Guava Splitter vs StringUtils: Which is More Efficient?
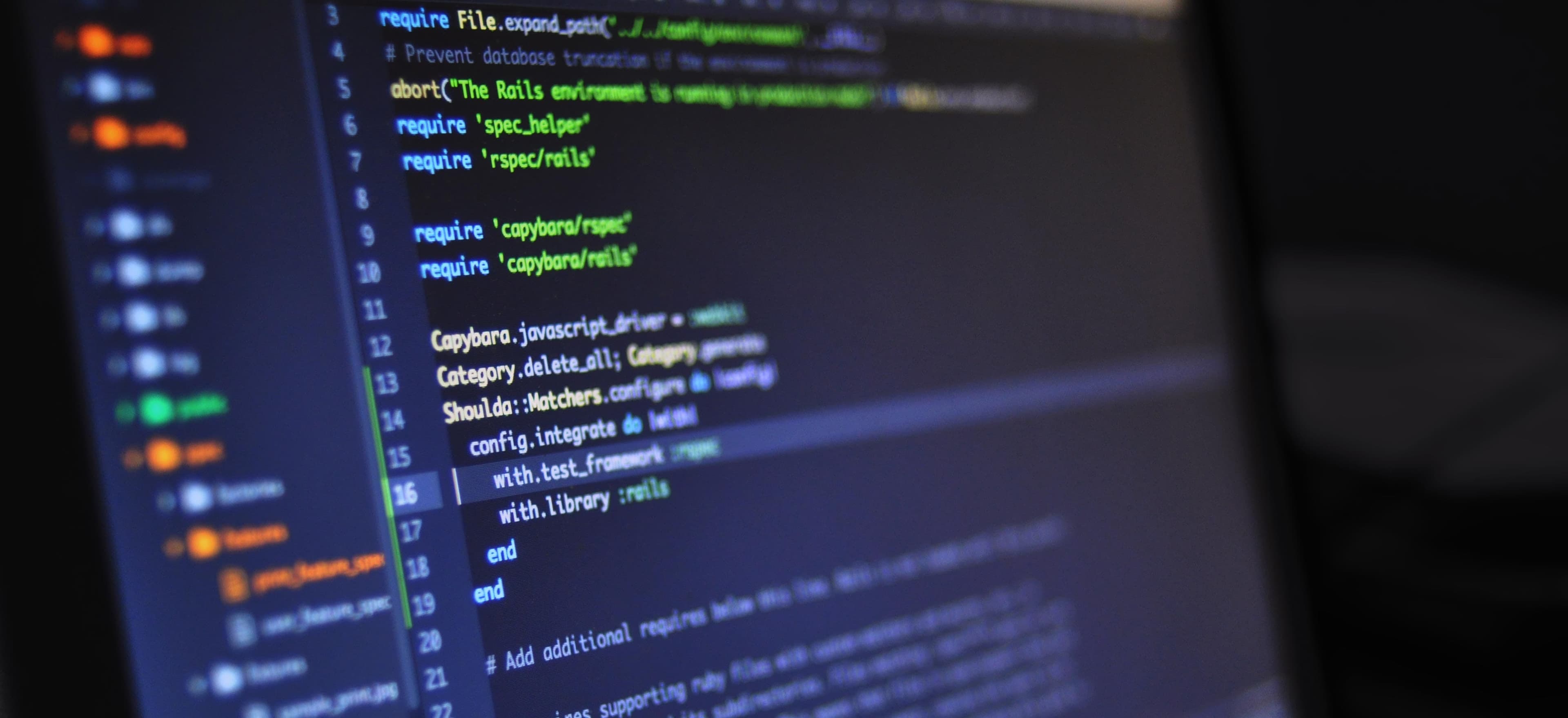
- Published on
When it comes to splitting strings in Java, developers often find themselves weighing the pros and cons of various approaches. Two widely used methods for string splitting are the Guava Splitter and the StringUtils from Apache Commons Lang. While both serve the same basic purpose, there are nuances in their functionality and efficiency that warrant a closer examination.
Getting Started to String Splitting
String splitting is a common operation in Java programming and is the process of breaking a string into an array of substrings based on a delimiter. This operation comes in handy for parsing input, handling textual data, and manipulating strings. In Java, developers have a multitude of tools at their disposal for string splitting, with Guava Splitter and StringUtils being two popular choices.
Guava Splitter
Guava, developed by Google, is a set of core libraries that includes Splitter, a utility class for splitting strings. One of the standout features of Guava Splitter is its expressive and fluent API that allows developers to specify various options for string splitting.
Let's consider an example of using Guava Splitter to split a comma-separated string:
String input = "apple,banana,orange";
Iterable<String> result = Splitter.on(',')
.trimResults()
.split(input);
In this example, the Splitter.on(',')
specifies the delimiter as a comma, and .trimResults()
is used to trim the whitespace from the elements obtained after splitting. This shows the readability and ease of use offered by Guava Splitter's API.
StringUtils from Apache Commons Lang
Apache Commons Lang is a popular library that provides a host of utilities for common programming tasks. Among its many offerings, StringUtils includes methods for string manipulation, including string splitting.
Using StringUtils to split a string looks like this:
String input = "apple,banana,orange";
String[] result = StringUtils.split(input, ",");
In this example, StringUtils.split
takes the input string and the delimiter as parameters and returns an array of substrings. While StringUtils offers a straightforward approach to string splitting, it may lack the nuanced options provided by Guava Splitter.
Efficiency Comparison
Now, let's delve into the efficiency comparison between Guava Splitter and StringUtils. Efficiency in this context could encompass factors such as performance, resource utilization, and code simplicity.
Performance
In terms of performance, both Guava Splitter and StringUtils provide efficient ways to split strings. However, microbenchmarking and profiling specific use-cases in your application may be necessary to draw definitive conclusions. It's important to note that the performance difference between the two may not be significant for most applications.
Resource Utilization
When it comes to resource utilization, Guava Splitter and StringUtils are both designed to be memory-efficient. They are unlikely to cause substantial differences in memory consumption during string splitting operations.
Code Simplicity and Readability
While both libraries offer clean and readable APIs, Guava Splitter's fluent interface provides a more expressive and elegant way of defining the splitting behavior. This can lead to more maintainable and understandable code, especially when dealing with complex splitting requirements.
A Final Look
In conclusion, the choice between Guava Splitter and StringUtils for string splitting in Java ultimately depends on the specific requirements of the project. If your use-case demands a high degree of flexibility and expressiveness in defining splitting options, Guava Splitter may be the preferred choice. On the other hand, for straightforward string splitting needs, StringUtils from Apache Commons Lang offers a reliable and simple solution.
It's worth noting that both Guava and Apache Commons Lang are robust libraries with a wide range of functionalities beyond string splitting. It's advisable to consider the overall requirements of your project and evaluate the suitability of these libraries in the broader context of your application.
In the end, whether you opt for the expressive elegance of Guava Splitter or the simplicity of StringUtils, the most crucial factor is to write clean, maintainable, and efficient code that best serves the needs of your application.
In the ever-evolving landscape of Java development, having a keen understanding of the available tools and libraries empowers developers to make informed choices that contribute to the robustness and performance of their applications.
So, which string splitting utility do you prefer in your Java projects? Let's keep the discussion going!
Find out more about string manipulation in Java by exploring the official documentation and dive deeper into the capabilities of Guava and Apache Commons Lang.
Checkout our other articles