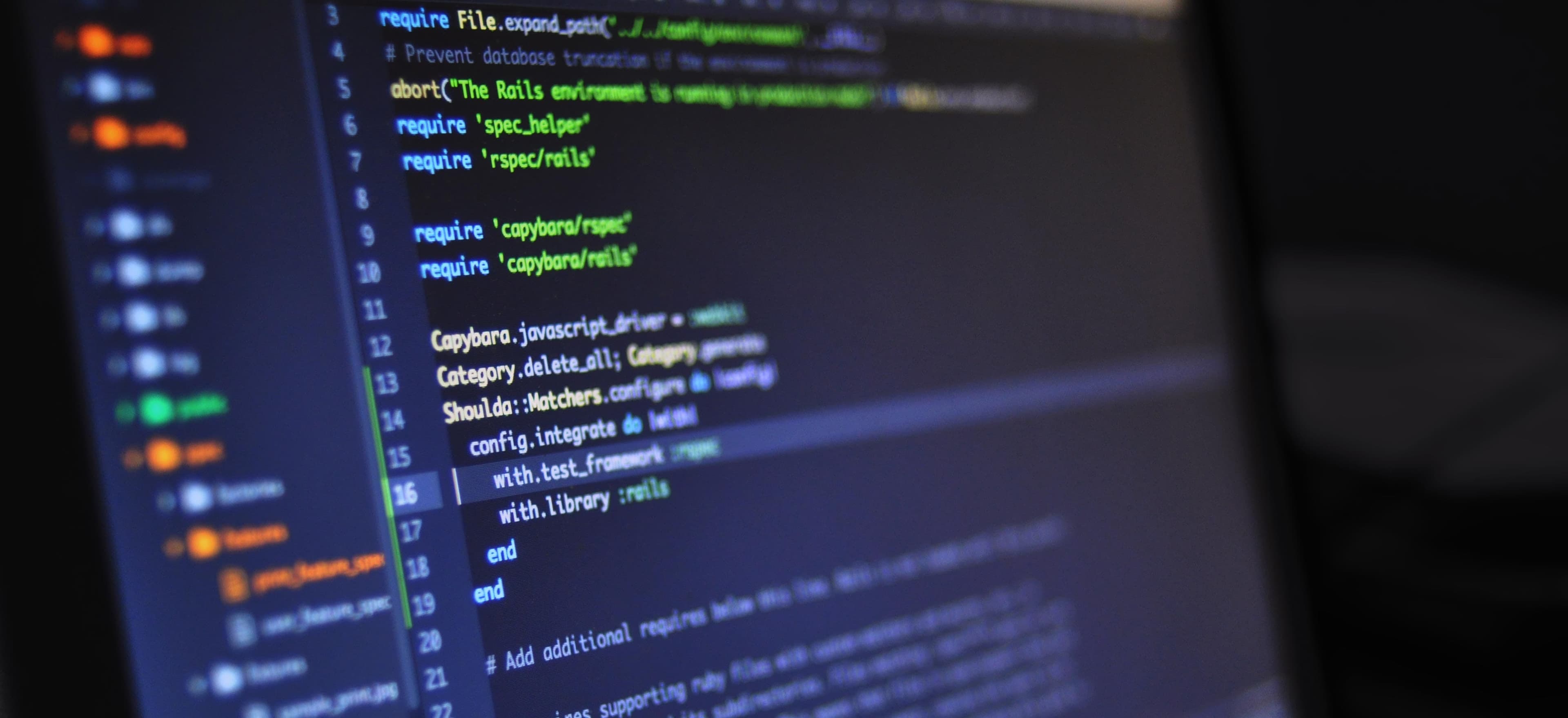
- Published on
Boost Your Spring Apps with Groovy: Dynamic Features Unleashed
If you are a Java developer leveraging the Spring framework, you might be aware of the power and flexibility it offers. However, have you ever considered making your Spring applications even more dynamic and expressive? Enter Groovy, a powerful, optionally-typed language that complements Java and seamlessly integrates with the Java Virtual Machine (JVM). In this post, we will explore how incorporating Groovy into your Spring applications can unlock a new level of dynamism and productivity.
What is Groovy?
Groovy is an agile and dynamic language for the Java Virtual Machine. It combines the best features of Java along with dynamic features from languages like Python and Ruby. With its elegant syntax and seamless interoperability with Java, Groovy has become increasingly popular among Java developers. Additionally, Groovy provides advanced features such as closures, runtime and compile-time meta-programming, and scripting capabilities.
Integrating Groovy with Spring
To begin our journey of integrating Groovy with Spring, let's start by adding Groovy support to a Spring Boot application. First, we need to include the necessary dependencies in our pom.xml
if we are using Maven or build.gradle
if we are using Gradle.
Maven
<dependencies>
<dependency>
<groupId>org.codehaus.groovy</groupId>
<artifactId>groovy-all</artifactId>
<version>3.0.7</version>
</dependency>
</dependencies>
Gradle
dependencies {
implementation 'org.codehaus.groovy:groovy-all:3.0.7'
}
With the Groovy dependency added, we can now start leveraging Groovy's dynamic features within our Spring application.
Dynamism in Action: Using Groovy for Configuration
One of the key areas where Groovy shines in a Spring application is in the realm of configuration. Let's take a look at how we can utilize Groovy's expressive syntax to configure a simple Spring bean.
Java Configuration
@Configuration
public class AppConfig {
@Bean
public GreetingService greetingService() {
return new GreetingServiceImpl();
}
}
Groovy Configuration
@Configuration
class AppConfig {
@Bean
GreetingService greetingService() {
new GreetingServiceImpl()
}
}
In the Groovy configuration, we notice the absence of explicit return statements and semicolons. Groovy's conciseness and expressiveness allow us to achieve the same result with a more succinct syntax.
Embracing Dynamic Typing with Groovy
Groovy's dynamic typing can be a game-changer when integrating with Spring. Consider a scenario where we want to deserialize JSON data into a Java object using Spring's RestTemplate
. Let's first look at how we achieve this in Java.
Java Approach
ResponseEntity<User> response = restTemplate.exchange(
"https://api.example.com/user/123",
HttpMethod.GET,
null,
User.class
);
User user = response.getBody();
In the Java approach, we explicitly specify the type User
for deserialization. Now, let's achieve the same result using Groovy's dynamic typing.
Groovy Approach
def response = restTemplate.exchange(
"https://api.example.com/user/123",
HttpMethod.GET,
null,
User
)
def user = response.body
In the Groovy approach, we leverage dynamic typing by omitting the explicit type declaration. The code is cleaner and more concise, thanks to Groovy's dynamic nature.
Leveraging Closures for Concise Event Handling
Another area where Groovy enhances Spring applications is in event handling, particularly with closures. Consider a scenario where we want to define an event listener using Spring's ApplicationListener
interface.
Java Event Listener
@Component
public class UserEventListener implements ApplicationListener<UserEvent> {
@Override
public void onApplicationEvent(UserEvent event) {
// Event handling logic
}
}
Groovy Event Listener
@Component
class UserEventListener implements ApplicationListener<UserEvent> {
@Override
void onApplicationEvent(UserEvent event) {
// Event handling logic
}
}
In the Groovy event listener, we notice a more concise syntax. With Groovy, we can write closures more naturally, resulting in cleaner and more expressive event handling code.
Runtime and Compile-time Meta-programming
Groovy's meta-programming capabilities bring a new dimension of flexibility to Spring applications. For instance, let's say we want to add a custom method to an existing class during runtime.
Java Meta-programming
// Not supported in Java
Groovy Runtime Meta-programming
String.metaClass.toTitleCase = {
delegate.toLowerCase().split(' ').collect { it.capitalize() }.join(' ')
}
String titleCaseString = "hello, world!".toTitleCase()
assert titleCaseString == "Hello, World!"
In the Groovy example, we dynamically add the toTitleCase
method to the String
class during runtime. This powerful feature allows for flexible enhancements to existing classes at runtime.
Integrating Groovy Scripts with Spring
Groovy's prowess in scripting also makes it an excellent choice for integrating dynamic scripts within Spring applications. Let's consider a scenario where we want to execute a Groovy script within a Spring context.
// Script.groovy
class Script {
def run() {
println "Executing the script within Spring context"
}
}
// Application context
def groovyShell = new GroovyShell()
def script = groovyShell.parse(new File("Script.groovy"))
script.run()
In the example above, we seamlessly execute a Groovy script within a Spring context using the GroovyShell
. This capability opens up a world of possibilities for dynamic scripting within Spring applications.
The Bottom Line
Groovy's dynamic features offer a compelling synergy with the Spring framework. From concise and expressive configurations to dynamic typing and powerful meta-programming capabilities, Groovy elevates the dynamism and productivity of Spring applications. By integrating Groovy into your Spring projects, you unlock a powerful combination that leverages the strengths of both languages. Embrace Groovy's dynamism and unleash a new level of versatility in your Spring applications!
Start integrating Groovy with Spring today and experience the dynamism firsthand!
Remember, the power of Groovy is at your fingertips—it's time to unleash it in your Spring applications!
Checkout our other articles