Cracking Coplien's Code: Rethinking Unit Testing Efficacy
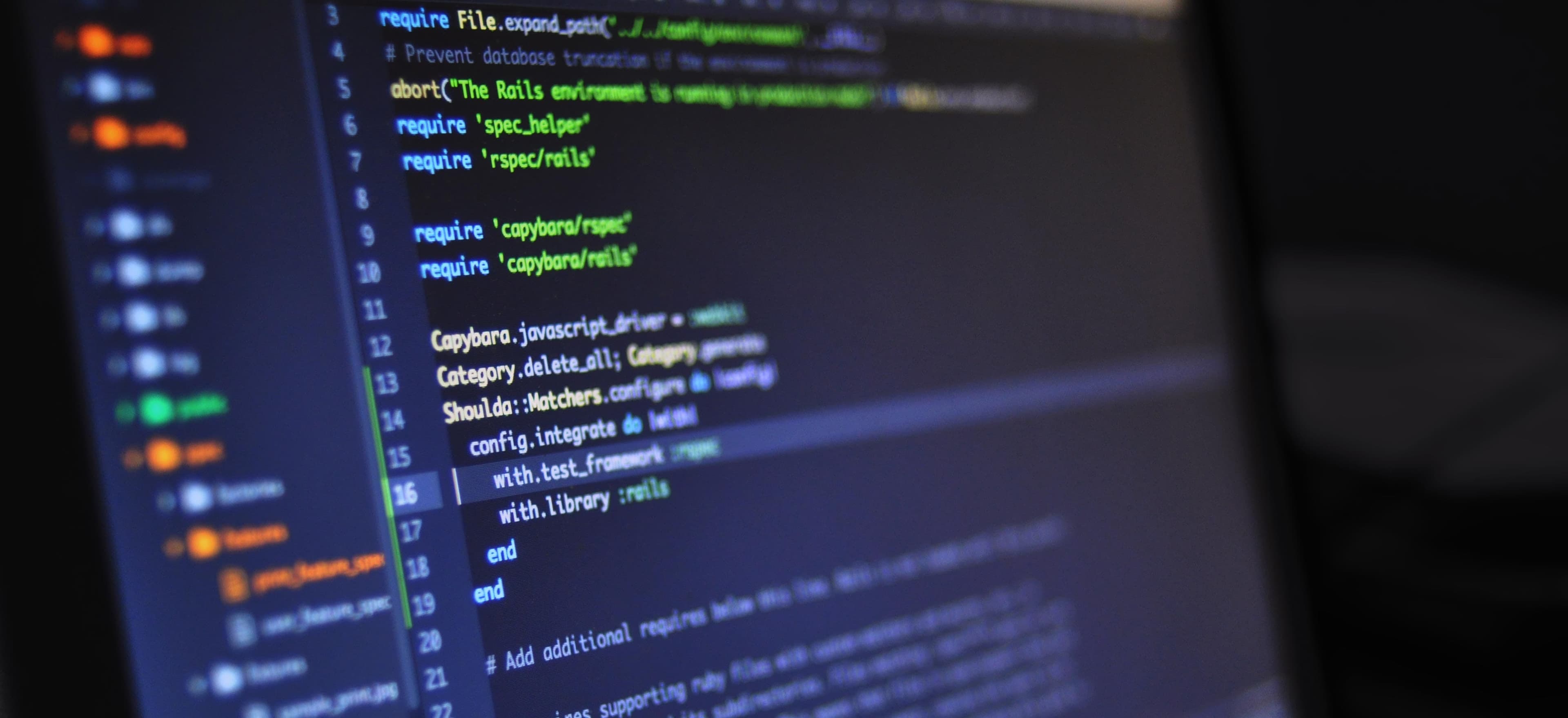
- Published on
Cracking Coplien's Code: Rethinking Unit Testing Efficacy
Diving Into the Subject
Coplien's Law states that a "test is only as good as the designer of the test." This raises an important issue: How effective are unit tests in Java projects, and are they truly ensuring the reliability and robustness of our code? In this blog post, we will delve into the concept of unit testing in Java, explore its pros and cons, and discuss effective strategies to create valuable unit tests that uphold the integrity of our codebase.
At the heart of any Java project lies the crucial chore of unit testing. While writing production code is undeniably important, ensuring that the code works as expected and continues to do so in the face of changes is equally essential. Unit testing serves as the vanguard in this noble battle – defending against regressions and providing the confidence to refactor and modify code with a safety net.
Defining Unit Testing
Unit testing is a software testing method where individual units or components of a software are tested to verify that each unit works as designed. In Java, unit tests are commonly written using frameworks such as JUnit or TestNG, both of which provide annotations for defining test methods and assertions for validating expected outcomes. Let's take a simple example to illustrate the essence of unit testing:
public class MathUtils {
public int add(int a, int b) {
return a + b;
}
}
Now, let's write a corresponding JUnit test:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
int result = mathUtils.add(3, 5);
assertEquals(8, result);
}
}
In this example, we have a simple MathUtils
class with an add
method and a corresponding JUnit test to verify the functionality of the add
method.
The Pros and Cons of Unit Testing
Pros
-
Early Bug Detection: Unit tests can detect bugs at an early stage of development, thereby reducing the cost and effort required for fixing them later.
-
Documentation: Well-written unit tests can serve as a form of documentation, illustrating the expected behavior of the code.
-
Refactoring Confidence: Having a comprehensive suite of unit tests allows developers to refactor their code with confidence, knowing that any breaking changes will be caught by the tests.
Cons
-
Maintenance Overhead: Writing and maintaining unit tests requires additional effort and time, which can be seen as overhead, especially in agile environments where rapid development is emphasized.
-
False Sense of Security: A suite of passing unit tests does not guarantee bug-free code. It can provide a false sense of security if the tests are not thorough or are poorly designed.
-
Testing Private Methods: Unit testing private methods can be challenging and sometimes impractical. This can lead to incomplete test coverage and a false sense of security.
Rethinking Unit Testing Efficacy
Test Behavior, Not Implementation
Unit tests should focus on the behavior of the code, not its implementation details. This approach, known as behavior-driven development (BDD), emphasizes writing tests in a human-readable format that describes the expected behavior of the code. By adhering to BDD principles, tests become more resilient to changes in the codebase, as they are not tightly coupled to the internal implementation.
For example, instead of testing an internal method like this:
@Test
public void testCalculateTax() {
TaxCalculator taxCalculator = new TaxCalculator();
double result = taxCalculator.calculateTax(1000);
assertEquals(100, result);
}
We should focus on the behavior of the method:
@Test
public void testCalculateTax() {
double result = new TaxCalculator().calculateTax(1000);
assertEquals(100, result);
}
By focusing on the behavior of the calculateTax
method rather than the instantiation of the TaxCalculator
class, the test becomes more resilient to changes in the codebase.
Embrace Test-Driven Development (TDD)
Test-driven development (TDD) enforces writing tests before writing the actual production code. This approach not only ensures test coverage from the outset but also prompts the developer to design code that is testable. By following TDD, developers can foster a culture of creating reliable, well-tested code, leading to better software quality in the long run.
Leverage Mocking and Stubbing
When testing a specific unit, it's often necessary to isolate it from other dependencies. This is where mocking and stubbing come into play. Frameworks like Mockito provide elegant solutions for creating mock objects and stubbing method calls, allowing developers to focus solely on the behavior of the unit under test.
An example using Mockito to mock an external dependency in a unit test:
@Test
public void testGetUserById() {
UserService userService = Mockito.mock(UserService.class);
Mockito.when(userService.getUserById(1L)).thenReturn(new User("John Doe"));
UserManager userManager = new UserManager(userService);
User user = userManager.getUserById(1L);
assertEquals("John Doe", user.getName());
}
Here, we have mocked the UserService
dependency to ensure that the getUserById
method returns a specific User
object, allowing us to focus on testing the behavior of the UserManager
class.
Continuous Integration Testing
Integrating unit tests into a continuous integration (CI) pipeline ensures that every code change is accompanied by a suite of tests. This practice not only prevents the introduction of regressions but also fosters a culture of quality and assurance within the development team. Jenkins and Travis CI are popular choices for setting up CI pipelines with robust support for Java projects.
To Wrap Things Up
In conclusion, unit testing in Java is a critical aspect of software development, but its efficacy is heavily dependent on the approach and care invested in creating the tests. By rethinking traditional unit testing practices, embracing behavior-driven development, test-driven development, and leveraging advanced testing techniques, developers can elevate the reliability and robustness of their Java codebases. Remember, the true essence of unit testing lies in crafting tests that truly validate the correctness and behavior of the code, rather than merely its existence.
Unit tests are the unsung heroes of a robust software system, and it's time to let them shine.
Join the conversation on Coplien's Law and JUnit testing to further enrich your understanding of unit testing.
Checkout our other articles