Capturing Frames from Video with Xuggler: A How-To Guide
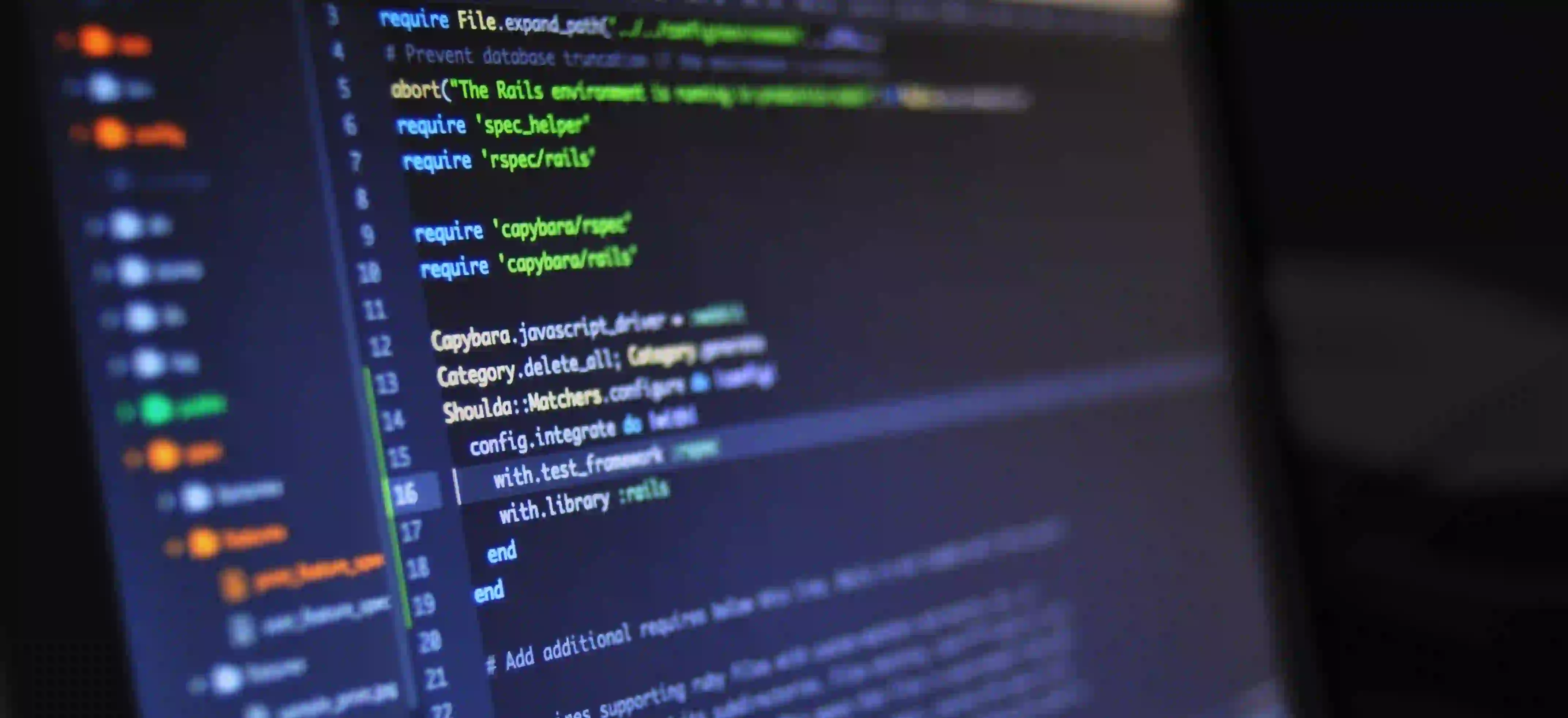
Capturing Frames from Video with Xuggler: A How-To Guide
In the realm of multimedia processing with Java, Xuggler stands tall as a powerful and versatile tool. Whether you're a seasoned developer or just dipping your toes into multimedia manipulation, understanding how to capture individual frames from a video using Xuggler is a valuable skill. In this guide, we'll walk through the process step by step, providing code examples and explanations along the way.
What is Xuggler?
Xuggler is an open-source multimedia framework for Java that provides a set of APIs for decoding, encoding, and manipulating audio and video. It offers a simple and powerful way to work with multimedia data in your Java applications. With Xuggler, you can perform tasks such as reading video files, capturing frames, modifying audio streams, and much more.
Prerequisites
Before diving into the code, make sure you have the following prerequisites in place:
- Java Development Kit (JDK): Ensure that you have Java SE Development Kit installed on your system.
- Xuggler Library: Download the Xuggler library from the official website or include it as a dependency in your build tool (Maven, Gradle, etc.).
Capturing Frames from Video
Now, let's walk through the process of capturing frames from a video using Xuggler. We'll start by setting up the necessary dependencies and then dive into the code.
Setting Up Dependencies
If you're using Maven, you can include the Xuggler dependency in your pom.xml
as follows:
<dependencies>
<dependency>
<groupId>com.xuggle</groupId>
<artifactId>xuggle-xuggler</artifactId>
<version>5.4</version>
</dependency>
</dependencies>
For Gradle, add the following to your build.gradle
:
dependencies {
implementation 'com.xuggle:xuggle-xuggler:5.4'
}
Capturing Frames
To capture frames from a video using Xuggler, follow these steps:
-
Create a MediaReader: Create an instance of
IMediaReader
to read the video file.☕snippet.javaIMediaReader mediaReader = ToolFactory.makeReader("path_to_video_file");
Make sure to replace
"path_to_video_file"
with the actual path to your video file. -
Set Up Frame Listener: Create a
MediaListenerAdapter
to handle the captured frames.☕snippet.javamediaReader.setBufferedImageTypeToGenerate(BufferedImage.TYPE_3BYTE_BGR); mediaReader.addListener(new MediaListenerAdapter() { @Override public void onVideoPicture(IVideoPictureEvent event) { // Process the captured frame (event.getImage()). } });
In the
onVideoPicture
method, you can perform operations on the captured frame such as saving it to a file, processing it, or displaying it. -
Read and Process Frames: Start reading the video and processing the frames.
☕snippet.javawhile (mediaReader.readPacket() == null) { // Continue reading and processing frames. }
This loop continuously reads packets from the video and triggers the
onVideoPicture
method for each captured frame. -
Close the MediaReader: Once done, close the media reader to release system resources.
☕snippet.javamediaReader.close();
-
Complete Code Example:
☕snippet.javaimport com.xuggle.mediatool.IMediaReader; import com.xuggle.mediatool.ToolFactory; import com.xuggle.xuggler.IVideoPictureEvent; import com.xuggle.xuggler.IVideoResampler; import com.xuggle.xuggler.demos.VideoImage; import com.xuggle.xuggler.Global; import com.xuggle.xuggler.ICodec; import com.xuggle.xuggler.IPacket; import com.xuggle.xuggler.IPixelFormat; import java.awt.image.BufferedImage; import java.util.concurrent.TimeUnit; public class VideoFrameCapture { public static void main(String[] args) { final IMediaReader mediaReader = ToolFactory.makeReader("path_to_video_file"); mediaReader.setBufferedImageTypeToGenerate(BufferedImage.TYPE_3BYTE_BGR); mediaReader.addListener(new VideoImage()); while (mediaReader.readPacket() == null) { // Continue reading and processing frames. } mediaReader.close(); } public static class VideoImage extends MediaListenerAdapter { public void onVideoPicture(IVideoPictureEvent event) { try { // Convert the frame to a BufferedImage BufferedImage image = Utils.videoPictureToImage(event.getImage()); // Perform operations on the captured frame } catch (Exception e) { e.printStackTrace(); } } } public static class Utils { public static BufferedImage videoPictureToImage(IVideoPicture frame) { IVideoPicture newPic = IVideoPicture.make( frame.getPixelType(), frame.getWidth(), frame.getHeight() ); if (resampler == null) { resampler = IVideoResampler.make( newPic.getWidth(), newPic.getHeight(), IPixelFormat.Type.BGR24, frame.getWidth(), frame.getHeight(), frame.getPixelType() ); if (resampler == null) { throw new RuntimeException("Could not create color space resampler for: " + filename); } } if (resampler.resample(newPic, frame) >= 0) { BufferedImage javaImage = Utils.videoPictureToImage(newPic); return javaImage; } } } }
Final Thoughts
Capturing frames from a video using Xuggler opens up a world of possibilities for multimedia processing in Java. Whether you're building a video editing application, analyzing video content, or implementing computer vision algorithms, the ability to extract individual frames is a fundamental skill. Armed with the knowledge and code provided in this guide, you're now equipped to harness the power of Xuggler for frame capture in your Java applications. Explore, experiment, and unleash the potential of multimedia manipulation with Xuggler!