Master JPA: Unified Exception Handling & VO Validations
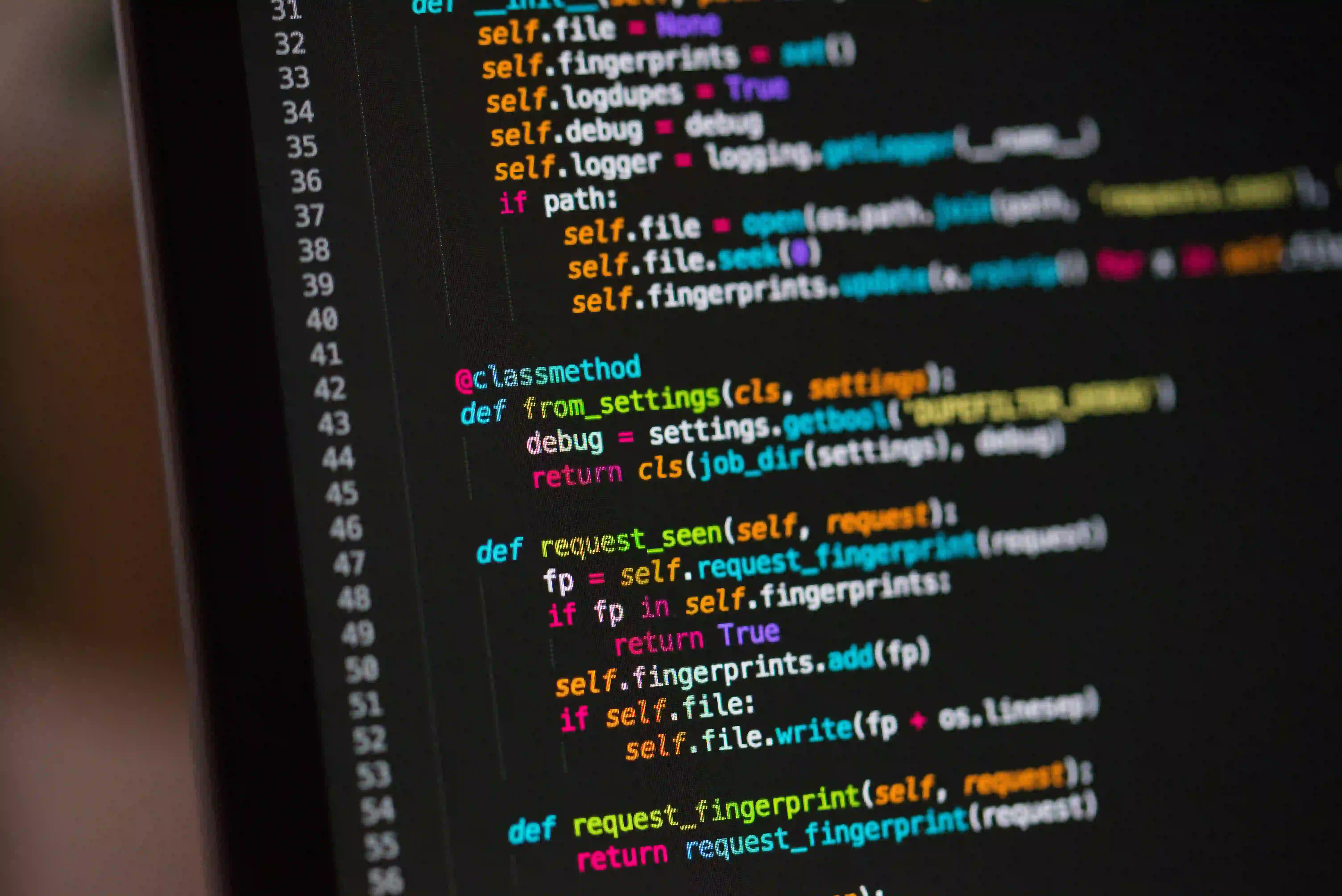
Master JPA: Unified Exception Handling & VO Validations
When developing applications with Java Persistence API (JPA), it's crucial to handle exceptions gracefully and ensure the validity of Value Objects (VO) to maintain data integrity. In this blog post, we'll delve into unified exception handling and VO validations in JPA, empowering you to master these essential aspects of JPA development.
Unified Exception Handling in JPA
Exception handling is a critical aspect of any application, and JPA is no exception (excuse the pun). Unified exception handling enables you to centralize and streamline the handling of JPA-related exceptions. Rather than scattering exception handling logic throughout your codebase, you can consolidate it in a single location, enhancing maintainability and readability.
Let's consider a simple example of unified exception handling in JPA using Spring Data JPA.
@ControllerAdvice
public class ExceptionHandlerController {
@ExceptionHandler(DataIntegrityViolationException.class)
public ResponseEntity<String> handleDataIntegrityViolationException(DataIntegrityViolationException ex) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("Data integrity violation: " + ex.getMessage());
}
@ExceptionHandler(EntityNotFoundException.class)
public ResponseEntity<String> handleEntityNotFoundException(EntityNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("Entity not found: " + ex.getMessage());
}
// Other exception handlers
}
In the above code, @ControllerAdvice
is used to define global exception handlers for the specified exception types. When an exception occurs within the application, these handlers will intercept the exception and respond accordingly, providing a consistent and structured approach to exception handling.
By centralizing exception handling in this manner, you can easily manage and customize error responses, ensuring a consistent user experience across your application.
Value Object Validations in JPA
Value Objects (VO) are immutable objects that encapsulate attributes, thus representing an entity's state. It's imperative to validate VO attributes to maintain data integrity within the JPA context. Leveraging the Bean Validation API (JSR 380), you can apply declarative constraints on VO attributes, ensuring they adhere to specific validation rules.
Consider a simple VO representing a user's details with validation constraints.
public class UserVO {
@NotNull
@Size(min = 2, max = 50)
private String username;
@Email
private String email;
// Getters and setters
}
In the above code, the @NotNull
and @Size
constraints ensure that the username
attribute is not null and meets the specified length criteria. Similarly, the @Email
constraint validates the email
attribute for a valid email format.
By incorporating such validations, you can preemptively ensure the integrity of VO attributes, mitigating the risk of invalid data being persisted within the JPA context.
Why Unified Exception Handling & VO Validations Matter
Unified exception handling and VO validations are pivotal in JPA development for several compelling reasons:
-
Consistency: Centralized exception handling fosters a consistent approach to managing and responding to exceptions across the application, enhancing user experience and maintainability.
-
Data Integrity: VO validations ascertain that only valid data is persisted, safeguarding the integrity of the JPA entities and database records.
-
Maintenance: Consolidating exception handling logic streamlines maintenance by reducing redundancy and improving code readability.
-
Compliance: By adhering to standard validation constraints, you ensure that data meets predefined criteria, thus adhering to regulatory and domain-specific requirements.
Final Considerations
In this blog post, we've explored the significance of unified exception handling and VO validations in JPA development. By mastering these aspects, you can fortify your JPA-powered applications with robust error management and data integrity, setting the stage for reliable and resilient software solutions.
Unified exception handling and VO validations not only bolster the reliability of your JPA applications but also contribute to a more manageable and scalable codebase. Embrace these practices to elevate your JPA development prowess and deliver enhanced user experiences.