Overcoming the One-Jar-to-Rule-Them-All Deployment Hurdle
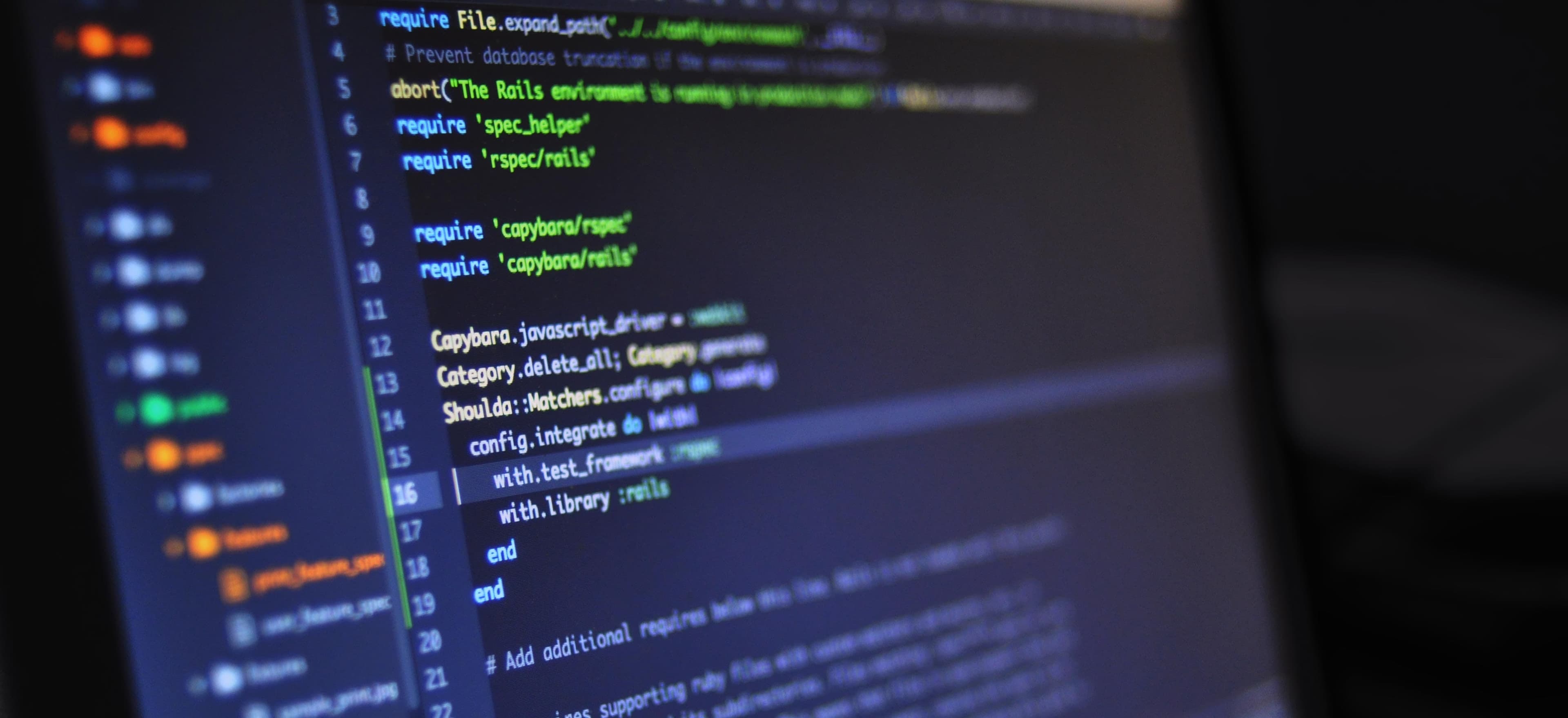
- Published on
Overcoming the One-Jar-to-Rule-Them-All Deployment Hurdle
As a Java developer, you must be familiar with the hassle of dealing with the "one JAR to rule them all" deployment challenge. This problem arises when a project has multiple dependencies, and managing the deployment becomes a nightmare because of the tangled web of JAR files. In this blog post, we will explore different strategies to overcome this deployment hurdle and streamline the deployment process using modern tools and techniques.
The "Fat" or "Uber" JAR Approach
One common approach to dealing with multiple dependencies in Java projects is to create a "fat" or "uber" JAR. This means bundling all the project's dependencies into a single JAR file, making it easier to distribute and run the application. While this approach solves the issue of managing multiple JAR files, it comes with its own set of challenges.
Pros of the "Fat" JAR approach:
- Simplifies deployment process
- Easier for end-users to run the application
- Self-contained and portable
Cons of the "Fat" JAR approach:
- Increased JAR size, which can lead to longer startup times
- Potential conflicts with dependencies
- Lack of modularity and reusability
// Example of creating a "Fat" JAR using Maven Shade Plugin
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<createDependencyReducedPom>true</createDependencyReducedPom>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
While the "Fat" JAR approach may be suitable for small projects or quick prototypes, it is not ideal for larger, more complex applications. Let's explore alternative strategies to tackle the deployment hurdle more efficiently.
Modularization with Java 9 and Beyond
With the introduction of modules in Java 9, developers now have a more structured way to manage dependencies and modularize their applications. By defining module-info.java and using the Java Platform Module System (JPMS), projects can explicitly declare their dependencies and encapsulate internal components.
Pros of modularization:
- Clear separation of concerns
- Explicit declaration of dependencies
- Better encapsulation and reusability
- Improved maintainability and scalability
Cons of modularization:
- Learning curve for developers new to modules
- Compatibility issues with existing libraries and frameworks
// Example of module descriptor (module-info.java)
module com.example.myapp {
requires transitive org.example.dependency;
exports com.example.myapp.package;
}
While modularization offers a more elegant solution to managing dependencies, migrating existing projects to modules can be a daunting task. However, the long-term benefits in terms of maintainability and scalability make it a worthwhile investment.
Dependency Management with Apache Maven
Apache Maven is a widely used build automation and project management tool for Java projects. It simplifies the build process and offers robust dependency management, making it easier to handle project dependencies and their transitive dependencies.
By defining dependencies in the project's pom.xml file, Maven resolves and downloads the necessary JAR files from remote repositories, saving developers from the hassle of manually managing JAR files.
Pros of using Apache Maven for dependency management:
- Centralized dependency configuration
- Automatic resolution of transitive dependencies
- Simplified project setup and build process
Cons of using Apache Maven for dependency management:
- Overhead of learning and configuring Maven for the project
- Potential conflicts and version resolution issues
<!-- Example of declaring a dependency in pom.xml -->
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>my-dependency</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
By leveraging Apache Maven for dependency management, developers can streamline the project setup and ensure that the required dependencies are readily available during the build process.
Gradle Build Tool for Dependency Management
Gradle is another popular build automation tool that has gained traction in the Java community. What sets Gradle apart from Maven is its focus on flexibility and the ability to write build scripts in Groovy or Kotlin. With its rich plugin ecosystem and support for incremental builds, Gradle offers an efficient approach to managing project dependencies.
Pros of using Gradle for dependency management:
- Flexible and expressive build scripts
- Incremental builds for improved performance
- Rich plugin ecosystem for extending functionality
Cons of using Gradle for dependency management:
- Higher learning curve, especially for developers new to Groovy or Kotlin
- Limited support for certain legacy systems and integrations
// Example of declaring a dependency in build.gradle
dependencies {
implementation 'org.example:my-dependency:1.0.0'
}
By utilizing Gradle's powerful build scripts and dependency management capabilities, developers can tailor the build process to their specific requirements and efficiently manage project dependencies.
To Wrap Things Up
Dealing with the "one JAR to rule them all" deployment hurdle in Java projects requires a strategic approach to managing dependencies. While the "fat" JAR approach may offer initial convenience, it is essential to explore more sustainable solutions such as modularization with Java 9 and beyond, robust dependency management with Apache Maven, or the flexibility of Gradle build tool.
By understanding the pros and cons of each approach and evaluating the specific needs of the project, developers can effectively overcome the deployment hurdle and streamline the deployment process. Embracing modern tools and techniques for managing dependencies not only simplifies the deployment process but also sets the stage for a more maintainable and scalable codebase.
Now that we have explored different strategies to overcome the "one JAR to rule them all" deployment challenge, it's time to apply these insights in our Java projects and witness the tangible benefits in terms of streamlined deployment and efficient management of dependencies.
Remember, the key to successful deployment lies in understanding your project's needs and choosing the right strategy to tackle the "one JAR to rule them all" deployment hurdle.
Happy coding!
Checkout our other articles