Akka Actors: Solving Message Overload Efficiently
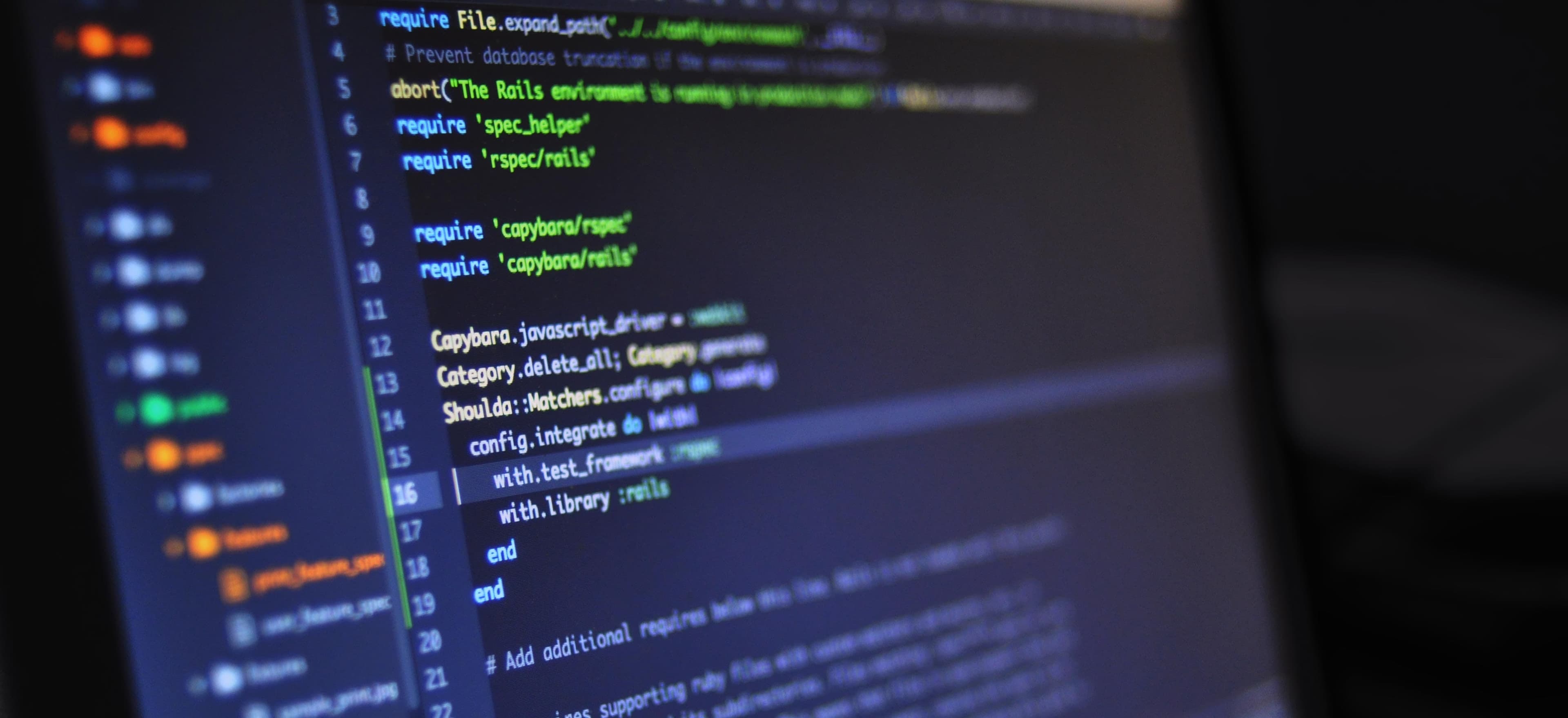
- Published on
Akka Actors: Solving Message Overload Efficiently
In modern software development, building concurrent and distributed systems is a common requirement. When it comes to Java, Akka is a powerful toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications.
Setting the Scene to Akka Actors
In Akka, the actor model provides a higher level of abstraction for writing concurrent and distributed systems. Actors are essentially small, independent entities that communicate through messages. They encapsulate state and behavior, and only communicate through message passing.
Handling Message Overload
In a highly concurrent system, it's common to encounter message overload, where an actor is receiving messages at a rate that it cannot handle efficiently. This can lead to increased memory consumption, degraded performance, and potential system crashes. Akka provides several powerful mechanisms for handling message overload efficiently.
1. Mailboxes
In Akka, each actor has its own mailbox, which acts as a queue for incoming messages. By default, Akka uses an unbounded mailbox, meaning that messages will be queued indefinitely. While this behavior may seem convenient, it can lead to message overload and potential memory issues.
To address this, Akka allows you to configure a bounded mailbox for actors, limiting the maximum number of messages that can be queued. By doing so, the system can gracefully handle message overload by either dropping excess messages or applying a custom overflow strategy.
// Example of configuring a bounded mailbox in Akka
Mailbox mailbox = BoundedMailbox.create(1000);
Props props = Props.create(MyActor.class).withMailbox(mailbox);
By using a bounded mailbox and carefully choosing an overflow strategy, you can prevent message overload from impacting the overall system performance.
2. Backpressure
Another powerful mechanism provided by Akka for handling message overload is backpressure. In a message-driven system, backpressure is the ability to signal to a message sender that the receiver is not able to keep up with the rate of incoming messages.
Akka Streams, a part of the Akka toolkit, provides built-in support for backpressure. When implementing stream processing with Akka Streams, backpressure can be used to throttle the rate of incoming messages, preventing overload and ensuring that the system operates within its capacity.
// Example of implementing backpressure with Akka Streams
Source<String, NotUsed> source = // ...
Sink<String, CompletionStage<Done>> sink = // ...
source.via(flow).to(sink).run(materializer);
By integrating backpressure into your message-driven systems, you can effectively manage message overload and prevent cascading failures due to excessive message processing.
3. Supervision Strategies
In Akka, supervision strategies allow you to define how an actor should handle failures, including scenarios where message overload leads to exceptions being thrown. By defining custom supervision strategies, you can control the behavior of actors in response to message processing errors.
// Example of defining a custom supervision strategy in Akka
OneForOneStrategy strategy = new OneForOneStrategy(
10,
Duration.ofMinutes(1),
DeciderBuilder.match(ArithmeticException.class, ex -> SupervisorStrategy.restart())
.matchAny(ex -> SupervisorStrategy.stop())
.build()
);
By carefully defining supervision strategies, you can ensure that the impact of message overload on the system is minimized, and that failed actors are appropriately managed to prevent cascading failures.
The Closing Argument
In the realm of Java development, Akka provides a robust set of tools for building highly concurrent and distributed systems. When it comes to handling message overload efficiently, Akka's support for bounded mailboxes, backpressure, and supervision strategies equips developers with the means to build resilient and responsive message-driven applications.
By carefully considering and implementing these mechanisms, developers can ensure that their Akka-based systems can gracefully handle message overload, preventing degradation of performance and maintaining system stability.
In conclusion, Akka's actor model, combined with its powerful support for managing message overload, makes it a highly compelling choice for building modern, resilient, and scalable Java applications.
For more in-depth information about Akka Actors and managing message overload, feel free to visit the official Akka documentation and Lightbend's Akka guide.
Checkout our other articles