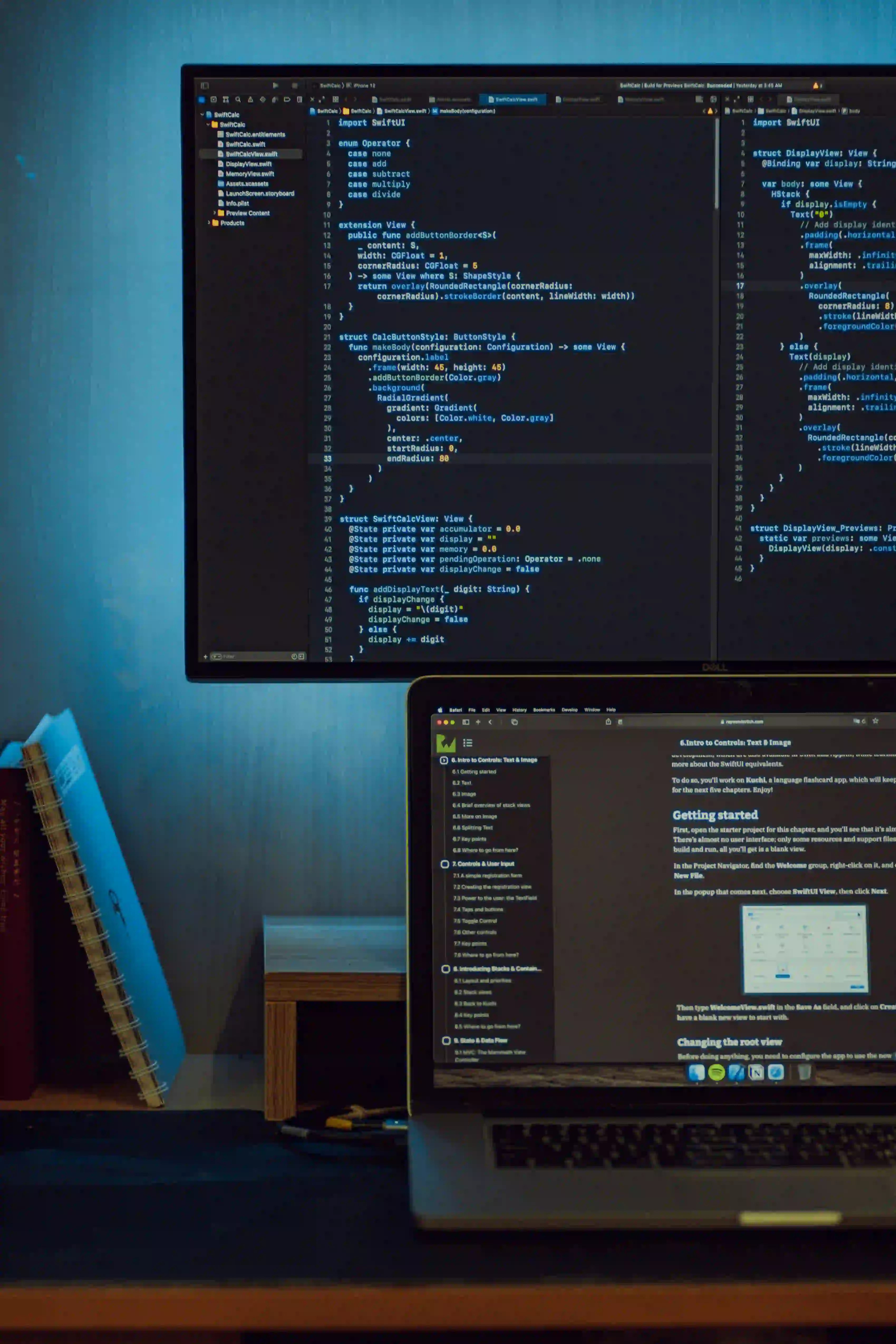
Mastering OAuth2 JWT: Solving Centralized Authorization Woes
In the world of web development, security is paramount. One of the most crucial aspects of securing web applications is ensuring that only authorized users have access to sensitive resources. This is where OAuth2 and JSON Web Tokens (JWT) come into play. In this blog post, we'll delve into the world of OAuth2 JWT, exploring its inner workings, implementation, and the benefits it offers for securing your Java applications.
Understanding OAuth2 and JWT
OAuth2: The Authorization Framework
OAuth2 is an industry-standard protocol for authorization. It allows a user to grant a third-party application limited access to their resources, without revealing their credentials. The OAuth2 framework consists of various flows (e.g., Authorization Code, Implicit, Client Credentials, etc.) that cater to different use cases. It is widely used for securing APIs and enabling single sign-on (SSO) for web and mobile applications.
JWT: Compact and Self-Contained Tokens
JSON Web Tokens (JWT) are a compact, URL-safe means of representing claims to be transferred between two parties. These claims are typically used for authentication and authorization. JWTs are digitally signed, enabling secure transmission of information between parties. They are often used as access tokens within OAuth2 flows, providing a stateless way to carry user identity information.
Implementing OAuth2 JWT in Java
Using Spring Security OAuth2
When it comes to implementing OAuth2 in Java, Spring Security OAuth2 stands out as a popular choice. Spring Security OAuth2 provides comprehensive support for implementing OAuth2 and JWT-based authentication and authorization in Java applications. It seamlessly integrates with Spring Security, making it relatively straightforward to secure your APIs and web applications.
Let's take a look at a simple configuration for enabling OAuth2 and JWT in a Spring Boot application:
@Configuration
@EnableWebSecurity
@EnableAuthorizationServer
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user")
.password(passwordEncoder().encode("password"))
.roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In this configuration, we enable OAuth2 and JWT support using Spring Security OAuth2. We also specify an in-memory user with a role and password. This is a simplified example; in a real-world scenario, user details would be fetched from a database or an external identity provider.
Generating and Validating JWTs
Now that we have our OAuth2 and JWT infrastructure in place, let's focus on the generation and validation of JWTs. Spring Security OAuth2 takes care of this for us, but it's essential to understand the underlying process.
When a user authenticates and obtains an access token, it's a JWT that they receive. This token contains information about the user and their granted authorities. On subsequent requests to secure endpoints, this token is included in the Authorization
header of the HTTP request.
@RestController
public class UserController {
@GetMapping("/user")
public Principal retrieveUser(Principal principal) {
return principal;
}
}
In this example, the UserController
retrieves user information based on the provided JWT. Spring Security OAuth2 ensures that the JWT is valid and extracts the user details, making them available for use within the application.
Benefits of OAuth2 JWT
Stateless Authentication and Authorization
One of the primary advantages of using OAuth2 JWT for securing your Java applications is the stateless nature of JWTs. Traditional session-based authentication requires server-side storage of session information, leading to scalability and performance challenges, especially in distributed systems. JWTs, being self-contained and digitally signed, alleviate these issues by enabling stateless authentication and authorization.
Enhanced Security
JWTs, due to their digital signature, provide a high level of security. This ensures that the token has not been tampered with, providing integrity and authenticity to the claims within the token. Additionally, the use of HTTPS for transmitting JWTs further strengthens their security. This makes OAuth2 JWT a robust choice for securing your Java applications, especially when dealing with microservices and distributed architectures.
Interoperability and Standardization
OAuth2 and JWT are widely adopted standards in the industry, enjoying broad support across various programming languages and platforms. This means that JWTs issued by an OAuth2 server in a Java application can be seamlessly validated and utilized by services written in different languages, promoting interoperability and easing integration efforts in a multi-technology ecosystem.
To Wrap Things Up
In a world where security is non-negotiable, OAuth2 JWT emerges as a potent mechanism for securing Java applications. Its stateless nature, robust security, and broad industry support make it an attractive choice for implementing authentication and authorization. With the power of Spring Security OAuth2, incorporating OAuth2 JWT into your Java applications becomes accessible and effective.
In this blog post, we've only scratched the surface of OAuth2 JWT in the context of Java. It's a vast topic with intricacies that warrant further exploration, especially in the context of real-world applications and complex use cases.
Whether you're building RESTful APIs, microservices, or web applications, understanding and mastering OAuth2 JWT can significantly bolster the security posture of your Java applications. Embrace the power of OAuth2 JWT and embark on a journey to conquer the centralized authorization woes that may plague your development endeavors.
So go ahead, dive deeper, experiment, and elevate your Java application security with OAuth2 JWT!
For more in-depth insights and hands-on tutorials, visit the official Spring Security documentation and JWT.io for comprehensive JWT resources. Happy coding and stay secure!