Escape Monolith: A Smooth Path to Microservices Migration
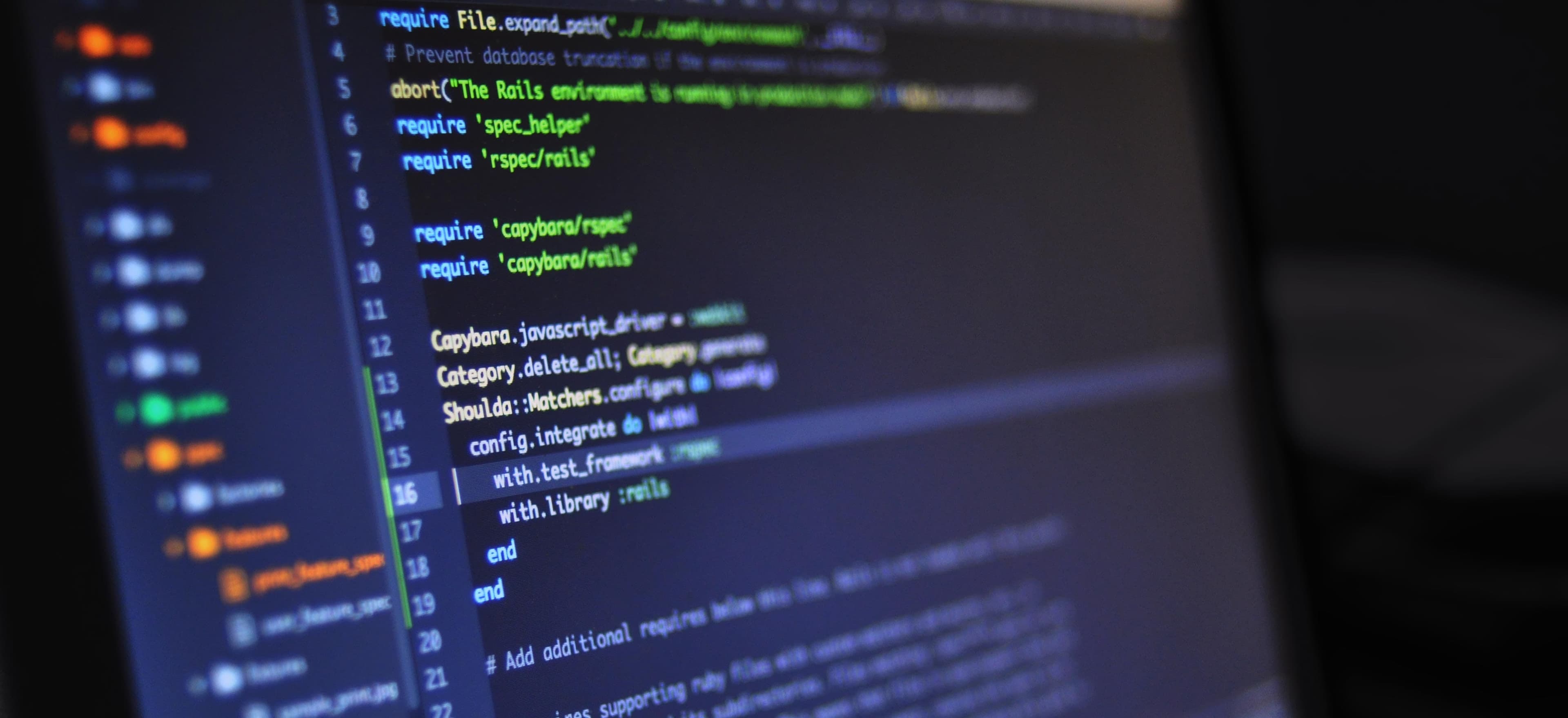
- Published on
Escape Monolith: A Smooth Path to Microservices Migration
In the fast-paced world of software development, the migration from monolithic applications to microservices architecture has become a common trend. This transition offers numerous benefits, including improved scalability, better fault isolation, and increased flexibility. However, migrating from a monolith to microservices is a complex and challenging process that requires careful planning and execution.
In this article, we will explore the key steps and best practices for migrating from a monolithic architecture to microservices using Java. We will cover various aspects of this migration, including breaking down the monolith, identifying service boundaries, choosing communication protocols, and ensuring data consistency.
Understanding the Monolith
Before diving into the migration process, it's crucial to understand the monolithic architecture that needs to be migrated. In a monolithic application, all components are tightly coupled, and any changes or updates require a full redeployment of the entire application. This lack of flexibility and scalability often becomes a bottleneck as the application grows in complexity.
Breaking Down the Monolith
The first step in the migration process is breaking down the monolithic application into smaller, more manageable components. This involves identifying distinct and cohesive areas of functionality within the monolith that can be extracted as microservices.
// Example of breaking down a monolith into separate microservices
public class CustomerService {
// ...
}
public class OrderService {
// ...
}
public class ProductService {
// ...
}
By breaking down the monolith into separate microservices, we can achieve better modularity and independent deployment of each service.
Identifying Service Boundaries
One of the most critical aspects of migrating to a microservices architecture is identifying the boundaries of each service. This involves defining clear interfaces and responsibilities for each microservice to ensure loose coupling and high cohesion.
// Example of defining service boundaries with clear interfaces
public interface ProductService {
Product getProductById(String productId);
List<Product> getProductsByCategory(String category);
// ...
}
Identifying clear service boundaries allows for independent development and deployment of microservices, and it also facilitates easier scaling and maintenance.
Choosing Communication Protocols
In a microservices architecture, communication between services is a vital aspect. Choosing the right communication protocol is crucial for ensuring reliable and efficient interaction between microservices.
Java offers various options for inter-service communication, including HTTP/REST, Messaging Queues (such as Kafka or RabbitMQ), and gRPC. The choice of communication protocol should be based on the specific requirements of the application, such as performance, reliability, and scalability.
// Example of using HTTP/REST for inter-service communication
@RequestMapping(value = "/products/{productId}", method = RequestMethod.GET)
public Product getProduct(@PathVariable String productId) {
// ...
}
By carefully selecting the appropriate communication protocol, we can ensure seamless interaction between microservices without introducing unnecessary complexities.
Ensuring Data Consistency
Maintaining data consistency is a significant challenge when transitioning from a monolithic architecture to microservices. In a monolith, the database is typically shared among all components, whereas in a microservices architecture, each service has its database.
To ensure data consistency, it is essential to carefully consider data management strategies such as the use of distributed transactions, event sourcing, or eventual consistency patterns.
// Example of applying eventual consistency pattern for data consistency
@Autowired
private ProductService productService;
public void updateProductAndNotifyOrderService(Product product) {
productService.updateProduct(product);
notifyOrderService(product.getId());
}
By employing suitable data consistency strategies, we can mitigate the challenges associated with maintaining data integrity in a distributed microservices environment.
Testing and Monitoring
As with any significant architectural change, thorough testing and monitoring are essential during the migration process. Comprehensive unit tests, integration tests, and end-to-end tests should be conducted to ensure the functionality and reliability of each microservice.
Furthermore, implementing robust monitoring and logging mechanisms is crucial for detecting and diagnosing issues within the microservices architecture.
// Example of implementing logging for monitoring and diagnostics
private static final Logger logger = LoggerFactory.getLogger(ProductService.class);
public void updateProduct(Product product) {
// ...
logger.info("Product updated: {}", product.getId());
}
By prioritizing testing and monitoring, we can minimize the risk of potential issues and ensure a smooth transition to a microservices architecture.
Key Takeaways
In conclusion, the migration from a monolithic architecture to microservices is a complex endeavor that demands careful planning and meticulous execution. By understanding the nuances of both architectures and following best practices, such as breaking down the monolith, identifying service boundaries, choosing communication protocols, ensuring data consistency, and prioritizing testing and monitoring, the migration process can be navigated smoothly.
Java, with its robust ecosystem and support for microservices frameworks like Spring Boot and Micronaut, provides an excellent platform for carrying out this migration. By leveraging the power of Java and adhering to best practices, organizations can unlock the numerous benefits of a microservices architecture while mitigating the associated challenges.
References:
- Building Microservices with Java
- Microservices Architecture: Advantages and Drawbacks
- Data Consistency in Microservices