Cut the Costs: Unveiling Hidden Expenses in Continuous Delivery
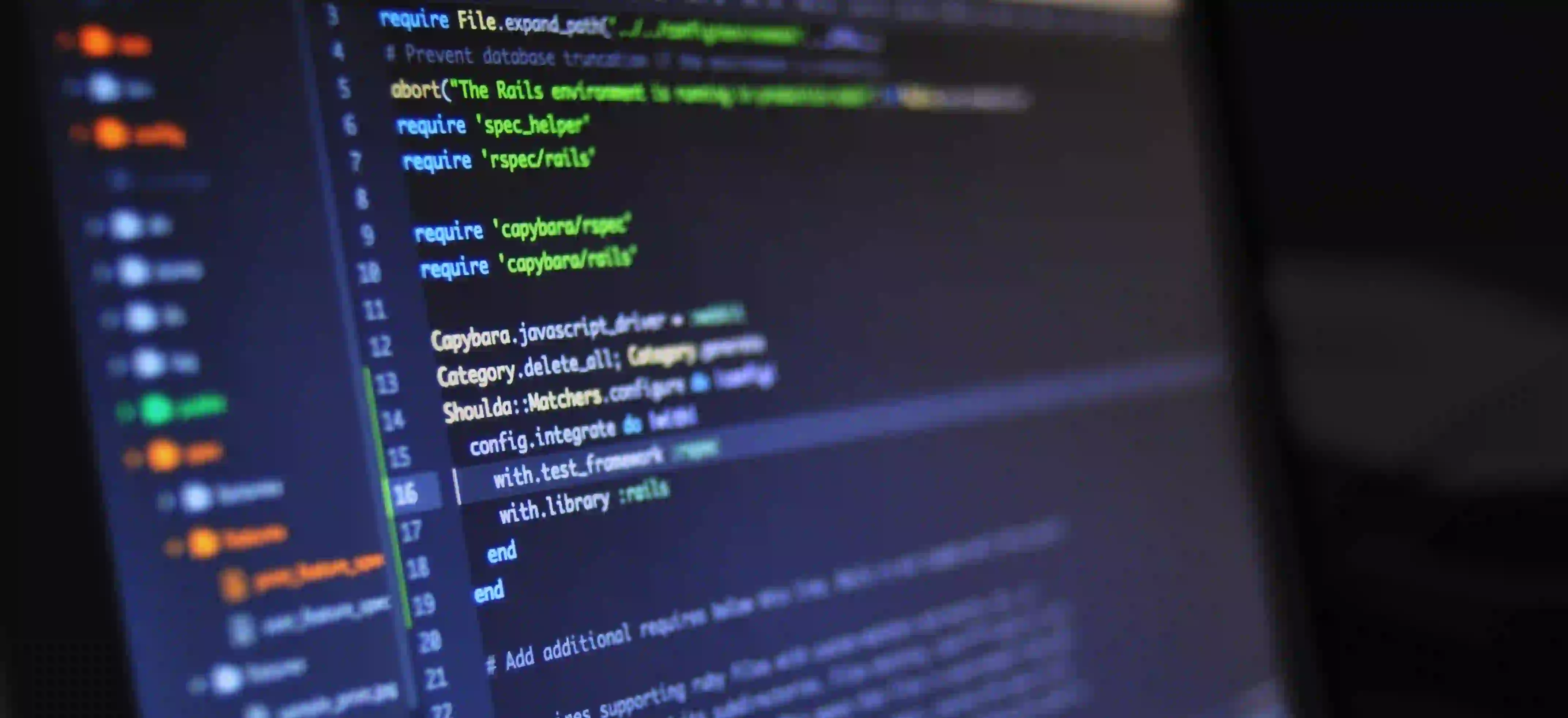
The Hidden Expenses in Continuous Delivery: How to Optimize Your Java Code
As a Java developer, you're familiar with the concept of continuous delivery, a practice that allows you to ship code to production in a safe, quick, and sustainable way. However, what you might not be aware of are the hidden costs associated with continuous delivery in Java development. In this article, we'll delve into the nuances of continuous delivery in Java, uncover the hidden expenses, and explore how you can optimize your Java code to cut costs.
The Ubiquitous Overhead
Continuous delivery comes with its fair share of overheads, especially in Java development. The buildup of redundant code, inefficient algorithms, and suboptimal resource utilization can significantly inflate your operational costs. One of the key culprits is the excessive consumption of memory, often resulting from careless coding practices.
Consider the following Java code snippet, where a list of integers is sorted using the Collections.sort
method:
List<Integer> numbers = new ArrayList<>();
// Populate the list...
Collections.sort(numbers);
At first glance, this code appears innocent. However, as the size of the list grows, the memory footprint of the Collections.sort
method can become prohibitive. By opting for a more memory-efficient sorting algorithm, such as merge sort or quicksort, you can drastically reduce the hidden memory expenses associated with sorting large collections in Java.
Balancing Performance and Complexity
Java developers often face the dilemma of balancing performance and complexity. While it's tempting to resort to intricately layered architectures and convoluted design patterns in pursuit of optimal performance, the associated maintenance and runtime costs can be substantial.
Let's consider an example where a Java application makes extensive use of nested for-loops for data processing:
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
// Nested operations...
}
}
While nested for-loops might seem innocuous, they can lead to quadratic time complexity, especially with large input sizes. This can result in unanticipated operational expenses arising from increased computational overhead. By refactoring the code to employ more efficient algorithms, such as divide and conquer or dynamic programming, you can strike a balance between performance and complexity, ultimately curbing hidden costs in continuous delivery.
Harnessing the Power of Multithreading
Java’s support for multithreading is a double-edged sword. While it offers parallelism and concurrent execution, it also introduces the risk of contention, deadlock, and thread synchronization overheads, all of which can escalate operational costs.
Consider a scenario where a multithreaded Java application experiences contention due to shared resources:
public class SharedResource {
private int value;
public synchronized void increment() {
value++;
}
}
In this case, the synchronized method introduces a bottleneck, leading to contention among multiple threads accessing the increment
method. This contention can amplify operational expenses and hinder the scalability of the application.
To mitigate these hidden costs, consider using non-blocking algorithms, such as compare-and-swap (CAS) instructions and atomic variables, to alleviate contention and synchronization overheads in multithreaded Java applications.
Efficient Memory Management
Memory management is a critical aspect of optimizing Java code for continuous delivery. Inefficient memory allocation and deallocation can give rise to hidden expenses, including garbage collection overhead and memory fragmentation.
Consider the following Java code snippet, where a large number of objects are repeatedly created and discarded:
for (int i = 0; i < n; i++) {
SomeObject obj = new SomeObject();
// Perform operations...
}
In this scenario, the frequent creation and subsequent disposal of SomeObject
instances can strain the garbage collector and result in excessive memory churn. By implementing object pooling and reusing existing objects, you can minimize the hidden costs associated with memory allocation and garbage collection in Java.
Key Takeaways
Continuous delivery in Java presents a plethora of hidden expenses, ranging from memory overheads and computational complexities to multithreading contention and memory management inefficiencies. By optimizing your Java code to mitigate these expenses, you can streamline your continuous delivery pipeline, enhance operational efficiency, and cut costs in the long run.
As you strive for continuous improvement in your Java development endeavors, it's imperative to remain cognizant of these hidden expenses and adopt proactive measures to address them. By leveraging memory-efficient algorithms, streamlining computational complexities, harnessing the power of multithreading judiciously, and implementing efficient memory management techniques, you can pave the way for a cost-effective and sustainable continuous delivery process in Java.