Mastering Java 8 Logging: Unlock Deferred Invocation
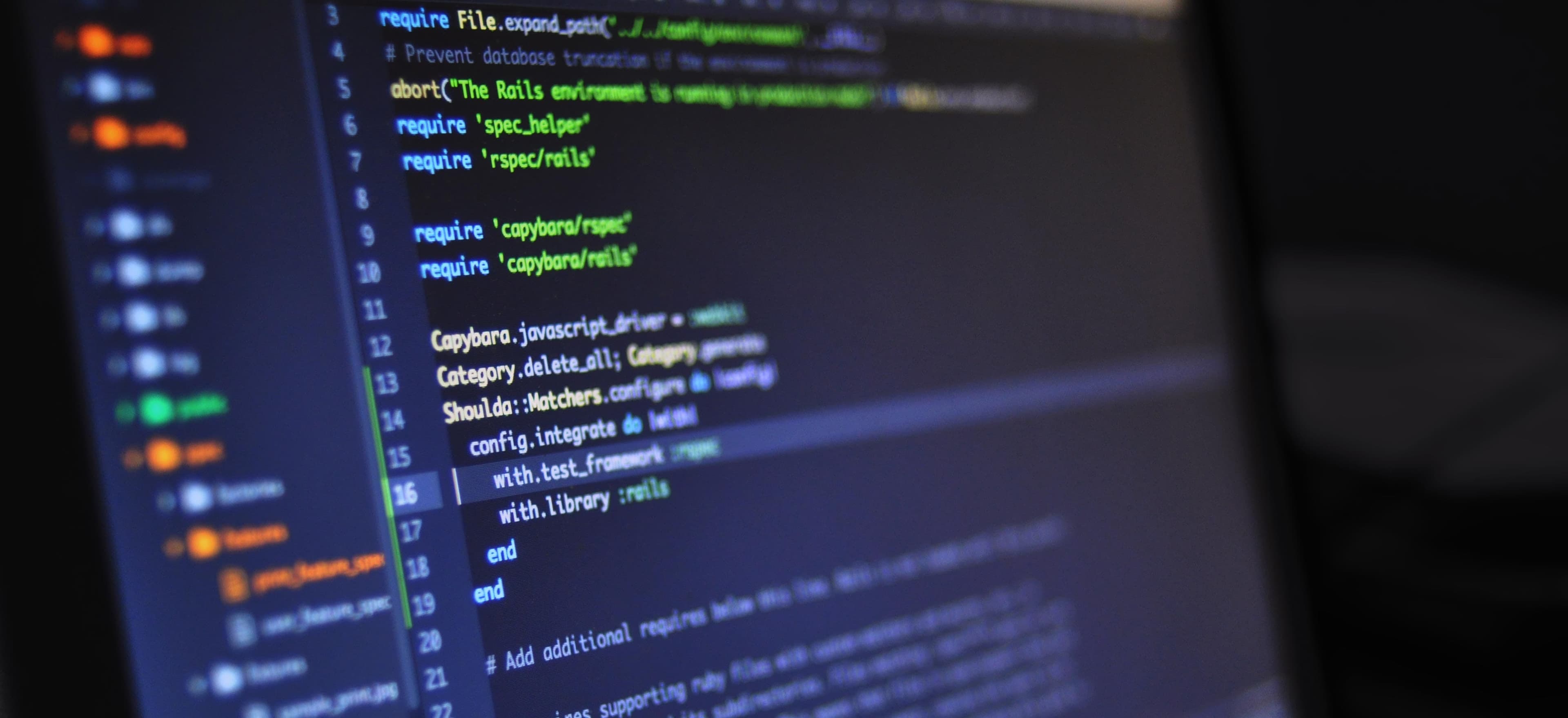
- Published on
Mastering Java 8 Logging: Unlock Deferred Invocation
Logging is an essential aspect of application development and is crucial for monitoring and debugging. In Java, logging is typically facilitated through the use of the java.util.logging
package. With the introduction of Java 8, a powerful logging enhancement known as deferred invocation was introduced, providing a more efficient and flexible way to manage logging. This article will delve into the intricacies of deferred invocation and demonstrate its implementation in Java 8.
Understanding Deferred Invocation
Deferred invocation is a fundamental concept in Java 8 logging that allows for the deferred execution of expensive or time-consuming log messages. It is particularly beneficial when the log messages are not expected to be output due to the logging level, thereby optimizing performance by avoiding unnecessary computations.
Consider a scenario where a log message is required to compute and format a complex message that may never be used if the log level is set to a higher severity, such as INFO
or ERROR
. In traditional logging, the message computation would occur regardless of whether it is actually logged. However, with deferred invocation, the message computation is postponed until it is determined that the message will be logged, based on the logging level.
Deferred Invocation in Java 8
In Java 8, the Supplier
functional interface plays a pivotal role in enabling deferred invocation for logging. The Supplier
interface represents a supplier of results and facilitates deferred execution by providing a mechanism to supply a value only when it is required.
Let's delve into a practical example to illustrate the implementation of deferred invocation in Java 8 logging.
import java.util.logging.Logger;
import java.util.function.Supplier;
public class DeferredLoggingExample {
private static final Logger LOGGER = Logger.getLogger(DeferredLoggingExample.class.getName());
public void performComputation() {
// Expensive computation or operation
String result = "Result of computation";
LOGGER.fine(() -> "Computed result: " + result); // Deferred invocation using Supplier
}
}
In the example above, the fine
level log message is associated with a lambda expression that serves as a Supplier<String>
. This lambda expression is only invoked if the logging level is set to FINE
or a lower severity; otherwise, the message computation is avoided entirely.
Leveraging Deferred Invocation for Performance Optimization
The application of deferred invocation can significantly optimize performance by circumventing unnecessary computations for log messages that may not be output based on the logging level. This becomes especially prominent in scenarios where the log messages involve intricate computations or operations that incur performance overhead.
By utilizing deferred invocation, developers can ensure that expensive log messages are only computed when they are deemed necessary, mitigating performance bottlenecks and enhancing the overall efficiency of the application.
Configuring Logging Levels for Deferred Invocation
An essential aspect of realizing the benefits of deferred invocation is the proper configuration of logging levels. In Java 8, this can be achieved through the logging.properties
configuration file, which allows for the fine-tuning of logging behavior and levels.
Let's consider the following excerpt from a sample logging.properties
file:
handlers=java.util.logging.ConsoleHandler
java.util.logging.ConsoleHandler.level=FINE
com.example.application.level=INFO
In the above configuration, the logging level for the ConsoleHandler
is set to FINE
, indicating that messages with a severity level of FINE
or lower will be output. Additionally, the logging level for the com.example.application
package is set to INFO
, implying that messages with a severity level of INFO
or lower will be logged.
By appropriately configuring the logging levels, developers can harness the power of deferred invocation to optimize performance and streamline logging operations.
Advantages of Deferred Invocation
- Performance Optimization: Deferred invocation mitigates unnecessary computations for log messages not expected to be output, thereby enhancing application performance.
- Flexible Logging: With deferred invocation, log messages can contain complex computations without incurring performance overhead unless they are actually logged.
My Closing Thoughts on the Matter
In conclusion, mastering deferred invocation in Java 8 logging is pivotal for optimizing application performance and streamlining logging operations. By leveraging the Supplier
functional interface and configuring logging levels appropriately, developers can effectively implement deferred invocation to defer expensive log message computations and ensure efficient logging behavior.
Deferred invocation stands as a testament to the continual evolution and enhancement of logging capabilities in Java, presenting developers with a powerful mechanism to optimize application performance and logging operations.
By incorporating deferred invocation into logging practices, Java developers can elevate the efficiency and performance of their applications while maintaining robust logging capabilities.
For further exploration of Java logging and deferred invocation, refer to the official Java Logging API documentation.
Incorporating deferred invocation into your Java 8 logging practices can yield significant performance benefits and optimize your application's logging behavior. Mastering this feature empowers developers to strike a balance between comprehensive logging and efficient performance.
Checkout our other articles