Overcoming Dependency Injection Dilemmas in Ceylon
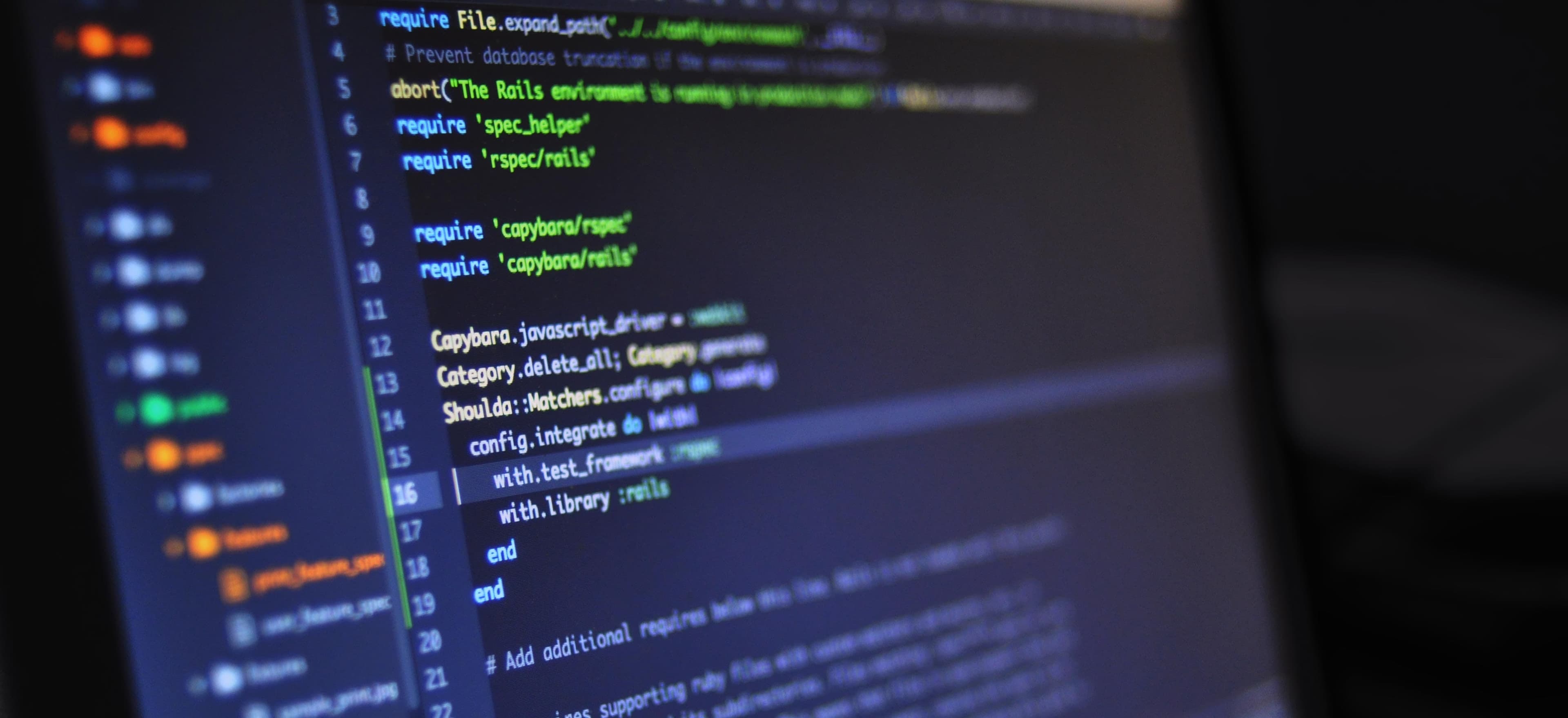
- Published on
Overcoming Dependency Injection Dilemmas in Ceylon
Dependency injection is a powerful design pattern in software engineering that allows for the creation of loosely coupled code by shifting the responsibility of obtaining the required dependencies from within the class to an external provider. In the Java ecosystem, several frameworks and libraries are available to facilitate dependency injection, such as Spring, Guice, and Dagger. However, when working with Ceylon, a modern, modular, and statically-typed language for the Java Virtual Machine, developers often encounter unique challenges when implementing dependency injection. In this blog post, we will explore these dilemmas and provide effective strategies to overcome them.
The Challenge of Dependency Injection in Ceylon
Ceylon, with its emphasis on modularity and strong typing, presents a distinctive set of challenges when it comes to dependency injection. While Java frameworks like Spring and Guice rely heavily on annotations for marking injection points and managing component dependencies, Ceylon, being a language with its syntax and semantics, demands a more tailored approach to dependency injection.
Leveraging Ceylon's Hierarchical Modular Structure
Ceylon's hierarchical modular structure provides a natural way to organize and manage dependencies within a project. By structuring modules effectively and defining clear module dependencies, developers can take advantage of Ceylon's module system to achieve dependency injection without relying on external frameworks.
// Example of module descriptor in Ceylon
module com.example.myapp "1.0.0" {
import ceylon.collection {
List
}
import com.example.mylib "1.0.0";
}
In the above example, the module descriptor explicitly states its dependencies on other modules, facilitating a clear understanding of the module's dependencies and promoting modular encapsulation.
Custom Dependency Injection in Ceylon
In scenarios where external frameworks are not preferred or feasible, custom dependency injection can be implemented in Ceylon. By creating simple and intuitive mechanisms for managing dependencies within the codebase, the need for external frameworks can be alleviated.
// Example of custom dependency injection in Ceylon
shared interface Service {
void performTask();
}
shared class MyServiceImpl() satisfies Service {
shared actual void performTask() {
// Implementation of the task
}
}
shared class MyComponent(Service service = MyServiceImpl()) {
shared Service service;
shared default new(service);
shared actual void doSomething() {
service.performTask();
}
}
In the above example, we define a Service
interface and a corresponding implementation MyServiceImpl
. The MyComponent
class then utilizes the Service
by performing the task. By setting the default implementation of the Service
interface within the MyComponent
class, we effectively achieve dependency injection without relying on external frameworks.
Leveraging Ceylon's Type System for Dependency Injection
Ceylon's powerful type system provides an excellent foundation for implementing dependency injection. By utilizing generic types and interfaces, developers can design flexible and reusable components that can be injected with different dependencies at runtime.
// Example of leveraging Ceylon's type system for dependency injection
interface Repository<DataType> {
DataType findById(Integer id);
}
class InMemoryRepository<DataType>() satisfies Repository<DataType> {
shared actual DataType findById(Integer id) {
// Implementation to retrieve data from in-memory store
}
}
class DatabaseRepository<DataType>() satisfies Repository<DataType> {
shared actual DataType findById(Integer id) {
// Implementation to retrieve data from database
}
}
class DataService<DataType>(Repository<DataType> repository) {
shared Repository<DataType> repository;
shared actual DataType fetchData(Integer id) {
return repository.findById(id);
}
}
In this example, we define a generic Repository
interface and two concrete implementations: InMemoryRepository
and DatabaseRepository
. The DataService
class utilizes the Repository
interface, allowing for flexible dependency injection of different repository implementations at runtime.
Aspect-Oriented Programming for Dependency Injection
Aspect-oriented programming (AOP) can be an effective approach for implementing cross-cutting concerns, such as logging, security, and transaction management, in a modular and reusable manner. In Ceylon, developers can leverage AOP techniques to achieve dependency injection of aspects, thereby promoting separation of concerns and enhancing code maintainability.
// Example of aspect-oriented programming for dependency injection in Ceylon
shared aspect Logging {
shared void logBefore() {
// Logging logic before method execution
}
shared void logAfter() {
// Logging logic after method execution
}
}
shared class MyService {
shared void doSomething() {
// Business logic
}
}
In this example, the Logging
aspect defines before and after advice for logging, which can be applied to the MyService
class. By applying the Logging
aspect to the MyService
class, the logging behavior is injected into the class without directly modifying its code, thus achieving dependency injection of cross-cutting concerns.
Key Takeaways
While the challenges of implementing dependency injection in Ceylon may differ from those in traditional Java applications, the language's unique features and flexibility enable developers to devise effective strategies for managing dependencies. By leveraging Ceylon's modular structure, custom dependency injection, type system, and aspect-oriented programming, developers can overcome dependency injection dilemmas and build modular, maintainable, and scalable software systems in Ceylon. Embracing these strategies will empower developers to harness the full potential of dependency injection in Ceylon and elevate the quality and robustness of their codebases.