Achieving Perfect Test Isolation with JUnit: The How-To Guide
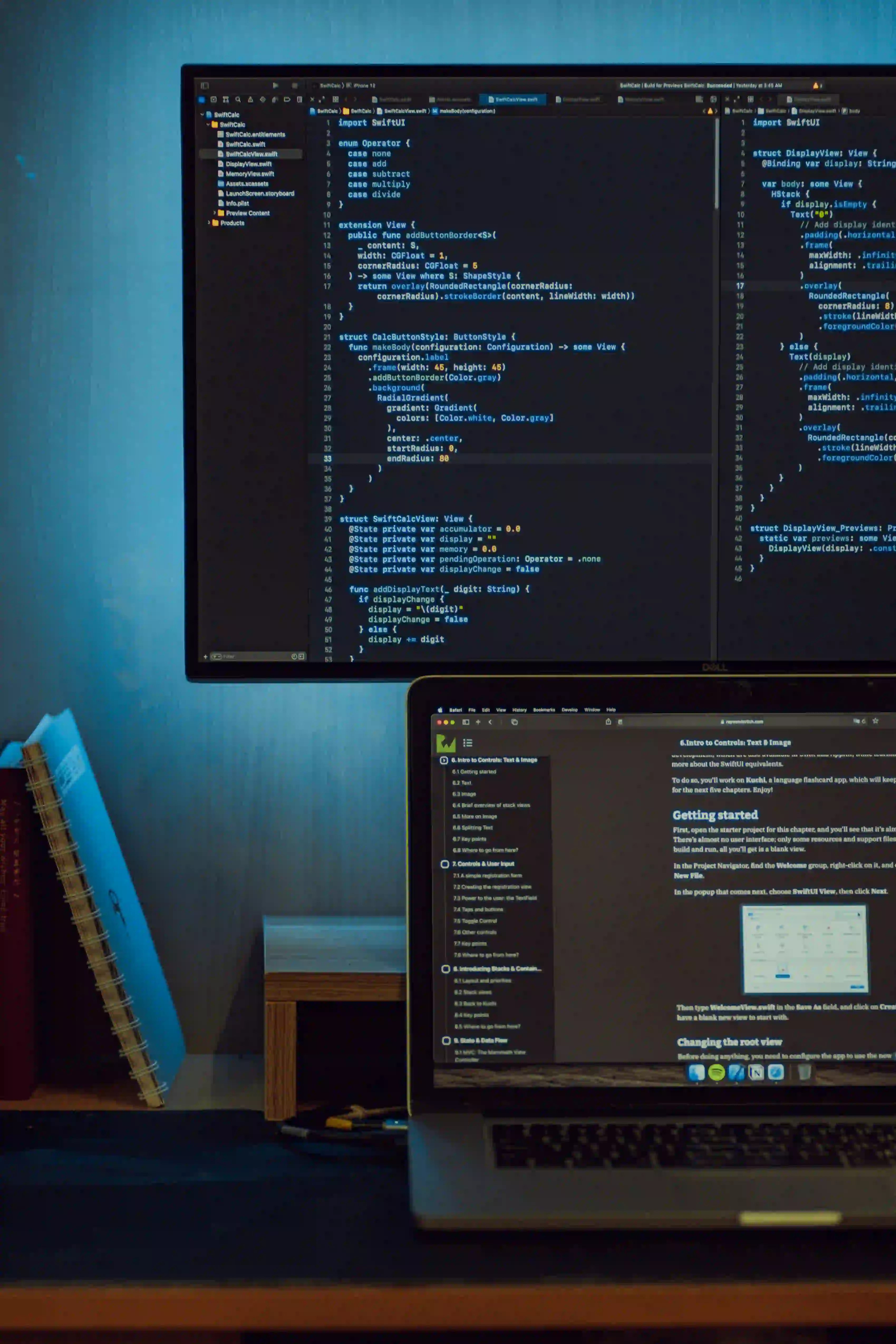
Achieving Perfect Test Isolation with JUnit: The How-To Guide
Testing is an important aspect of the software development process. In Java, JUnit is one of the most popular testing frameworks. As developers, we strive to write tests that are independent of each other, ensuring perfect isolation. This is crucial to avoid unexpected interactions between tests and to make debugging easier. In this guide, we'll explore how to achieve perfect test isolation using JUnit.
What is Test Isolation?
Test isolation means that each test should run independently of the others. This ensures that the outcome of one test does not affect the outcome of another. Achieving test isolation is important for building reliable and maintainable test suites.
Understanding the Importance of Test Isolation
Test isolation is crucial for the following reasons:
-
Predictable Testing: Isolated tests allow for predictable results, making it easier to diagnose and fix issues.
-
Maintenance: When tests are isolated, it's easier to maintain and update them without causing unintended side effects on other tests.
-
Parallel Execution: Isolated tests can be run in parallel, improving the overall test suite's efficiency.
Achieving Test Isolation with JUnit
JUnit provides several mechanisms to achieve test isolation. Let's explore some of the best practices.
Using @Before
and @After
Annotations
The @Before
and @After
annotations in JUnit allow us to execute setup and teardown code before and after each test method. This approach ensures that each test runs in isolation by resetting the state before and after execution.
public class MyTest {
private MyClass myClass;
@Before
public void setUp() {
myClass = new MyClass();
// Additional setup code
}
@Test
public void testMethod1() {
// Test method 1
}
@Test
public void testMethod2() {
// Test method 2
}
@After
public void tearDown() {
// Teardown code
}
}
Why: The @Before
and @After
annotations ensure that each test method starts with a clean and consistent state, independent of other test methods.
Utilizing @BeforeEach
and @AfterEach
in JUnit 5
In JUnit 5, the @BeforeEach
and @AfterEach
annotations serve the same purpose as @Before
and @After
in JUnit 4, respectively. These annotations help maintain test isolation by setting up and tearing down the test environment before and after each test method execution.
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.Test;
public class MyTest {
private MyClass myClass;
@BeforeEach
public void setUp() {
myClass = new MyClass();
// Additional setup code
}
@Test
public void testMethod1() {
// Test method 1
}
@Test
public void testMethod2() {
// Test method 2
}
@AfterEach
public void tearDown() {
// Teardown code
}
}
Why: Leveraging @BeforeEach
and @AfterEach
in JUnit 5 ensures that each test method runs in isolation and maintains a clean state without interference from other tests.
Employing Mocking Frameworks for External Dependencies
When a class being tested has external dependencies, such as database connections or network calls, mocking these dependencies becomes essential to achieve test isolation. Frameworks like Mockito and PowerMock enable mocking these external dependencies, ensuring that each test focuses solely on the code being tested.
// Using Mockito for mocking external dependencies
import org.junit.jupiter.api.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.junit.jupiter.MockitoExtension;
import org.junit.jupiter.api.extension.ExtendWith;
@ExtendWith(MockitoExtension.class)
public class MyTest {
@InjectMocks
private MyClass myClass;
@Mock
private ExternalDependency externalDependency;
@Test
public void testMethod1() {
// Define mock behaviors
when(externalDependency.someMethod()).thenReturn(someValue);
// Test method 1
}
// Additional test methods
}
Why: Using mocking frameworks allows us to isolate the code under test by replacing external dependencies with controlled mock objects, ensuring test independence.
Employing Parameterized Tests
JUnit's parameterized tests allow us to run the same test with different inputs. This can contribute to test isolation by conducting multiple test cases within the same test method, instead of having multiple test methods for similar scenarios.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
public class ParameterizedTestExample {
@ParameterizedTest
@ValueSource(ints = { 1, 2, 3 })
public void testMethod(int value) {
// Test with different input values
}
}
Why: Parameterized tests enable us to test the same behavior with different inputs within a single test method, promoting test isolation and reducing redundancy.
Wrapping Up
Achieving perfect test isolation with JUnit is crucial for building reliable and maintainable test suites. By utilizing setup and teardown methods, leveraging mocking frameworks, and employing parameterized tests, developers can ensure that each test runs independently and produces consistent results.
In conclusion, mastering test isolation with JUnit empowers developers to build robust and reliable test suites, contributing to overall software quality and maintainability.
Remember, perfect test isolation is not just an aspiration – it's an achievable goal with the right techniques and best practices in place. With JUnit as our ally, we can conquer the challenge of test isolation and elevate the quality of our software through comprehensive, reliable testing.