Common Pitfalls in API Designing
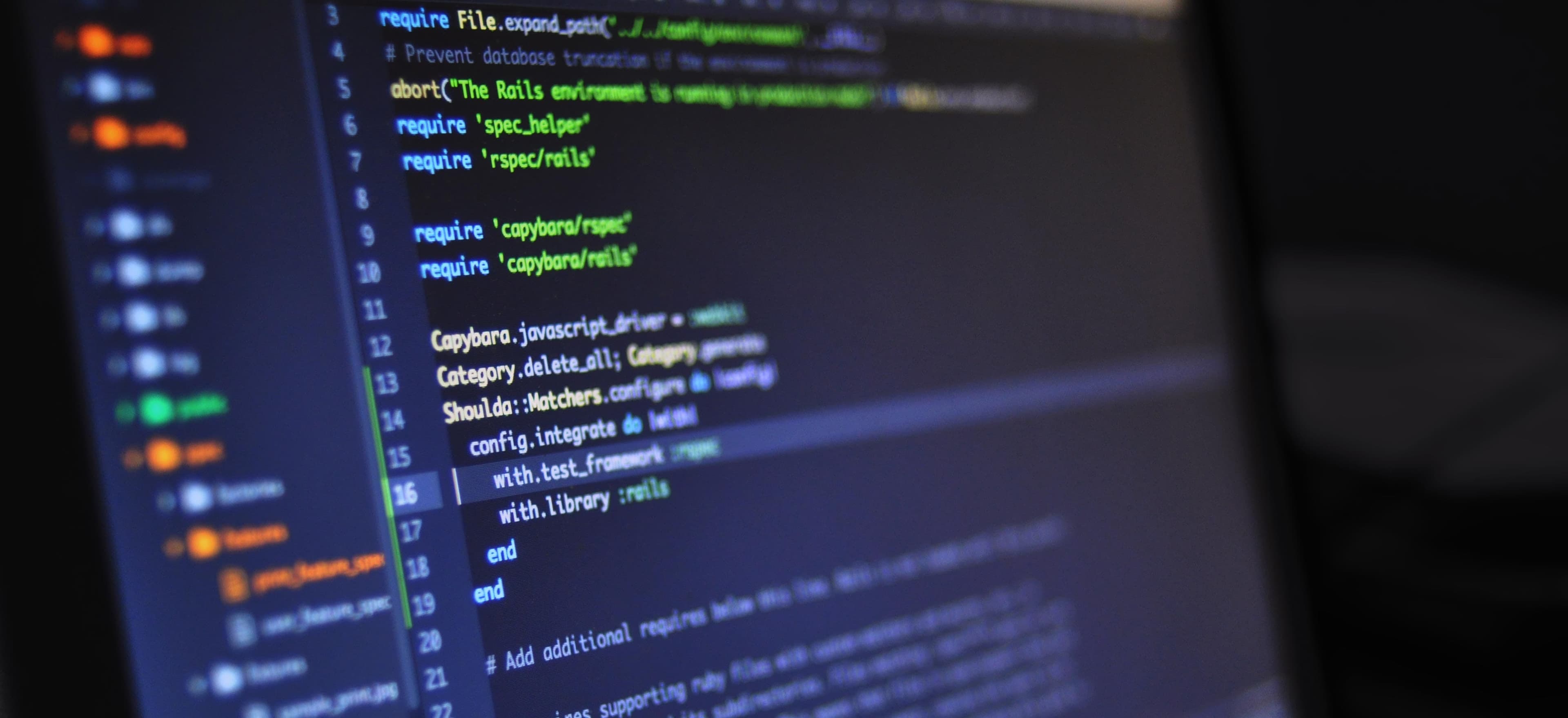
- Published on
Common Pitfalls in API Designing
When it comes to designing APIs in Java, there are numerous pitfalls that developers often encounter. Whether it's a lack of consistency, poor error handling, or inefficient resource utilization, these pitfalls can hinder the performance and usability of the API. In this blog post, we'll explore some of the common pitfalls in API designing and discuss best practices to avoid them.
Lack of Consistency
One of the most common pitfalls in API designing is the lack of consistency in naming conventions, parameter ordering, and response formats. Inconsistent naming can confuse developers who are consuming the API, making it difficult for them to understand how to use it effectively.
When designing an API, it's crucial to establish clear and consistent naming conventions for endpoints, parameters, and responses. This can greatly improve the usability of the API and make it easier for developers to work with.
// Inconsistent naming
public void getUserInfo(String id);
public void fetchUserData(Integer userId);
// Consistent naming
public void getUserInfo(int userId);
Poor Error Handling
Another common pitfall is the inadequate handling of errors within the API. Failing to handle errors properly can lead to unexpected behavior and make it challenging for developers to diagnose and resolve issues.
When designing an API, it's essential to define clear error messages and status codes for different error scenarios. Additionally, the API should provide meaningful and descriptive error messages to help developers understand what went wrong.
// Poor error handling
if (user == null) {
return "User not found";
}
// Improved error handling
if (user == null) {
throw new UserNotFoundException("User not found");
}
Unnecessary Complexity
It's common for APIs to become unnecessarily complex, with convoluted endpoint structures and excessive use of parameters. This complexity can make the API difficult to use and understand, leading to increased development time and potentially more bugs.
To avoid unnecessary complexity, it's important to keep the API simple and focused on specific use cases. The endpoints should be intuitive and easy to navigate, with minimal required parameters.
// Unnecessary complexity
public List<User> getUsersByRoleAndStatus(String role, String status, int page, int pageSize);
// Simplified approach
public List<User> getUsersByRole(String role);
Inefficient Resource Utilization
Inefficient resource utilization, such as excessive use of memory or CPU, can significantly impact the performance of an API. This can lead to slow response times and increased server costs.
When designing an API, it's essential to consider resource utilization and optimize the code for performance. This includes minimizing unnecessary database queries, caching data where appropriate, and using efficient data structures and algorithms.
// Inefficient resource utilization
public List<User> getAllUsers() {
return userRepository.findAll(); // Fetches all users from the database
}
// Improved resource utilization
public List<User> getAllUsers() {
if (cachedUsers != null) {
return cachedUsers;
} else {
List<User> users = userRepository.findAll();
cachedUsers = users;
return users;
}
}
Overlooking Documentation
One of the most critical pitfalls in API designing is the oversight of comprehensive documentation. Failing to document the API thoroughly can make it challenging for developers to understand how to use it effectively, leading to confusion and frustration.
When designing an API, it's crucial to provide clear and comprehensive documentation, including details on endpoints, parameters, responses, and error handling. This documentation should be easily accessible and regularly updated to reflect any changes to the API.
Wrapping Up
In conclusion, designing an API in Java comes with its fair share of pitfalls, from lack of consistency and poor error handling to unnecessary complexity and inefficient resource utilization. By adhering to best practices and considering the challenges discussed in this blog post, developers can create well-designed APIs that are easy to use, maintain, and perform efficiently.
To delve deeper into API design best practices, consider exploring resources such as Oracle's Java API Design Guide and RESTful API Design Best Practices. By continually refining and enhancing their API designing skills, developers can contribute to the creation of robust and user-friendly APIs in the Java ecosystem.
Checkout our other articles