Unlocking AOP Magic in Java EE with Simple CDI Techniques
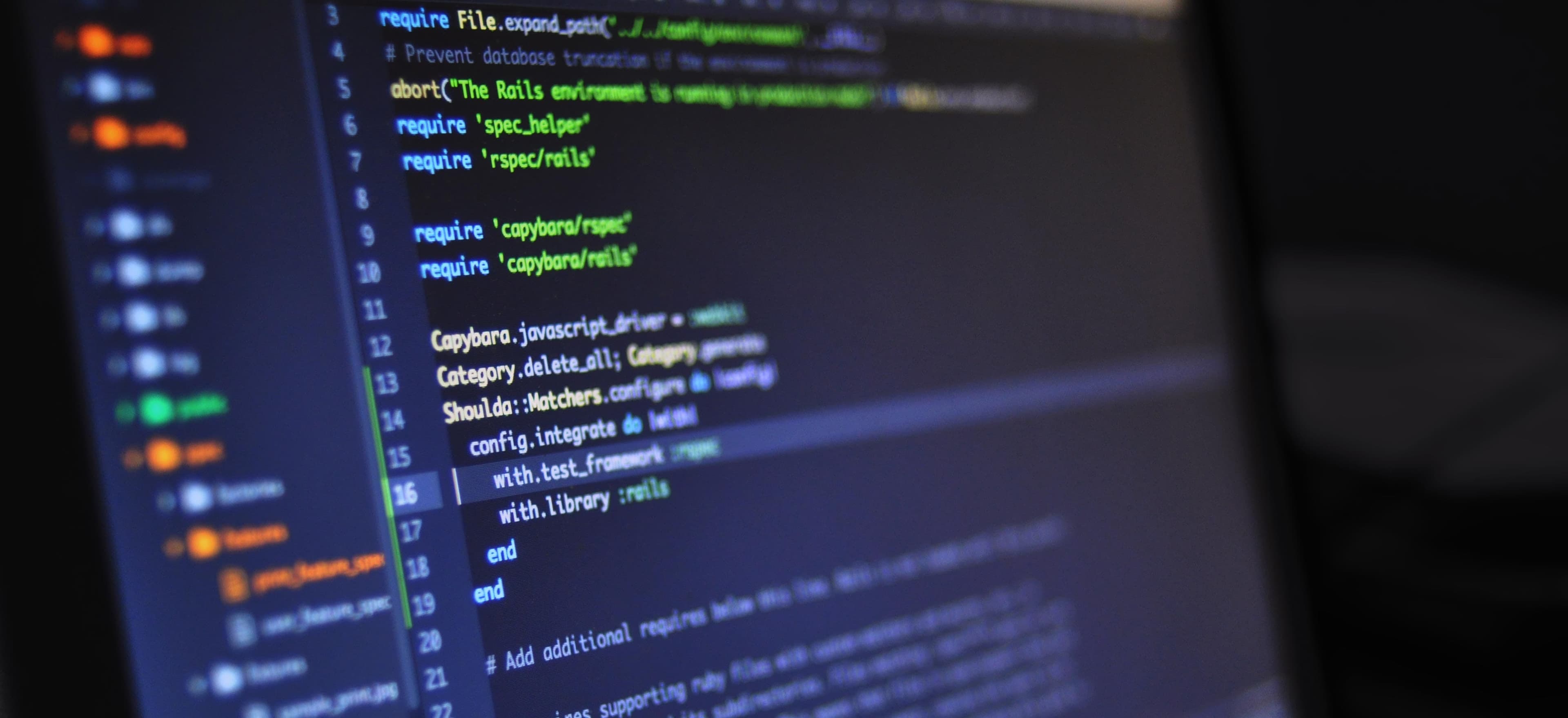
- Published on
Unlocking AOP Magic in Java EE with Simple CDI Techniques
In the world of Java EE development, Aspect-Oriented Programming (AOP) plays a crucial role in achieving modularity and separating cross-cutting concerns from the business logic. AOP allows developers to encapsulate cross-cutting concerns, such as logging, security, and transaction handling, into reusable modules, leading to cleaner and more maintainable code.
In this article, we will explore how to leverage the power of AOP in Java EE using simple Contexts and Dependency Injection (CDI) techniques. We'll delve into practical examples and code snippets to illustrate how CDI can be used to implement aspect-oriented behaviors in Java EE applications.
Understanding AOP and CDI
Before diving into the implementation details, let's briefly touch upon the concepts of AOP and CDI.
Aspect-Oriented Programming (AOP) is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. In Java, AOP is typically implemented using libraries such as AspectJ, which provides a way to define aspects and apply them to the existing codebase.
Contexts and Dependency Injection (CDI) is a powerful dependency injection framework introduced in Java EE 6. It provides a set of tools for creating decoupled and reusable components within a Java EE application.
Leveraging CDI for AOP
One of the key features of CDI is the ability to create interceptors and decorators, which can be used to implement AOP in Java EE applications. Let's explore how CDI allows us to achieve this.
Creating an Interceptor
Interceptors in CDI are used to intercept method calls and can be applied to multiple beans in a uniform manner. To create an interceptor, we can simply define a class and annotate it with @Interceptor
and @Priority
to specify the order in which interceptors should be invoked.
@Interceptor
@Priority(Interceptor.Priority.APPLICATION)
public class LoggingInterceptor {
@AroundInvoke
public Object logMethodInvocation(InvocationContext context) throws Exception {
// Perform logging before method invocation
System.out.println("Entering method: " + context.getMethod().getName());
try {
// Proceed with the method invocation
return context.proceed();
} finally {
// Perform logging after method invocation
System.out.println("Exiting method: " + context.getMethod().getName());
}
}
}
In the above code snippet, we create a simple logging interceptor that logs the method invocation before and after the actual method execution.
Applying Interceptors to Beans
Once the interceptor is defined, we can easily apply it to any CDI-managed bean by simply annotating the target method or class with @Interceptors
.
@Stateless
@Interceptors(LoggingInterceptor.class)
public class ProductService {
public void getProductDetails(int productId) {
// Business logic for retrieving product details
}
public void updateProduct(Product product) {
// Business logic for updating product
}
}
In this example, the LoggingInterceptor
will be applied to all methods within the ProductService
bean, allowing us to log method invocations without cluttering the business logic of the bean.
Declaring Interceptor Binding
In addition to applying interceptors directly to beans, CDI allows us to create custom interceptor bindings, providing a more fine-grained control over which methods or classes should be intercepted.
@Inherited
@InterceptorBinding
@Target({ ElementType.METHOD, ElementType.TYPE })
@Retention(RetentionPolicy.RUNTIME)
public @interface Audited {
}
By defining a custom interceptor binding like the Audited
annotation above, we can specify that only methods or classes annotated with @Audited
should be intercepted by the associated interceptors.
@Stateless
public class OrderService {
@Audited
public void placeOrder(Order order) {
// Business logic for placing an order
}
public void cancelOrder(int orderId) {
// Business logic for canceling an order
}
}
By annotating the placeOrder
method with @Audited
, we can now selectively apply interceptors based on custom criteria.
Bringing It All Together
In this article, we have explored how Contexts and Dependency Injection (CDI) can be leveraged to implement Aspect-Oriented Programming (AOP) in Java EE applications. We have discussed the creation of interceptors, their application to beans, and the declaration of custom interceptor bindings, showcasing the power of CDI in achieving modularity and separation of concerns.
By incorporating AOP practices into Java EE development using CDI, developers can enhance the maintainability and reusability of their code while keeping the business logic clean and focused. This approach also promotes the effective separation of concerns, making the codebase more resilient to changes and easier to maintain in the long run.
Click here to explore more about AOP in Java.
In conclusion, CDI provides a simple yet powerful way to unlock the magic of AOP in Java EE, offering developers the tools they need to build scalable and maintainable applications.
Give it a try, and start weaving some AOP magic into your Java EE projects with CDI!