Boost Your Pipeline: Adding Dynamic Code Analysis Easily
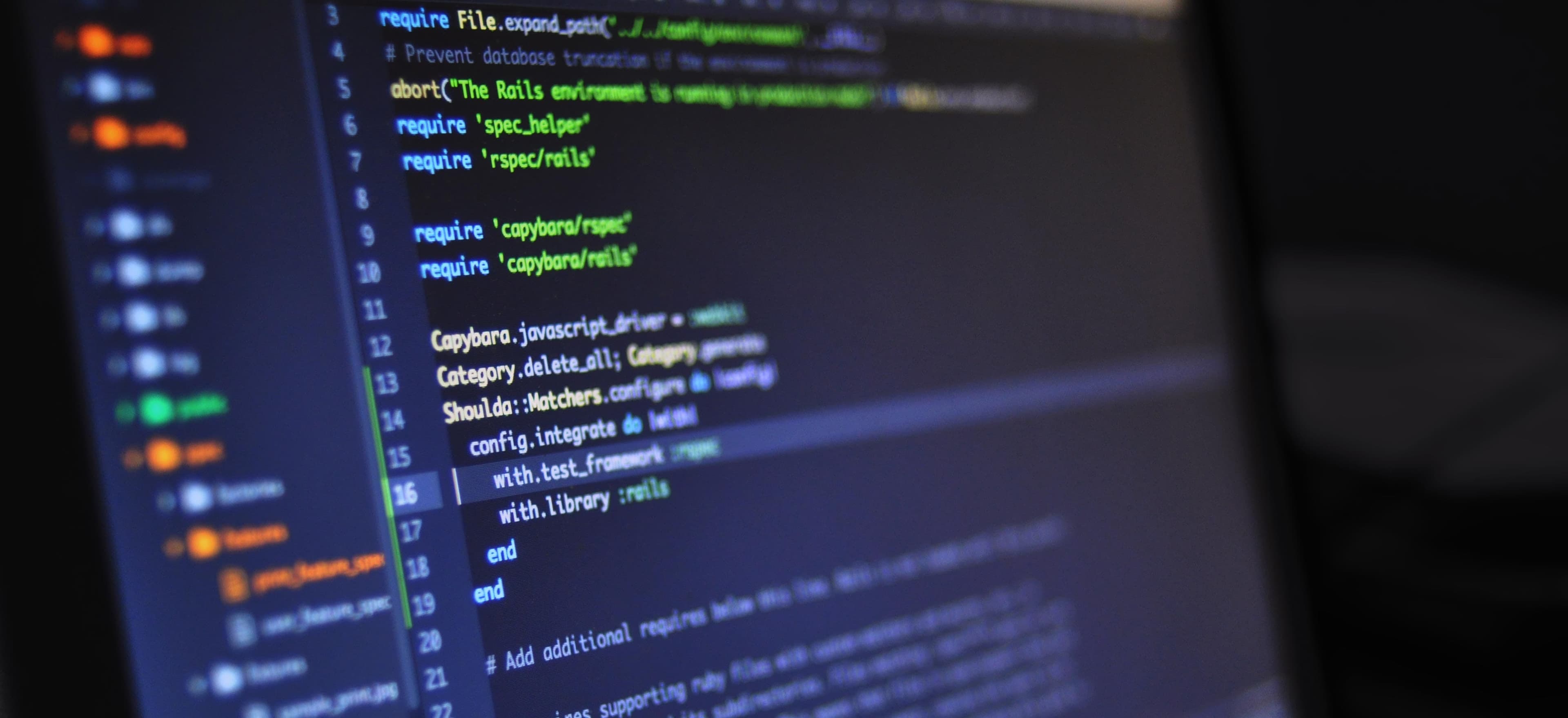
- Published on
Boost Your Pipeline: Adding Dynamic Code Analysis Easily to Your Java Projects
In today's fast-paced software development world, ensuring the quality and security of your code is paramount. Dynamic code analysis emerges as a critical tool in the developer's arsenal, enabling the identification and resolution of potential vulnerabilities and inefficiencies in Java applications. This blog post aims to demystify the process of integrating dynamic code analysis into your Java projects, ensuring a streamlined, efficient, and secure development pipeline.
Understanding Dynamic Code Analysis
Dynamic code analysis refers to the process of examining a program's behavior during its execution, as opposed to static code analysis, which inspects code without running it. This method is indispensable for identifying runtime issues such as memory leaks, threading issues, and performance bottlenecks, which static analysis might miss.
Why is it so critical for Java projects? Java, being one of the most versatile and widely used programming languages, finds its application in everything from web applications to Android apps, where efficiency and security are non-negotiable.
Integrating Dynamic Code Analysis into Your Java Projects
Integrating dynamic code analysis into your Java projects doesn't have to be an ordeal. Below, we outline a straightforward approach to incorporating a dynamic analysis tool into your workflow, exemplified with the FindBugs, a popular Java-based tool that focuses on identifying potential bugs.
Step 1: Choose the Right Tool
Choosing the right dynamic analysis tool is pivotal. FindBugs, now succeeded by SpotBugs, is an excellent starting point due to its comprehensive database of bug patterns and integration capabilities with build tools and IDEs. However, it's crucial to evaluate various tools based on your project's specific needs.
Step 2: Integration with Build Tools
Most Java projects utilize build tools like Maven or Gradle. Here's how you can integrate SpotBugs into a Maven project:
<plugin>
<groupId>com.github.spotbugs</groupId>
<artifactId>spotbugs-maven-plugin</artifactId>
<version>4.2.3</version>
<executions>
<execution>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
For Gradle projects, adding the following to your build.gradle
file will suffice:
plugins {
id "com.github.spotbugs" version "4.7.0"
}
spotbugsMain {
reports {
html.enabled = true
xml.enabled = false
}
}
This code snippet does more than just include SpotBugs in your project — it configures the tool to generate reports in HTML while disabling XML output, making the findings more accessible.
Step 3: Continuous Integration Setup
Integrating dynamic code analysis into your continuous integration (CI) pipeline ensures that every code commit is automatically examined, enhancing code quality without manual intervention. Tools like Jenkins, GitLab CI, or GitHub Actions can be employed to automate this process.
Here's an example of a GitHub Actions workflow that incorporates SpotBugs:
name: Java CI with Maven
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v2
with:
java-version: '11'
distribution: 'adopt'
- name: Build with Maven
run: mvn -B package --file pom.xml
- name: Run SpotBugs
run: mvn com.github.spotbugs:spotbugs-maven-plugin:check
This workflow not only builds your Java project using Maven but also runs SpotBugs as part of the process, ensuring that any potential issues are caught early.
Benefits of Dynamic Code Analysis in Java
- Identifies Runtime Issues: Unlike static analysis, dynamic code analysis can catch issues that only manifest during the program's execution.
- Improves Code Quality: By revealing inefficiencies and errors, dynamic code analysis aids in refining the quality of your codebase.
- Augments Security: It helps in uncovering vulnerabilities that could be exploited by malicious entities.
- Facilitates Regulatory Compliance: Certain industries require adherence to specific coding standards, which dynamic code analysis can help achieve.
Challenges and Considerations
While dynamic code analysis is immensely beneficial, it's not without its challenges. Performance overhead, false positives, and the need for test environment setup are pertinent issues. Mitigating these requires fine-tuning the analysis tool's configuration and integrating feedback into the development process continuously.
In Conclusion, Here is What Matters and Further Reading
Integrating dynamic code analysis into your Java projects significantly uplifts the quality, security, and compliance of your code. The simplicity of incorporating tools like SpotBugs into your development and CI pipelines means there's minimal excuse not to employ this powerful aid.
For those keen on diving deeper into dynamic code analysis and its applications in Java, the official SpotBugs documentation provides a wealth of information. Additionally, exploring resources such as the OWASP Secure Coding Practices can offer broader insights into securing Java applications.
By embracing dynamic code analysis, Java developers can significantly mitigate risks, enhance code quality, and streamline their development workflow, thereby ensuring the delivery of superior software products.
Checkout our other articles