Maximizing Efficiency: Solving Concurrency in Object Pools
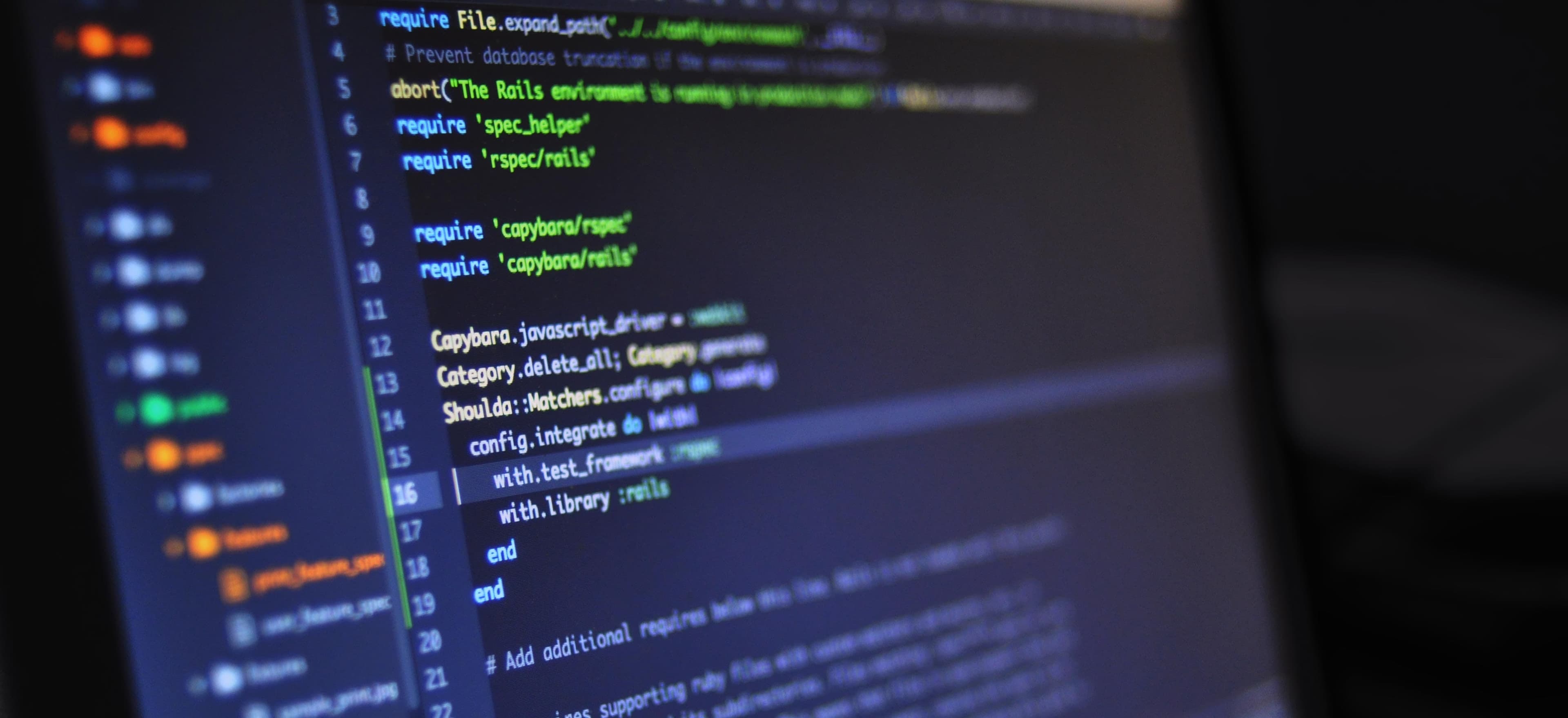
- Published on
Maximizing Efficiency: Solving Concurrency in Object Pools
In the world of Java programming, concurrency is a significant challenge that requires thoughtful consideration and strategic solutions. One common scenario that demands concurrent programming techniques is managing object pools efficiently.
What are Object Pools?
Object pools are a well-established concept in software engineering, especially in Java. They are designed to manage and provide a reusable set of objects, thus reducing the overhead of creating and destroying objects. Object pools are often used in scenarios where object creation is expensive, such as with database connections, thread management, and other resource-intensive tasks.
Concurrency Challenges in Object Pools
When dealing with object pools, concurrency challenges arise when multiple threads require access to the objects within the pool. In a multi-threaded environment, issues such as race conditions, deadlock, and resource contention can occur if concurrency is not managed effectively. To address these challenges, it's crucial to implement concurrency control mechanisms that ensure thread safety and optimal performance.
The Role of Java Concurrency in Object Pool Management
Java provides a robust set of concurrency utilities and mechanisms to tackle these challenges. Leveraging these tools effectively can lead to efficient and scalable object pool management.
Let's delve into some key techniques and best practices for solving concurrency issues in Java object pools.
Use Case: Object Reclamation in Object Pools
One common challenge in object pool management is the reclamation of objects. A scenario may arise where an object is currently in use by one thread, but another thread needs access to it. Efficient object reclamation is critical in such cases to avoid contention and bottlenecks.
Leveraging java.util.concurrent
for Object Pool Management
The java.util.concurrent
package offers a rich set of concurrency utilities that can be harnessed for effective object pool management. One such utility is the Semaphore
class, which provides a simple yet powerful way to control access to a shared resource.
import java.util.concurrent.Semaphore;
public class ObjectPool<T> {
private final Semaphore available;
private final List<T> pool;
public ObjectPool(int size, List<T> objects) {
available = new Semaphore(size, true);
pool = new ArrayList<>(objects);
}
public T acquire() throws InterruptedException {
available.acquire();
return getObjectFromPool();
}
public void release(T object) {
if (returnObjectToPool(object)) {
available.release();
}
}
// Other methods for managing the pool
}
In the above example, a Semaphore
is used to control access to objects in the pool. When a thread needs an object, it calls the acquire()
method, which blocks if no objects are available. Upon releasing the object, the release()
method signals the Semaphore
to make the object available for another thread.
Leveraging ReentrantLock
for Fine-Grained Control
While Semaphore
provides a high-level abstraction for controlling resource access, sometimes a more fine-grained control is necessary. This is where the ReentrantLock
class comes into play. It allows for more flexibility and advanced control over resource access.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class ObjectPool<T> {
private final Lock lock;
private final List<T> pool;
public ObjectPool(List<T> objects) {
lock = new ReentrantLock(true);
pool = new ArrayList<>(objects);
}
public T acquire() {
lock.lock();
try {
return getObjectFromPool();
} finally {
lock.unlock();
}
}
public void release(T object) {
lock.lock();
try {
returnObjectToPool(object);
} finally {
lock.unlock();
}
}
// Other methods for managing the pool
}
In this example, the ReentrantLock
is used to guard the critical sections of the object pool, providing exclusive access to the pool's resources. It's important to always release the lock in a finally
block to ensure it's released even if an exception occurs.
Leveraging Concurrent Collections for Object Pools
Java's concurrent collections, such as ConcurrentHashMap
and ConcurrentLinkedQueue
, provide thread-safe alternatives to their non-concurrent counterparts. These collections are designed to be used in concurrent environments, making them well-suited for managing object pools in multi-threaded applications.
import java.util.Queue;
import java.util.concurrent.ConcurrentLinkedQueue;
public class ObjectPool<T> {
private final Queue<T> pool;
public ObjectPool(List<T> objects) {
pool = new ConcurrentLinkedQueue<>(objects);
}
public T acquire() {
return pool.poll();
}
public void release(T object) {
pool.offer(object);
}
// Other methods for managing the pool
}
In this example, the ConcurrentLinkedQueue
is used to manage the objects in the pool. The poll()
and offer()
methods are inherently thread-safe, providing a seamless and efficient way to acquire and release objects from the pool.
A Final Look
Effective concurrency management is crucial for optimizing the performance and scalability of object pools in Java applications. By leveraging Java's robust concurrency utilities, such as Semaphore
, ReentrantLock
, and concurrent collections, developers can implement efficient and thread-safe object pool management mechanisms.
Concurrency in object pools is a broad and dynamic topic, and these are just a few essential strategies to consider. As with any aspect of software development, a deep understanding of the problem domain and continuous learning are key to mastering concurrency in object pool management.
Concurrency can be complex, but with the right tools and techniques, Java developers can conquer the challenges and maximize the efficiency of their object pools, enabling smooth and responsive multi-threaded applications.