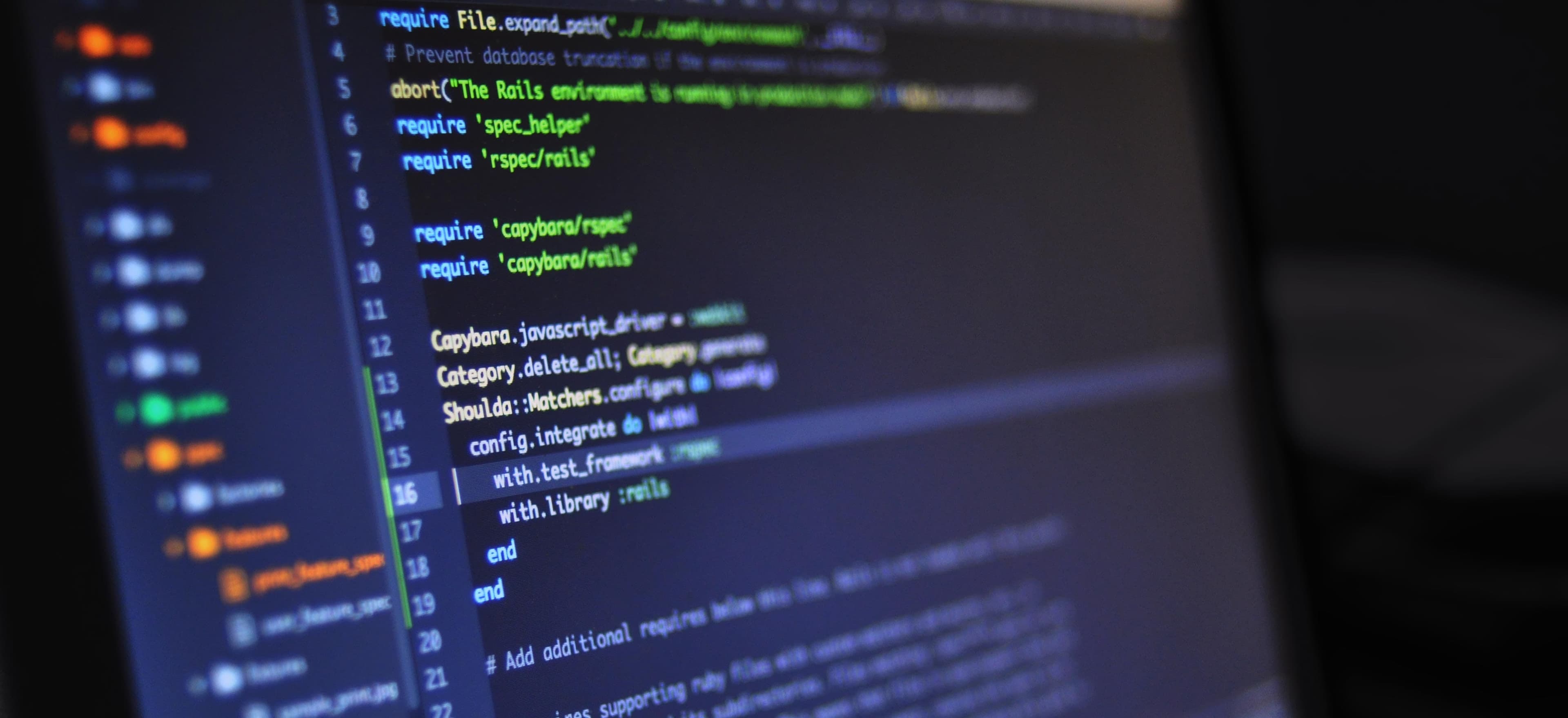
- Published on
Optimizing Performance for Bitmap Visualization in Java
Bitmap visualization is a common task in various Java applications, including image processing, computer graphics, and GUI development. However, when dealing with large bitmaps, performance optimization becomes crucial to ensure smooth rendering and efficient memory usage. In this article, we will explore some techniques for optimizing the performance of bitmap visualization in Java.
Understanding the Problem
Before delving into optimization techniques, it's important to understand why performance might be an issue when visualizing bitmaps in Java. The key challenges include:
- Memory Usage: Large bitmaps can consume significant amounts of memory, leading to potential OutOfMemory errors or excessive garbage collection overhead.
- Rendering Speed: Rendering large bitmaps pixel by pixel can be slow, especially when dealing with complex visualizations or frequent updates.
- Resource Management: Inefficient resource management, such as not releasing resources after bitmap processing, can lead to performance degradation over time.
Choosing the Right Data Structure
When working with bitmaps, choosing the right data structure can significantly impact performance. In Java, the BufferedImage
class is commonly used for bitmap manipulation and visualization. By understanding the characteristics of different BufferedImage
types, you can optimize memory usage and rendering speed.
// Create a BufferedImage with TYPE_INT_ARGB for optimal performance
BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_ARGB);
The TYPE_INT_ARGB
type provides optimal performance for bitmap manipulation, especially when dealing with complex visualizations or alpha channel transparency.
Implementing Lazy Loading
In scenarios where you are dealing with large bitmaps, it's essential to incorporate lazy loading to defer the initialization of the bitmap data until it is actually required for visualization. This can help reduce memory consumption and improve application startup times.
// Implementing lazy loading for bitmap data
public class LazyBitmap {
private byte[] bitmapData;
public byte[] getBitmapData() {
if (bitmapData == null) {
// Load the bitmap data from disk or network
// ...
}
return bitmapData;
}
}
By lazily loading bitmap data only when needed, you can avoid unnecessary memory overhead and improve the overall responsiveness of your application.
Utilizing Hardware Acceleration
Java provides support for hardware-accelerated graphics rendering through the Java 2D API. By leveraging hardware acceleration, you can offload the rendering workload to the GPU, leading to improved performance, especially when dealing with complex bitmap visualizations.
// Enable hardware acceleration for graphics rendering
GraphicsConfiguration config = GraphicsEnvironment.getLocalGraphicsEnvironment().getDefaultScreenDevice().getDefaultConfiguration();
BufferedImage acceleratedImage = config.createCompatibleImage(width, height);
By creating a compatible image with the default configuration, you can take advantage of hardware acceleration for bitmap visualization.
Memory Management and Recycling
Efficient memory management is critical when dealing with large bitmaps in Java. Failing to release memory resources can lead to memory leaks and degraded application performance. The SoftReference
class can be used to handle memory more effectively.
// Using SoftReference for efficient memory management
SoftReference<BufferedImage> softReference = new SoftReference<>(bufferedImage);
By using SoftReference
, you allow the garbage collector to prioritize memory reclamation based on available system memory, which can help prevent OutOfMemory errors when working with large bitmaps.
Parallel Processing for Bitmap Manipulation
When performing intensive bitmap manipulation operations, such as image filtering or transformation, leveraging parallel processing can significantly improve performance by distributing the workload across multiple CPU cores.
// Using parallel streams for bitmap manipulation
int[] pixels = bufferedImage.getRGB(0, 0, width, height, null, 0, width);
Arrays.parallelSetAll(pixels, i -> /* bitmap manipulation logic */);
bufferedImage.setRGB(0, 0, width, height, pixels, 0, width);
By utilizing parallel streams, you can harness the power of multicore processors to process bitmap data more efficiently.
Caching and Pre-rendering
Caching frequently used bitmap visualizations and pre-rendering complex visual components can help improve rendering speed and reduce computational overhead, especially in scenarios where the same bitmap visualization is reused across multiple instances.
// Caching pre-rendered bitmap visualizations
Map<String, BufferedImage> visualizationCache = new HashMap<>();
visualizationCache.put("key", preRenderedBitmap);
By caching pre-rendered bitmap visualizations, you can avoid redundant rendering operations and improve the overall responsiveness of your application.
My Closing Thoughts on the Matter
Optimizing the performance of bitmap visualization in Java involves a combination of efficient memory management, hardware acceleration, parallel processing, and smart resource utilization. By carefully selecting the right data structures, leveraging hardware acceleration, implementing lazy loading, and incorporating parallel processing, you can enhance the performance and responsiveness of bitmap visualization in your Java applications.
Incorporating these optimization techniques can lead to smoother, more efficient bitmap visualization, especially when working with large bitmaps or complex visualizations in Java.
Remember that performance optimization is a continuous process, and thorough profiling and testing are essential to identify and address performance bottlenecks in bitmap visualization.
By implementing these optimization techniques, you can ensure that your Java applications deliver exceptional bitmap visualization performance, providing a seamless user experience and efficient resource utilization.
Ensure that when dealing with large bitmaps, performance optimization becomes crucial to ensure smooth rendering and efficient memory usage. Let's explore some techniques for optimizing the performance of bitmap visualization in Java.