Overcoming the Pitfalls of Project Estimations
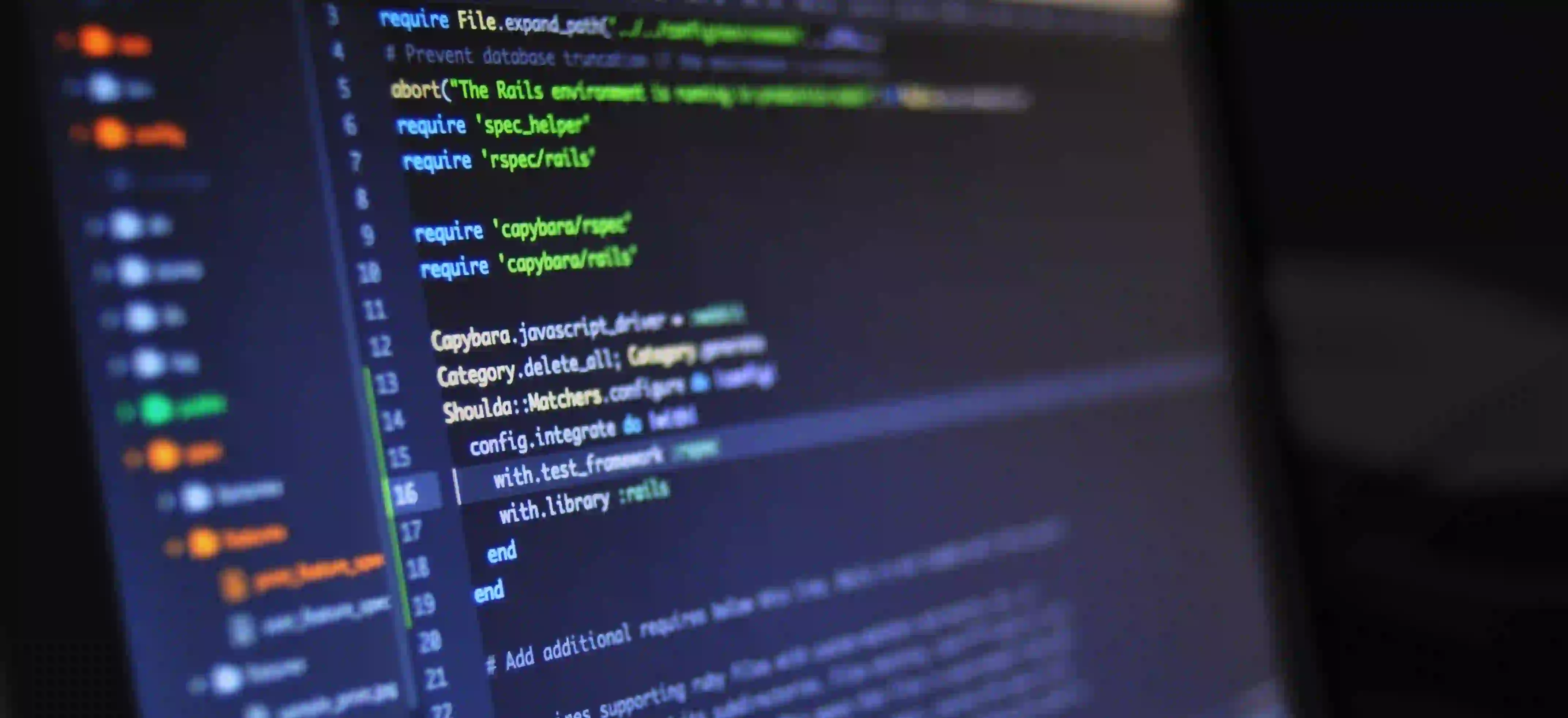
Overcoming the Pitfalls of Project Estimations
Estimating a project's scope, time, and resource requirements is a crucial aspect of software development. Yet, project estimations are often fraught with challenges, leading to missed deadlines, exceeded budgets, and dissatisfied stakeholders. In this article, we'll delve into the common pitfalls of project estimations and explore strategies to overcome them using Java.
The Importance of Accurate Project Estimations
Accurate project estimations are vital for several reasons:
-
Resource Allocation: Proper estimations assist in allocating the right resources, including human resources, time, and budget, ensuring optimal utilization.
-
Risk Management: Realistic estimations help in identifying potential risks and devising mitigation strategies early in the project lifecycle.
-
Stakeholder Expectations: Clear and accurate estimations aid in managing stakeholder expectations, fostering trust and collaboration.
Common Pitfalls of Project Estimations
1. Overlooking Complexity
Projects often contain hidden complexities that are not immediately apparent. Failure to account for these complexities leads to underestimated timelines and resources.
2. Optimistic Bias
Optimism is healthy, but overly optimistic estimations can set unrealistic expectations and lead to project delays and dissatisfaction.
3. Inadequate Requirements Analysis
Insufficient understanding of project requirements results in inaccurate estimations. Changes and additions during the project can further exacerbate this issue.
4. Not Factoring in Dependencies
Dependencies on external systems, libraries, or teams are often overlooked, leading to delays and disruptions.
5. Unrealistic Assumptions
Making unrealistic assumptions about team productivity, unforeseen obstacles, or environmental factors can skew estimations.
Strategies to Overcome Estimation Pitfalls using Java
1. Leveraging Historical Data
In Java, you can utilize historical data from previous projects to derive metrics such as lines of code written per hour, average bug fix time, and feature implementation rate. By analyzing this data, you can make more informed estimations for similar tasks in future projects.
// Example of utilizing historical data for estimation
int linesOfCodePerHour = 50;
int totalLinesOfCode = calculateTotalLinesOfCode();
int estimatedHours = totalLinesOfCode / linesOfCodePerHour;
In this example, the historical data on lines of code per hour is used to estimate the time required for a new task based on its size.
2. Embracing Agile Methodologies
Java, with its strong support for agile practices, allows for iterative development and frequent reassessment of project estimations. By breaking down tasks into smaller, manageable units, you can continuously refine and adjust estimations based on real-time feedback.
// Using Java for implementing agile practices
public void implementFeature(Feature feature) {
// Agile development approach
// Break down feature into smaller tasks and estimate time for each task
}
3. Applying Monte Carlo Simulations
Monte Carlo simulations, implemented in Java using libraries such as Apache Commons Math, can help in generating multiple scenarios based on probabilistic inputs, providing a more nuanced view of project timelines and resource requirements.
// Example of using Apache Commons Math for Monte Carlo simulations
double[] taskDurations = {5.2, 6.3, 4.8, 7.1, 5.9}; // Sample task durations
NormalDistribution normalDistribution = new NormalDistribution(mean, standardDeviation); // Create a normal distribution
double[] simulatedDurations = normalDistribution.sample(1000); // Simulate 1000 task durations
By simulating task durations, you can gain insights into potential project timelines considering variability and uncertainty.
4. Continuous Integration and Delivery (CI/CD)
Java's robust support for CI/CD practices enables frequent integration and deployment, providing real-time feedback on project progress. This feedback loop allows for accurate adjustments to estimations based on actual development and deployment metrics.
// Java's support for CI/CD
public void deployToProduction() {
// Continuous integration and delivery pipeline in Java
// Monitor deployment times and effectiveness for estimation refinement
}
5. Risk-based Estimations
Incorporating risk-based estimations into Java projects involves identifying potential risks early on, assessing their impact on project timelines, and factoring in buffer times to mitigate these risks.
// Incorporating risk-based estimations in Java projects
int optimisticEstimation = calculateOptimisticEstimation();
int pessimisticEstimation = calculatePessimisticEstimation();
int mostLikelyEstimation = calculateMostLikelyEstimation();
int expectedEstimation = (optimisticEstimation + (4 * mostLikelyEstimation) + pessimisticEstimation) / 6;
Using the PERT formula in this example, you can calculate a more realistic estimation by factoring in best-case, worst-case, and most likely scenarios.
Key Takeaways
Accurate project estimations are essential for the successful execution of software development projects. By leveraging Java's capabilities and adopting strategies such as historical data analysis, agile methodologies, Monte Carlo simulations, CI/CD practices, and risk-based estimations, you can navigate and overcome the common pitfalls associated with project estimations. These techniques, combined with a proactive and adaptable mindset, can contribute to improved project planning, execution, and stakeholder satisfaction in the ever-evolving landscape of software development.