Cracking the Code: Mastering Template Method in Java
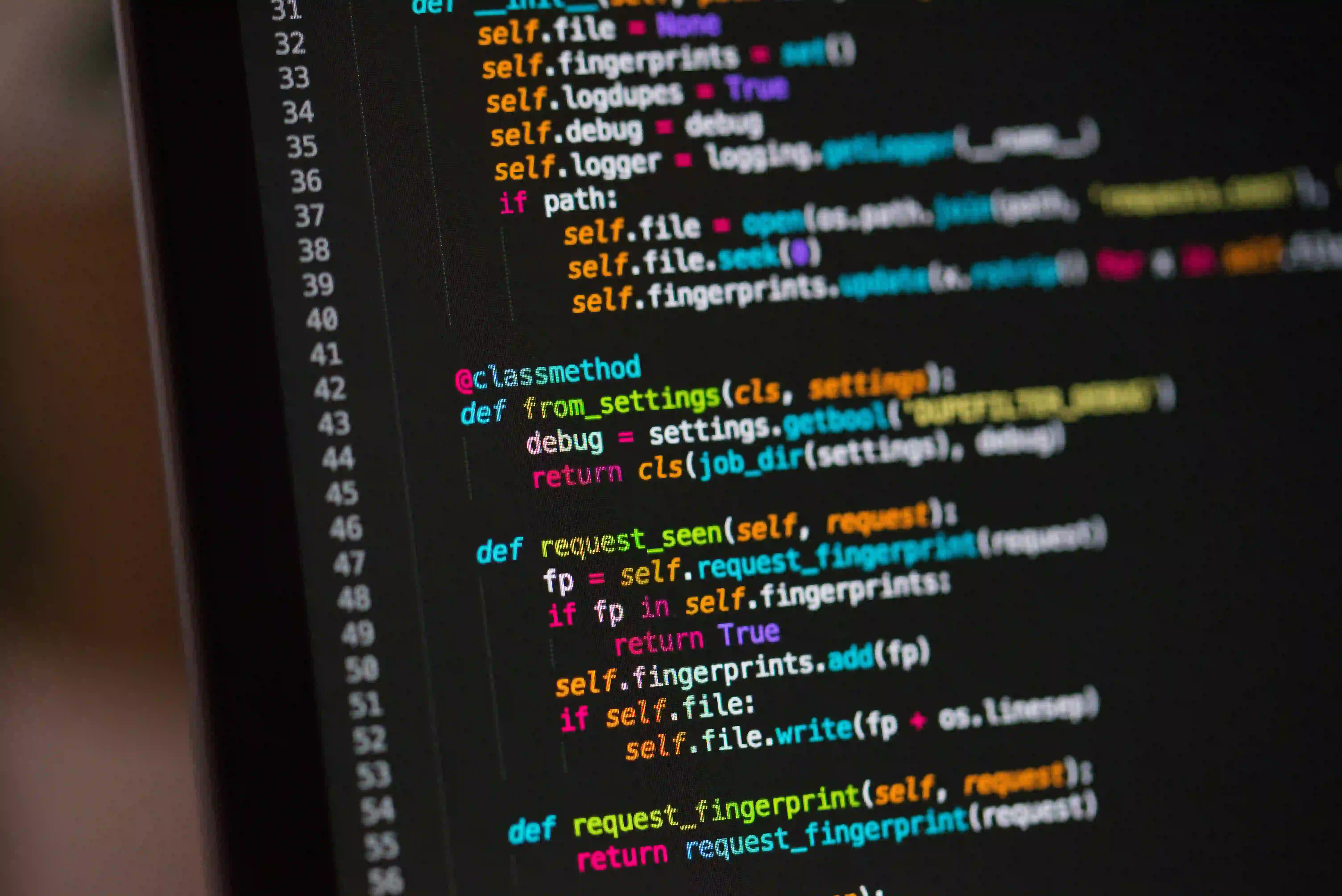
Cracking the Code: Mastering Template Method in Java
If you are an aspiring Java developer, you are probably familiar with the phrase "Don't repeat yourself" (DRY) and the importance of designing reusable code components. One design pattern that can help you achieve this is the Template Method pattern. In this post, we will dive deep into the world of Template Method in Java, understand its significance, and learn how to implement it effectively.
Understanding the Template Method Pattern
The Template Method pattern is a behavioral design pattern that defines the skeleton of an algorithm in a method, deferring some steps to subclasses. This pattern allows subclasses to redefine certain steps of an algorithm without altering its structure. It promotes code reusability and helps in defining a high-level algorithm while allowing flexibility in some of the implementation details.
How the Template Method Works
At the core of the Template Method pattern lies a template method that outlines the algorithm's structure by defining the sequence of steps to be followed. Some of these steps are left abstract, allowing concrete subclasses to provide their own implementations. The template method then calls these abstract steps at appropriate points within the algorithm.
Implementing the Template Method Pattern in Java
Now, let's delve into a practical example to understand how the Template Method pattern can be implemented in Java.
Consider a scenario where we need to define a general algorithm for building a house, but the specific steps involved in building a wooden house differ from those of a concrete house. We can use the Template Method pattern to address this requirement.
Step 1: Create an Abstract Class
public abstract class HouseBuilder {
public final void buildHouse() {
buildFoundation();
buildWalls();
buildRoof();
if (addExtraFeatures()) {
addExtraFeatures();
}
}
protected abstract void buildFoundation();
protected abstract void buildWalls();
protected abstract void buildRoof();
protected boolean addExtraFeatures() {
return true;
}
}
Step 2: Create Concrete Subclasses
public class WoodenHouseBuilder extends HouseBuilder {
@Override
protected void buildFoundation() {
// Implement building wooden house foundation
}
@Override
protected void buildWalls() {
// Implement building wooden house walls
}
@Override
protected void buildRoof() {
// Implement building wooden house roof
}
@Override
protected boolean addExtraFeatures() {
// Implement adding extra features specific to wooden house
return true;
}
}
public class ConcreteHouseBuilder extends HouseBuilder {
@Override
protected void buildFoundation() {
// Implement building concrete house foundation
}
@Override
protected void buildWalls() {
// Implement building concrete house walls
}
@Override
protected void buildRoof() {
// Implement building concrete house roof
}
}
In this example, the HouseBuilder
class represents the template method with the buildHouse
method providing the algorithm's structure. The abstract methods buildFoundation
, buildWalls
, and buildRoof
define the steps to be implemented by concrete subclasses. The addExtraFeatures
method is a hook that concrete subclasses can optionally override.
Benefits of Using the Template Method Pattern
Now that we have seen a practical implementation of the Template Method pattern, let's explore the benefits it offers:
-
Code Reusability: The Template Method pattern allows you to define the skeleton of an algorithm in one place and reuse it across multiple subclasses, avoiding code duplication.
-
Flexibility: It provides flexibility by allowing subclasses to implement specific steps of an algorithm while maintaining the overall structure defined in the template method.
-
Maintenance: When the algorithm's structure needs to be modified, you can make changes in the template method, and the modifications will automatically reflect in all the subclasses.
-
Encapsulation: The Template Method pattern encapsulates the algorithm's structure, making it easier to manage and modify individual steps without affecting the overall algorithm.
Best Practices for Using the Template Method Pattern
While implementing the Template Method pattern, consider the following best practices to ensure effective and maintainable code:
1. Identify the Common Algorithm
Before applying the Template Method pattern, carefully identify the common algorithm that can be defined in the template method. Analyze the steps that are shared across multiple subclasses and those that can be customized.
2. Preserve the Algorithm's Structure
Maintain the integrity of the algorithm's structure in the template method. This ensures that the high-level algorithm is consistent across all subclasses, promoting code uniformity and readability.
3. Leverage Hooks Where Necessary
Utilize hook methods judiciously to provide extension points for subclasses. Hooks allow subclasses to participate in the algorithm at specific points, providing customization without disrupting the overall flow.
4. Document the Template Method
Clearly document the template method and its intended purpose. Describe the sequence of steps and the expectations from concrete subclasses. This documentation serves as a guide for developers working with the template method.
Closing the Chapter
In conclusion, the Template Method pattern in Java is a powerful tool for defining reusable algorithms and promoting code consistency. By leveraging this pattern, you can encapsulate the structure of an algorithm while allowing flexibility for customizations in subclasses. Understanding and applying the Template Method pattern will not only enhance the maintainability and reusability of your code but also contribute to a more structured and organized codebase.
Start implementing the Template Method pattern in your Java projects and experience the benefits of creating a robust and maintainable code structure.
Remember, mastering the Template Method pattern takes practice, but the dividends it pays in terms of code reusability and maintainability make it a skill worth mastering. Happy coding!
Now, it's your turn. Give it a try and share your experiences with the Template Method pattern in the comments below!
To read more about the Template Method design pattern, visit Refactoring Guru for an in-depth understanding.