Solving the Mystery of Reflection Selector Expressions
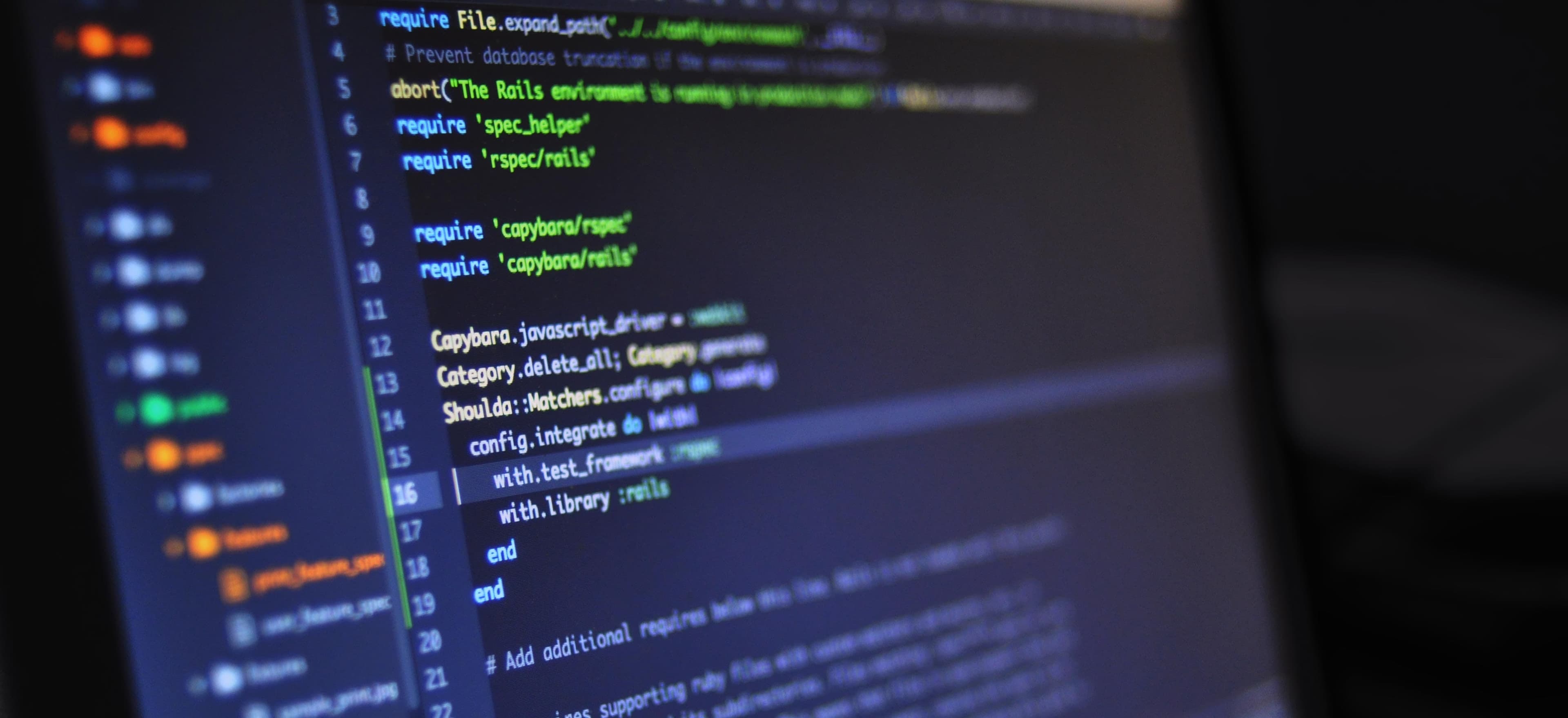
- Published on
Solving the Mystery of Reflection Selector Expressions
Reflection is a powerful feature in Java that allows a program to examine or modify its own structure at runtime. While reflection is a highly advanced concept, one specific aspect called "reflection selector expressions" often puzzles developers. In this article, we will unravel the mystery of reflection selector expressions, understand their purpose, and learn to use them effectively.
Understanding Reflection Selector Expressions
In Java, a reflection selector expression is a string that represents a field or method in a class. It is commonly used with the java.lang.reflect
package to dynamically access fields and methods of a class. Reflection selector expressions are primarily used in scenarios where the field or method name is not known at compile time, and the program needs to dynamically determine and access them at runtime.
Let's dive into an example to understand reflection selector expressions more clearly. Consider a class Person
with fields name
and age
. We can use reflection selector expressions to access and modify these fields at runtime.
public class Person {
private String name;
private int age;
// Getters and setters omitted for brevity
}
Using Reflection Selector Expressions
To explore the capabilities of reflection selector expressions, let's create a simple Java program that demonstrates their usage.
import java.lang.reflect.Field;
public class ReflectionDemo {
public static void main(String[] args) throws NoSuchFieldException, IllegalAccessException {
Person person = new Person();
// Accessing field using reflection selector expression
Field nameField = Person.class.getDeclaredField("name");
nameField.setAccessible(true);
nameField.set(person, "John Doe");
System.out.println("Name: " + person.getName());
}
}
In the code above, we use the Field.getDeclaredField(String name)
method to obtain a reference to the name
field of the Person
class using a reflection selector expression. Once we have the field, we can set its accessibility and update its value using Field.set(Object obj, Object value)
. This allows us to modify the name
field of the Person
object dynamically.
Advantages of Reflection Selector Expressions
Reflection selector expressions offer several benefits:
-
Dynamic Access: Reflection selector expressions enable dynamic access to fields and methods, allowing the program to adapt to changing requirements at runtime.
-
Decoupling: They help decouple the code by allowing interactions with classes and their members without having explicit compile-time dependencies.
-
Flexibility: Reflection makes it possible to create more generic and reusable code by working with classes and members in a dynamic manner.
Best Practices for Using Reflection Selector Expressions
While reflection selector expressions provide powerful capabilities, they come with a few caveats and best practices to keep in mind:
-
Performance Overhead: Reflection operations are usually slower than their non-reflection counterparts. Use them judiciously in performance-critical sections of the code.
-
Security Considerations: Reflection can bypass access control, so it's essential to use it with caution, especially in security-sensitive applications.
-
Error Handling: When using reflection, handle potential exceptions such as
NoSuchFieldException
andIllegalAccessException
to ensure graceful degradation in case of failures.
A Final Look
In this article, we demystified reflection selector expressions in Java and explored how they can be used to dynamically interact with class fields and methods at runtime. While reflection is a powerful tool, it should be used thoughtfully and judiciously due to its potential performance and security implications. By understanding reflection selector expressions and adhering to best practices, developers can harness the full potential of reflection while building robust and flexible Java applications.
Reflection selector expressions truly bring a new level of dynamism to Java, enabling developers to write adaptable and flexible code that can respond to changing requirements with ease. Embracing reflection intelligently can lead to more modular, reusable, and extensible Java applications while ensuring performance and security considerations are met.
Reflect on the power of reflection selector expressions and unlock the true potential of dynamic programming in Java!