Unlocking Secrets of Effective Java Exception Handling
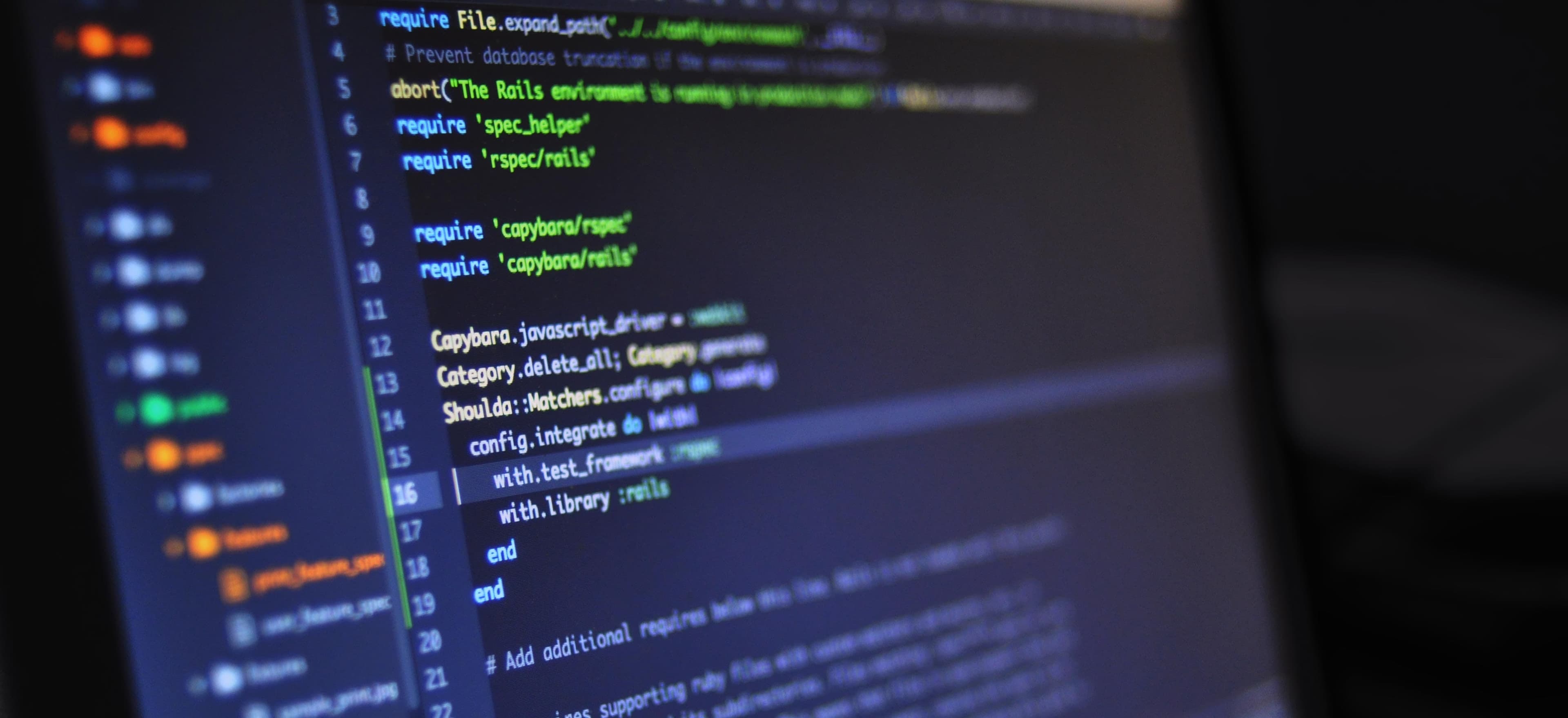
- Published on
Unlocking Secrets of Effective Java Exception Handling
Exception handling is an important aspect of programming in Java, as it allows developers to respond to unexpected events and errors in a controlled and graceful manner. In this blog post, we will delve into the best practices for exception handling in Java, exploring various strategies and techniques to make your code more robust and maintainable.
Understanding Exceptions in Java
In Java, an exception is an event that disrupts the normal flow of the program's instructions. When an error occurs, an object representing the error is created and thrown in the method that caused the error. This can be due to various reasons such as invalid input, network issues, or file I/O problems.
The try-catch
Block
The try-catch
block is the basic mechanism for handling exceptions in Java. It allows you to specify a block of code that may throw an exception, and catch and handle that exception if it occurs. This helps in preventing the program from crashing due to unhandled exceptions.
try {
// Code that may throw an exception
// ...
} catch (ExceptionType e) {
// Exception handling code
// ...
}
Why Use try-catch
Blocks?
The try-catch
block provides a structured way to handle exceptions, allowing you to separate normal code from error-handling code. It also prevents the propagation of unhandled exceptions, ensuring that your program remains stable and resilient in the face of unexpected errors.
Throwing Exceptions
In addition to handling exceptions, Java also allows you to create and throw your own exceptions. This can be useful when you want to signal an error condition in your code and propagate it to the calling code for handling.
public void doSomething() throws CustomException {
if (errorCondition) {
throw new CustomException("Error message");
}
}
Why Throw Custom Exceptions?
By throwing custom exceptions, you can provide meaningful error messages and differentiate between different types of errors in your code. This makes it easier for developers using your code to understand and handle specific error scenarios.
The finally
Block
The finally
block is used in conjunction with the try-catch
block to define a block of code that will be executed whether an exception is thrown or not. This is useful for performing cleanup tasks, such as closing file handles or releasing resources, regardless of whether an exception occurred.
try {
// Code that may throw an exception
// ...
} catch (ExceptionType e) {
// Exception handling code
// ...
} finally {
// Cleanup code
// ...
}
Why Use the finally
Block?
The finally
block ensures that essential cleanup tasks are carried out, even in the presence of exceptions. This is crucial for maintaining the integrity of resources and avoiding memory leaks or other undesirable side effects.
Exception Propagation
When an exception is thrown in a method, it can be propagated up the call stack to be handled by calling methods. This allows for centralized handling of exceptions at higher levels of the program, providing a systematic approach to dealing with errors.
public void doSomething() throws CustomException {
// Code that may throw an exception
// ...
}
Why Propagate Exceptions?
Exception propagation allows for a clean separation of concerns, where lower-level code doesn't need to worry about how exceptions are handled, and higher-level code can define the overall error-handling strategy for the entire application.
Exception Best Practices
Now that we've covered the basics of exception handling in Java, let's explore some best practices to ensure effective and robust exception handling in your code.
-
Use Specific Exception Types: Always use the most specific exception type that accurately represents the error condition. This helps in writing clear and precise exception-handling code.
-
Avoid Catching General Exceptions: Catching overly broad exception types such as
Exception
orThrowable
can make it difficult to diagnose and recover from specific errors. Instead, catch only those exceptions that you can handle or are expected to occur. -
Provide Descriptive Error Messages: When creating custom exceptions or handling existing ones, include informative error messages that convey the nature and cause of the error. This aids in troubleshooting and debugging.
-
Log Exceptions: Always log exceptions and their stack traces. This can be immensely helpful in diagnosing issues and understanding the flow of execution in case of errors.
-
Handle Exceptions Appropriately: Consider the appropriate action to take when an exception occurs. This could involve retrying the operation, rolling back a transaction, or gracefully degrading functionality.
The Bottom Line
Exception handling is a critical aspect of writing reliable and maintainable Java code. By understanding the mechanisms and best practices for handling exceptions, you can ensure that your code is resilient in the face of unexpected errors and provides meaningful feedback to users and maintainers.
Remember, effective exception handling not only prevents program crashes but also contributes to a better user experience and quicker issue resolution.
By incorporating these best practices and understanding the nuances of exception handling in Java, you can unlock the secrets to writing robust and error-tolerant applications.
To continue learning more about Java exception handling, check out Oracle's official documentation and Baeldung's in-depth guide.
Checkout our other articles