Solving User Authentication Errors in Spring MVC Security
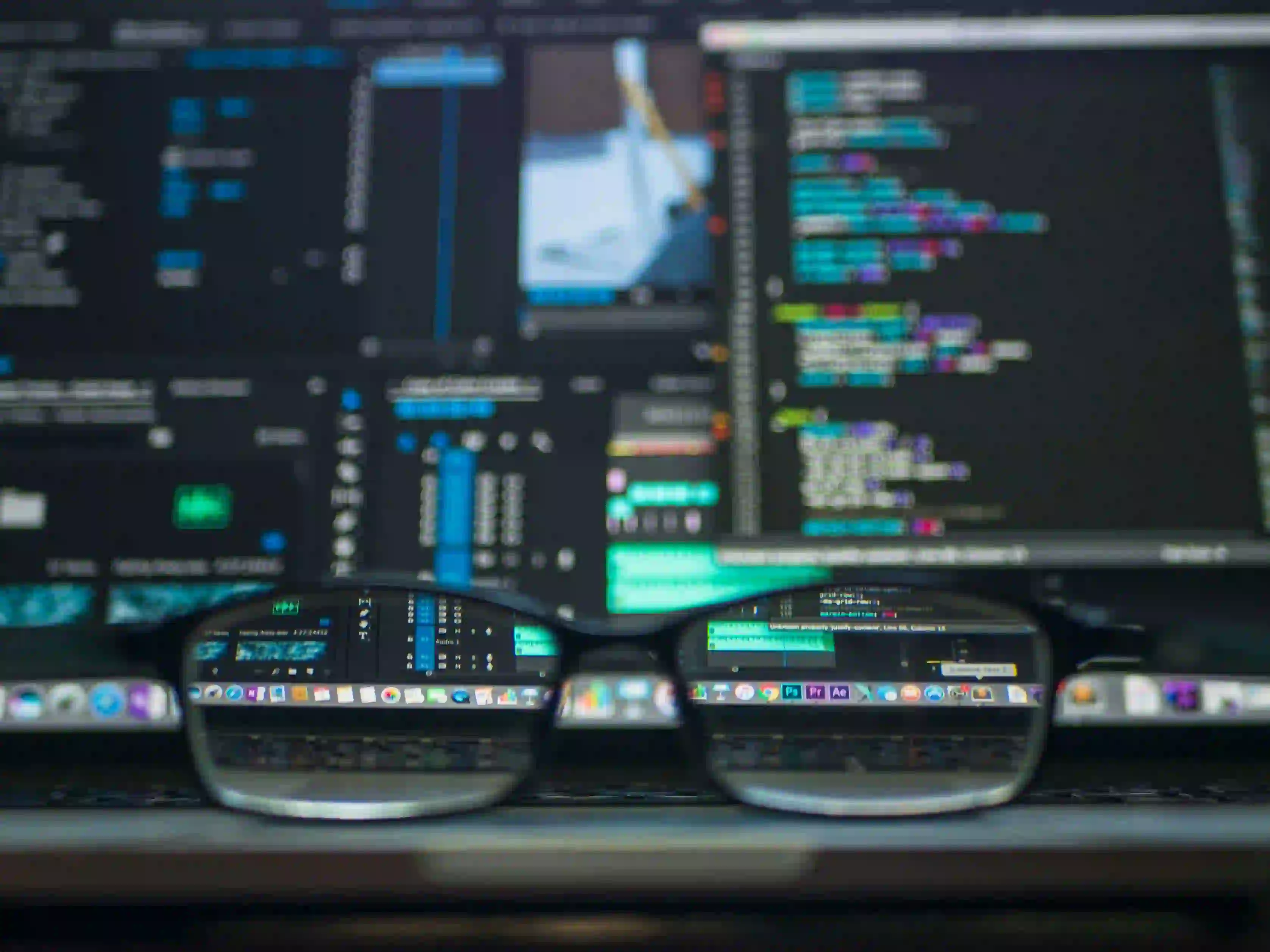
Solving User Authentication Errors in Spring MVC Security
User authentication is a crucial aspect of web application development, especially when it comes to secure user access. In the context of Java web development, Spring MVC Security provides a robust framework for implementing authentication and authorization mechanisms.
In this article, we will explore common user authentication errors that developers may encounter when working with Spring MVC Security, and provide solutions to resolve these issues effectively.
Understanding User Authentication in Spring MVC Security
Before delving into specific errors and their solutions, let's briefly recap the fundamentals of user authentication in Spring MVC Security.
Spring MVC Security uses the concept of AuthenticationProvider to handle user authentication. When a user attempts to log in, the UsernamePasswordAuthenticationToken is created with the provided username and password. This token is then passed to the authentication manager, which in turn delegates the authentication process to one or more configured AuthenticationProviders.
Once the user is successfully authenticated, a GrantedAuthority is assigned to the user, specifying their level of access within the application.
Common User Authentication Errors and Their Solutions
Error 1: "No AuthenticationProvider found for org.springframework.security.authentication.UsernamePasswordAuthenticationToken"
This error typically occurs when Spring Security is unable to locate an AuthenticationProvider that supports the provided UsernamePasswordAuthenticationToken.
Solution:
To resolve this error, ensure that you have configured an AuthenticationProvider that supports the UsernamePasswordAuthenticationToken. You can achieve this by creating a custom AuthenticationProvider that extends the AbstractUserDetailsAuthenticationProvider and overrides the additionalAuthenticationChecks method.
Here's an example of how to create a custom AuthenticationProvider:
@Component
public class CustomAuthenticationProvider extends AbstractUserDetailsAuthenticationProvider {
@Override
protected void additionalAuthenticationChecks(UserDetails userDetails, UsernamePasswordAuthenticationToken authentication) throws AuthenticationException {
// Add your custom authentication logic here
}
@Override
protected UserDetails retrieveUser(String username, UsernamePasswordAuthenticationToken authentication) throws AuthenticationException {
// Retrieve user details from your data source
}
}
Error 2: "UserDetailsService returned null, which is an interface contract violation"
This error occurs when the UserDetailsService implementation returns null, indicating that the user details are not found based on the provided username.
Solution:
Ensure that your UserDetailsService implementation correctly retrieves user details from the data source and returns a valid UserDetails object. Double-check the implementation to handle scenarios where the user details are not found, and throw a UsernameNotFoundException if necessary.
Here's an example of a basic UserDetailsService implementation:
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found with username: " + username);
}
return new CustomUserDetails(user);
}
}
Error 3: "Bad credentials"
The "Bad credentials" error is a common indication that the provided username or password is incorrect during the authentication process.
Solution:
Double-check the authenticity of the provided credentials against the stored user credentials. Verify the password encryption and hashing mechanisms to ensure that the comparison is accurate.
When using password hashing, it's essential to employ compatible encryption algorithms and ensure that the stored password is correctly hashed and compared with the user input.
Wrapping Up
In this article, we've explored common user authentication errors in Spring MVC Security and provided practical solutions to address these issues effectively. By understanding the underlying causes of these errors and implementing the recommended solutions, developers can enhance the reliability and security of user authentication within their Spring MVC applications.
Implementing robust user authentication is critical for securing web applications, and Spring MVC Security offers a comprehensive framework to achieve this goal effectively.
Remember, thorough testing and continuous validation of the authentication process are essential to ensure the seamless and secure user experience within your Java web applications.
For further in-depth insights into Spring MVC Security and user authentication best practices, refer to the official Spring Security documentation.
By addressing and resolving user authentication errors, developers can elevate the security and reliability of their Spring MVC applications, ensuring a seamless and secure user experience.
For further reading on advanced Spring Security features, explore the latest version of the Spring Security reference documentation, which provides comprehensive insights and best practices for implementing secure user authentication.