Boost Your Site Speed: Master Deliberate Caching Today!
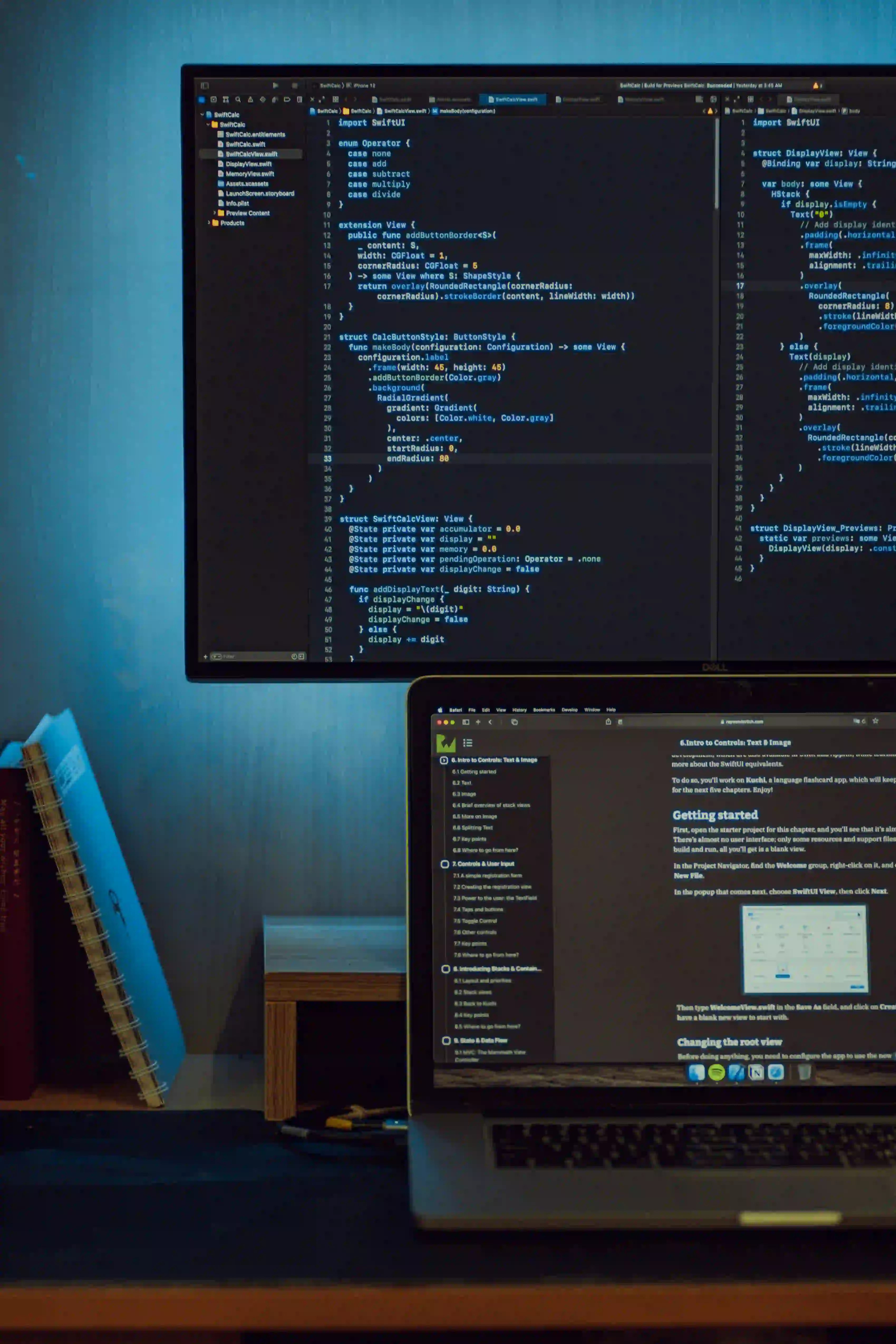
Boost Your Site Speed: Master Deliberate Caching Today!
In the competitive world of web development, site speed plays a crucial role in providing an exceptional user experience and boosting search engine rankings. Deliberate caching is an effective technique to improve site speed and optimize performance. In this article, we'll delve into the concept of deliberate caching, explore its benefits, and demonstrate how to implement it using Java.
Understanding Deliberate Caching
Deliberate caching involves storing frequently accessed data in a temporary storage area, such as memory or disk, to accelerate subsequent access to the same data. This technique reduces the need to recompute or retrieve data from its original source, thereby improving response times and overall site performance.
The key principle behind deliberate caching is to identify the data that is accessed frequently and cache it proactively, rather than relying on ad-hoc caching mechanisms. By intentionally caching specific data, developers can exert greater control over what is stored and for how long, leading to more predictable and efficient caching behavior.
Benefits of Deliberate Caching
Implementing deliberate caching in your Java applications can yield a range of benefits, including:
-
Improved Performance: By caching frequently accessed data, you can significantly reduce the response times for accessing that data, leading to faster application performance.
-
Reduced Load on Resources: Caching helps offload the computational and I/O resources by serving cached data instead of repeatedly retrieving it from the original source, thereby reducing the load on backend systems.
-
Enhanced Scalability: With deliberate caching, your application can handle increased loads more effectively, as the cached data can be readily served without taxing the backend infrastructure.
-
Consistent User Experience: Faster response times and improved performance contribute to a more consistent and satisfying user experience, leading to higher user engagement and retention.
Implementing Deliberate Caching in Java
Now, let's explore how to implement deliberate caching in Java using the popular caching library, Ehcache.
Step 1: Add Ehcache Dependency
First, you need to include the Ehcache dependency in your project. If you're using Maven, add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.4</version>
</dependency>
Step 2: Define Cache Configuration
Next, define the cache configuration where you specify the characteristics of the cache, such as its maximum size, expiration time, and eviction policy. Here's an example of creating a simple in-memory cache configuration:
CacheConfigurationBuilder<Long, String> configurationBuilder =
CacheConfigurationBuilder.newCacheConfigurationBuilder(Long.class, String.class,
ResourcePoolsBuilder.newResourcePoolsBuilder().heap(100, EntryUnit.ENTRIES));
CacheManager cacheManager =
CacheManagerBuilder.newCacheManagerBuilder().withCache("myCache", configurationBuilder).build(true);
Cache<Long, String> myCache = cacheManager.getCache("myCache", Long.class, String.class);
Step 3: Cache Data Proactively
Now that the cache is configured, you can start caching data proactively. For instance, if you have a method that retrieves user details from a database and it's a frequently accessed operation, you can cache the results using Ehcache:
public String getUserDetails(long userId) {
String userDetails = myCache.get(userId);
if (userDetails == null) {
userDetails = // Retrieve user details from the database
myCache.put(userId, userDetails);
}
return userDetails;
}
In this example, the getUserDetails
method first checks if the user details are already present in the cache. If not, it retrieves the details from the database and caches them for future access. This ensures that subsequent requests for the same user details are served from the cache, resulting in improved performance.
Step 4: Manage Cache Expiration
It's essential to manage cache expiration to prevent stale data from being served. Ehcache provides various expiration strategies, such as time-based expiration or expiration based on the number of accesses. Here's an example of setting time-based expiration for cached user details:
configurationBuilder.withExpiry(
Expirations.timeToLiveExpiration(Duration.ofMinutes(30)));
By setting a time-to-live expiration of 30 minutes, the cached user details will be automatically evicted from the cache after the specified duration, ensuring that fresh data is served to the users.
My Closing Thoughts on the Matter
Deliberate caching is a powerful technique for optimizing site speed and improving overall application performance. By leveraging deliberate caching in your Java applications, you can enhance user experience, reduce resource load, and achieve greater scalability. With the comprehensive capabilities of Ehcache, implementing deliberate caching becomes seamless and highly effective.
Incorporating deliberate caching as part of your performance optimization strategy not only benefits your users but also contributes to the success of your application in the competitive digital landscape.
Start mastering deliberate caching today and witness the transformative impact it can have on your Java applications!