System.gc() Demystified: When & How to Use It Effectively
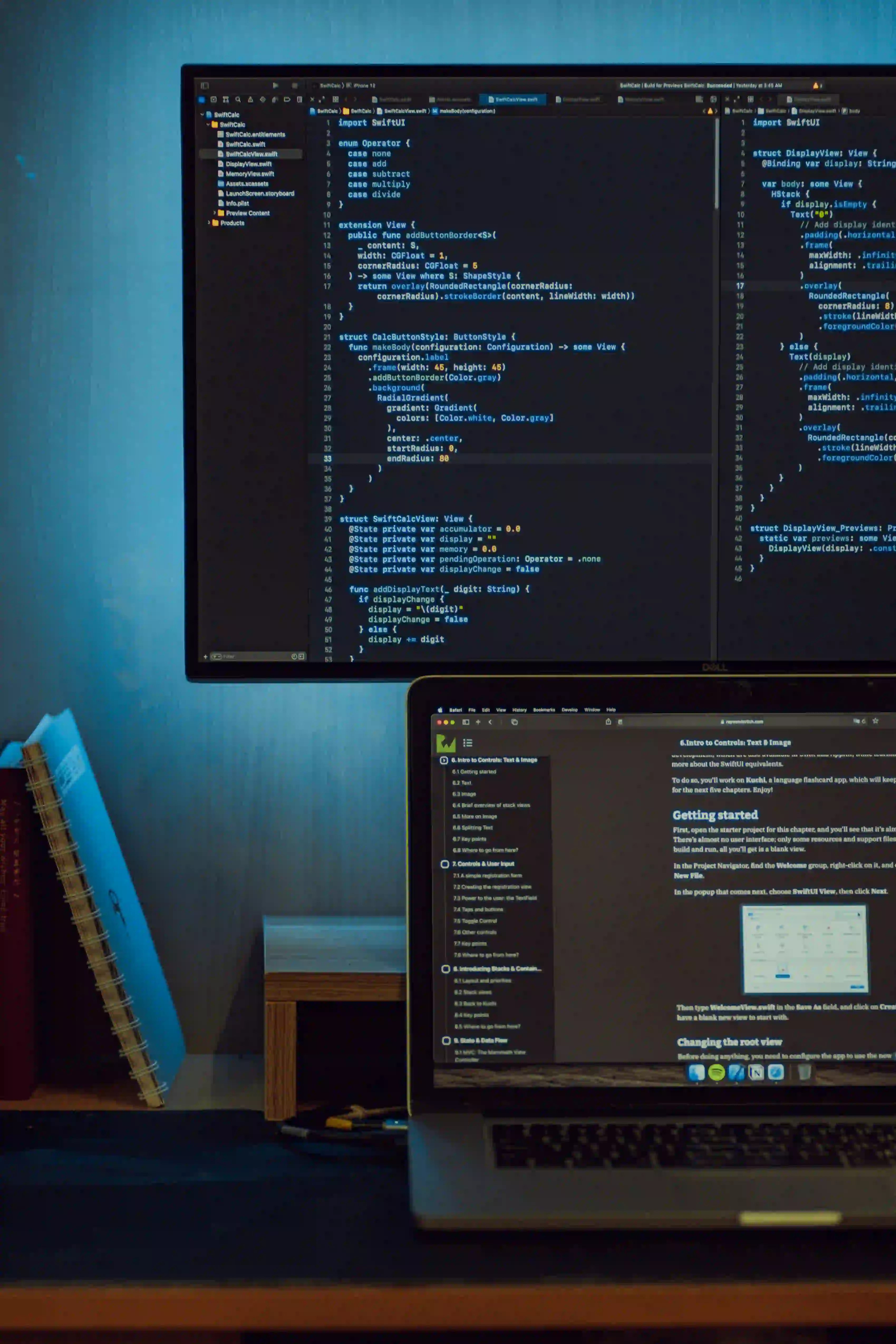
System.gc() Demystified: When & How to Use It Effectively
Garbage Collection (GC) is an integral aspect of memory management in Java, responsible for reclaiming memory occupied by objects that are no longer in use. The System.gc()
method serves as a way to suggest to the JVM that it should perform garbage collection, but its usage is often a point of contention within the Java community.
In this post, we will delve into the nuances of System.gc()
, its implications, and provide insights into when and how to use it effectively in your Java applications.
Understanding System.gc()
First and foremost, it's essential to understand that System.gc()
is merely a hint to the Java Virtual Machine (JVM) to trigger garbage collection. It does not enforce immediate garbage collection, nor does it guarantee that GC will occur at all. The decision to perform garbage collection ultimately rests with the JVM, which employs its own heuristics and algorithms to manage memory.
When to Use System.gc()
So, when should you consider incorporating System.gc()
into your Java code? In most scenarios, the best practice dictates that explicit calls to System.gc()
should be avoided. The JVM is adept at managing memory and triggering garbage collection when necessary, negating the need for manual intervention in the majority of cases.
Exceptions to the Rule
However, there are specific cases where using System.gc()
can be deemed as appropriate:
-
Benchmarking and Profiling: When conducting performance analysis or memory profiling,
System.gc()
can be strategically utilized to minimize the impact of existing garbage on the results. -
Resource-Constrained Environments: In scenarios where the application runs within resource-constrained environments, such as mobile or embedded systems, invoking
System.gc()
at opportune moments can assist in managing memory efficiently. -
Custom Java Virtual Machine Implementations: For developers working on custom JVM implementations or specialized environments, judicious use of
System.gc()
might be necessary to fine-tune memory management.
How to Use System.gc() Effectively
Should the need arise, it's crucial to employ System.gc()
judiciously and with a clear understanding of its implications. Here are some guidelines for effective usage:
Limit the Frequency
Avoid incorporating System.gc()
into critical code paths or invoking it at regular intervals. Overusing System.gc()
can lead to suboptimal performance and may disrupt the JVM's natural memory management rhythm.
Context-Aware Invocation
Employ System.gc()
in specific sections of your code where the repercussions of garbage collection can be minimized. For instance, triggering garbage collection at the end of a task or before a memory-intensive operation can help mitigate its impact on the application's performance.
Monitor and Analyze
Before resorting to System.gc()
, it's imperative to analyze the memory usage and behavior of your application using profilers or monitoring tools. By gaining insights into memory patterns and hotspots, you can make informed decisions about when and where to incorporate garbage collection hints.
Measure the Impact
When utilizing System.gc()
for performance optimization or memory management, it's essential to measure its impact. Conduct thorough benchmarking and profiling to ascertain whether the explicit invocation of garbage collection yields tangible benefits or introduces overhead.
Best Practices for Memory Management
While System.gc()
can serve as a makeshift solution in specific scenarios, it should not be misconstrued as a blanket remedy for memory management challenges in Java applications. Adhering to best practices for memory management is fundamental to ensuring optimal performance and resource utilization:
Proper Resource Handling
Adopt robust practices for resource handling, including explicit resource release, nullifying object references, and employing try-with-resources for managing external resources.
try (FileInputStream fileInputStream = new FileInputStream("file.txt")) {
// Process the file
} catch (IOException e) {
// Handle the exception
}
Memory Profiling and Analysis
Leverage tools such as VisualVM, Java Mission Control, or YourKit to conduct comprehensive memory profiling and analysis. Identifying memory leaks, inefficient object creation, and unnecessary retention of objects can aid in optimizing memory usage without resorting to explicit garbage collection hints.
Tuning Garbage Collection
Instead of relying on manual garbage collection invocations, focus on tuning the JVM's garbage collection behavior using appropriate command-line options and flags, such as -Xmx
, -Xms
, -XX:NewRatio
, and -XX:MaxGCPauseMillis
.
Performance Optimization
Prioritize performance optimization techniques, including caching, object pooling, and efficient data structures, to minimize the generation of short-lived objects and reduce the strain on the garbage collector.
Closing the Chapter
In conclusion, System.gc()
should be approached with caution and used sparingly in targeted scenarios where its benefits outweigh potential drawbacks. Overreliance on explicit garbage collection calls can undermine the JVM's intrinsic memory management capabilities and lead to suboptimal performance.
By adhering to best practices, leveraging monitoring tools, and embracing sound memory management principles, developers can curtail the necessity of manual garbage collection hints and foster efficient, sustainable memory utilization within their Java applications.
Remember, Java's garbage collection mechanism is sophisticated and proficient in managing memory autonomously. Trust in its capabilities, intervene judiciously, and let the JVM orchestrate garbage collection in harmony with your application's needs.