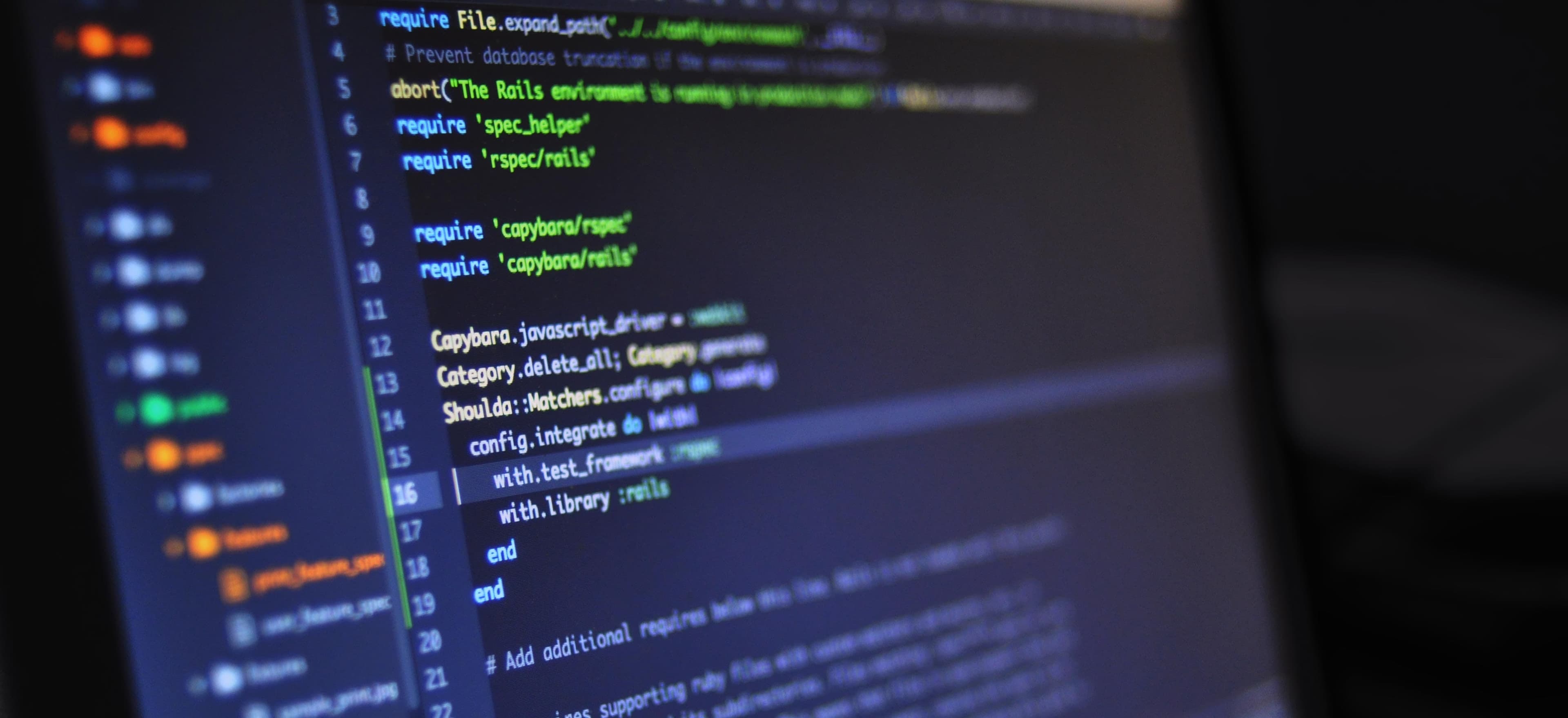
- Published on
Achieving Seamless Integration of Spring and GWT in Java Web Applications
In the world of Java web development, the integration of different frameworks and technologies is crucial to building robust and scalable applications. Spring and Google Web Toolkit (GWT) are two powerful tools in the Java ecosystem, and when used together, they can create a seamless and efficient development experience.
Understanding Spring and GWT
Spring Framework
Spring is a widely used open-source framework that provides comprehensive infrastructure support for developing Java applications. It offers a wide range of features, including inversion of control (IoC), aspect-oriented programming (AOP), and integration with other frameworks for various concerns such as data access, security, and more.
Google Web Toolkit (GWT)
Google Web Toolkit, or GWT, is a development toolkit for building and optimizing complex browser-based applications. It allows developers to write client-side applications in Java and then cross-compile them into highly optimized JavaScript.
The Challenge of Integration
One of the challenges in web application development is integrating the server-side components (such as those provided by the Spring framework) with the client-side components (such as those built with GWT). This integration is crucial for creating a cohesive and efficient web application.
Leveraging Spring-GWT Integration
Integrating Spring with GWT allows developers to leverage the strengths of both frameworks, providing a robust server-side infrastructure and a powerful client-side development experience. By doing so, they can build high-performance, maintainable, and scalable web applications.
Setting Up the Integration
To integrate Spring with GWT, the following steps need to be performed:
-
Create a GWT Module
Create a GWT module that will serve as the entry point for the client-side application.
package com.example.myapp.client; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.core.client.GWT; import com.google.gwt.user.client.ui.RootPanel; public class MyApp implements EntryPoint { public void onModuleLoad() { // Client-side initialization code here } }
The GWT module serves as the starting point of the client-side application and is typically responsible for setting up the user interface and initializing any required components.
-
Create Spring Controllers
Create Spring controllers that will handle incoming requests from the GWT client. These controllers will process the requests, interact with the business logic, and return the appropriate response.
@RestController @RequestMapping("/api") public class MyController { @Autowired private MyService myService; @RequestMapping("/data") public ResponseEntity<?> getData() { // Process the request using myService // Return the response } }
Spring controllers are responsible for handling incoming HTTP requests and generating the appropriate HTTP responses. They can interact with the business logic and use services to process the requests.
-
Configure Spring and GWT
Configure the Spring application context to manage the server-side components and beans. This involves setting up the necessary dependencies, mappings, and configurations for the Spring controllers and services.
<context:component-scan base-package="com.example.myapp.server" />
GWT-RPC can be used to communicate between the client and server, allowing remote procedure calls to be made from the client to the server.
-
Integrate GWT-RPC with Spring
Integrate GWT-RPC with Spring by creating service interfaces that extend RemoteService. Then, create corresponding service implementation classes that are managed by Spring.
public interface DataService extends RemoteService { Data fetchData(); } @Service("dataService") public class DataServiceImpl extends RemoteServiceServlet implements DataService { @Autowired private MyService myService; public Data fetchData() { // Implement the fetchData method using myService } }
Integrate GWT-RPC with Spring by leveraging Spring's dependency injection and managing the GWT service implementations as Spring beans. This allows the GWT client to communicate with the server-side components seamlessly.
Advantages of Spring-GWT Integration
Integrating Spring with GWT offers several advantages:
-
Server-side Scalability
Spring provides robust support for building scalable and maintainable server-side components. By integrating Spring with GWT, developers can leverage this scalability while building the client-side components in GWT.
-
Code Reusability
The integration allows for reusing existing Spring components and services on the server side, avoiding the need to rewrite business logic for the client-side functionality.
-
Strong Typing and Compilation
GWT's Java-to-JavaScript compilation process ensures strong typing and early error detection, providing a more robust and maintainable client-side codebase.
-
Asynchronous Communication
GWT's support for asynchronous communication enables efficient client-server interactions, providing a responsive user experience.
The Last Word
In conclusion, integrating Spring with GWT allows developers to create powerful, scalable, and maintainable web applications by leveraging the strengths of both frameworks. By following the steps outlined above, developers can seamlessly integrate server-side components built with Spring with client-side components built with GWT, creating a cohesive and efficient web application.
Integrating these two powerhouse frameworks together truly unlocks the potential for building high-performance, maintainable, and scalable web applications in the Java ecosystem.
So, the next time you embark on a Java web application development journey, consider harnessing the power of Spring-GWT integration for a seamless and rewarding development experience.
Happy coding!
For more information on Spring and GWT integration, you can refer to the following resources:
Feel free to share your thoughts and experiences with Spring-GWT integration in the comments below!
Checkout our other articles