Avoiding Type Errors in Java 8 Dependency Injection
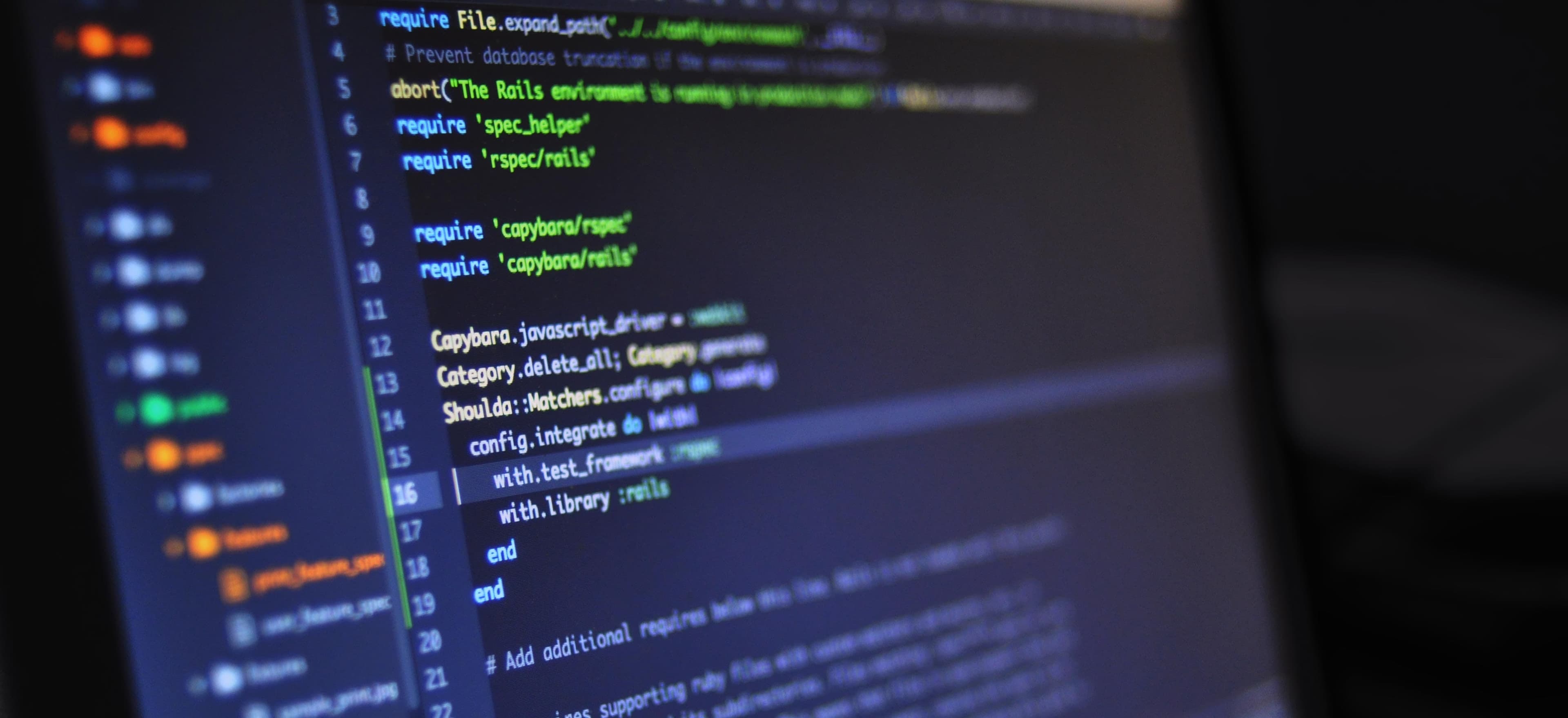
- Published on
Understanding Type Errors in Java 8 Dependency Injection
In the world of Java programming, dependency injection plays a crucial role in ensuring loose coupling and better code maintainability. However, with the benefits come certain challenges, particularly with type errors. Type errors can occur when the type requested does not match the type provided, resulting in runtime failures. In this blog post, we will explore how to mitigate type errors in Java 8 dependency injection through clear examples and best practices.
Understanding Dependency Injection
Before diving into type errors, let's first grasp the concept of dependency injection. In Java, dependency injection is a technique that provides objects with their dependencies rather than having the objects create or find their dependencies. This approach decouples the usage of an object from its creation, making the code more modular and easier to maintain.
Type Errors in Dependency Injection
Type errors in dependency injection can occur when the expected type of a dependency does not match the actual type provided during injection. This can lead to runtime failures, making it crucial to address these type discrepancies to ensure the robustness of the codebase.
Let's consider an example where a type error can manifest:
public class UserService {
private final UserRepository userRepository;
@Inject
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Other methods omitted for brevity
}
In the above snippet, UserService
expects a UserRepository
as a dependency. However, if during the injection process a different type is provided, it can lead to type errors at runtime.
Mitigating Type Errors
1. Using Generics
One effective approach to avoid type errors in dependency injection is by leveraging generics. By using generics, you can define the type of the injected dependency, providing compile-time safety and reducing the likelihood of runtime type errors.
public class UserService<T extends UserRepository> {
private final T userRepository;
@Inject
public UserService(T userRepository) {
this.userRepository = userRepository;
}
// Other methods omitted for brevity
}
By using generics, the UserService
class ensures that only subclasses of UserRepository
can be injected, mitigating potential type errors.
2. Utilizing Interface-based Injection
Another approach to minimize type errors is by utilizing interfaces for dependency injection. By defining interfaces for dependencies, you can ensure that the injected classes adhere to a specific contract, reducing the risk of incompatible types.
public interface UserRepository {
// Method signatures
}
public class UserService {
private final UserRepository userRepository;
@Inject
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Other methods omitted for brevity
}
In the above example, UserRepository
is an interface, and the UserService
class depends on the interface rather than a concrete implementation. This allows for flexibility in the injected classes while maintaining type safety.
3. Employing Qualifiers
Java 8 introduced the concept of qualifiers, which can be used to disambiguate between multiple beans of the same type. By leveraging qualifiers, you can specify the exact bean to be injected, thus preventing type errors arising from ambiguous dependencies.
public class UserService {
private final UserRepository primaryUserRepository;
private final UserRepository secondaryUserRepository;
@Inject
public UserService(@Primary UserRepository primaryUserRepository, @Secondary UserRepository secondaryUserRepository) {
this.primaryUserRepository = primaryUserRepository;
this.secondaryUserRepository = secondaryUserRepository;
}
// Other methods omitted for brevity
}
In the above snippet, @Primary
and @Secondary
are qualifiers used to differentiate between multiple UserRepository
implementations. This ensures that the correct type of UserRepository
is injected, minimizing type errors.
4. Constructor-based Injection
Employing constructor-based injection can also aid in preventing type errors. By explicitly defining dependencies through the constructor, any mismatch in types becomes evident at compile time, reducing the likelihood of runtime type errors.
public class UserService {
private final UserRepository userRepository;
@Inject
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Other methods omitted for brevity
}
In the above example, the UserService
class uses constructor-based injection, explicitly declaring its dependencies. This approach allows for clear visibility of the injected types, aiding in early detection of type discrepancies.
Final Thoughts
Type errors in Java 8 dependency injection can introduce runtime failures and adversely impact the stability of a codebase. By employing techniques such as generics, interface-based injection, qualifiers, and constructor-based injection, developers can mitigate type errors and ensure the robustness of their code. It is essential to leverage these best practices to foster a reliable and maintainable codebase when working with dependency injection in Java.
In conclusion, addressing type errors in Java 8 dependency injection not only enhances the overall quality of the code but also contributes to a more resilient and manageable codebase.
For further reading on Java 8 dependency injection and type safety, refer to the official Java Documentation and Dependency Injection in Java guide by Baeldung.
Happy coding!
Checkout our other articles