Unlocking Location Magic: GeoHash with Redis & Spring Boot
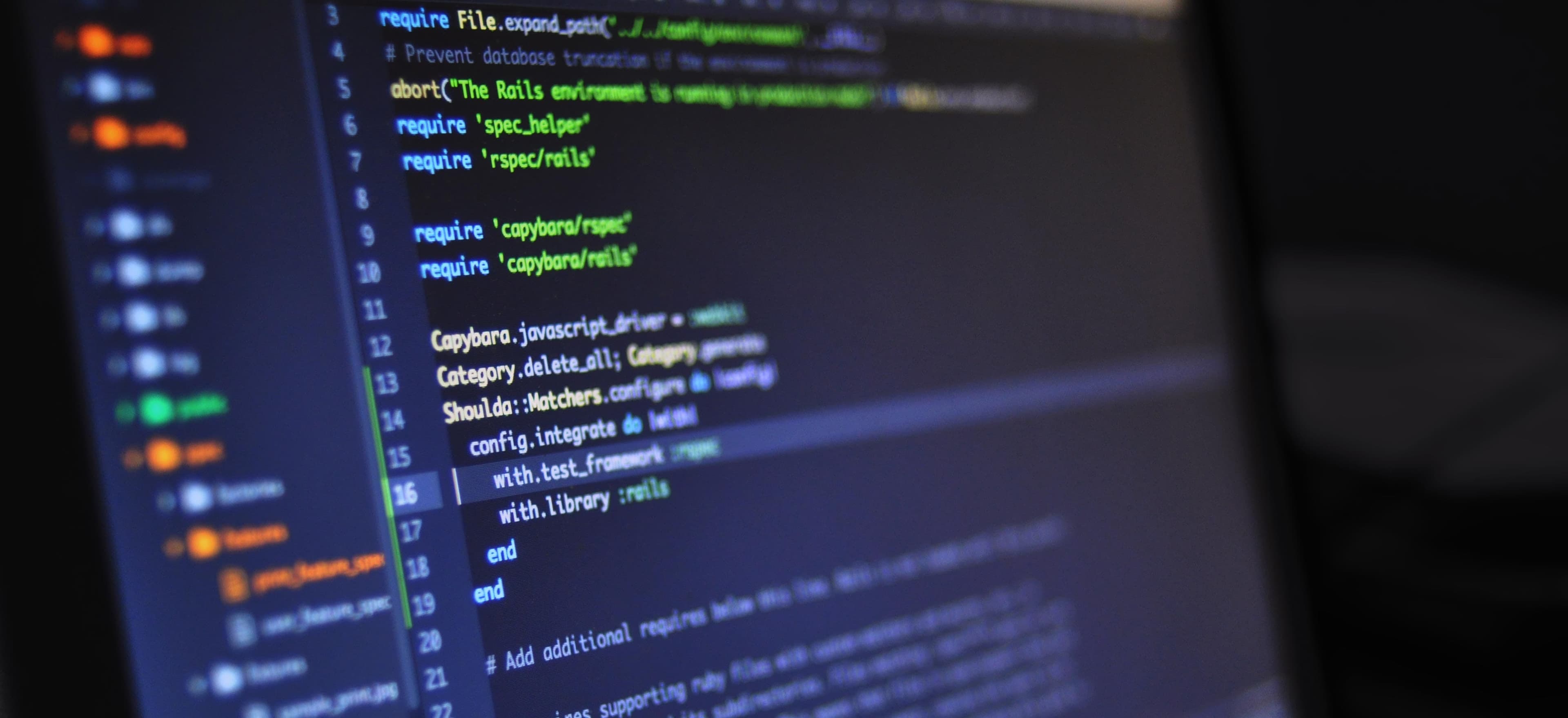
- Published on
Unlocking Location Magic: GeoHash with Redis & Spring Boot
Location-based services are becoming increasingly popular in the world of software development. Whether it's finding nearby restaurants, tracking deliveries, or creating social media check-ins, the need for location-based functionality is evident. In this blog post, we'll explore how to leverage GeoHash with Redis and Spring Boot to unlock the magic of location-based services in Java.
What is GeoHash?
GeoHash is a technique to encode geographic coordinates into a single string of text. This encoding is space-filling, meaning that nearby locations will have similar GeoHashes. This property makes GeoHash a powerful tool for proximity-based searches and calculations.
Why GeoHash with Redis?
Redis is an open-source, in-memory data structure store that is used as a database, cache, and message broker. It's known for its speed, simplicity, and versatility. By combining GeoHash with Redis, we can perform efficient spatial queries and store geospatial data with ease.
Getting Started with Spring Boot
Let's start by setting up a new Spring Boot project. You can use Spring Initializr to generate a new project with the following dependencies:
- Spring Web
- Spring Data Redis
Next, let's define a simple entity to represent a location:
import org.springframework.data.annotation.Id;
import org.springframework.data.redis.core.index.GeoIndexed;
public class Location {
@Id
private String id;
@GeoIndexed
private double latitude;
@GeoIndexed
private double longitude;
// getters and setters
}
In this Location
class, we use the @GeoIndexed
annotation provided by Spring Data Redis to mark the latitude
and longitude
fields as geospatial indexes.
Storing Locations in Redis
Now, let's create a service to manage the storage and retrieval of Location
objects in Redis:
import org.springframework.data.geo.Point;
import org.springframework.data.redis.core.ReactiveRedisTemplate;
import org.springframework.stereotype.Service;
import reactor.core.publisher.Mono;
@Service
public class LocationService {
private final ReactiveRedisTemplate<String, String> redisTemplate;
public LocationService(ReactiveRedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public Mono<Boolean> saveLocation(Location location) {
Point point = new Point(location.getLongitude(), location.getLatitude());
return redisTemplate.opsForGeo()
.add("locations", point, location.getId())
.map(count -> count == 1);
}
public Mono<Point> findLocation(String id) {
return redisTemplate.opsForGeo()
.position("locations", id)
.map(point -> {
if (point != null) {
return new Point(point.getX(), point.getY());
}
return null;
});
}
}
In this LocationService
class, we use ReactiveRedisTemplate
provided by Spring Data Redis to interact with Redis. The saveLocation
method stores a Location
as a geospatial point in a Redis Geo Set named "locations," using the latitude
and longitude
as coordinates. The findLocation
method retrieves the coordinates of a Location
based on its ID.
Performing Spatial Queries
With our locations stored in Redis, we can now perform spatial queries to find nearby locations. Let's create a controller to handle a simple proximity search:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class LocationController {
private final LocationService locationService;
public LocationController(LocationService locationService) {
this.locationService = locationService;
}
@GetMapping("/nearby")
public Mono<List<Point>> findNearbyLocations(
@RequestParam double latitude,
@RequestParam double longitude,
@RequestParam double radius
) {
return locationService.findNearbyLocations(latitude, longitude, radius);
}
}
In this LocationController
class, we define a findNearbyLocations
endpoint that takes latitude, longitude, and radius as parameters. The LocationService
will perform a spatial query to retrieve the nearby locations within the specified radius.
Bringing It All Together
Now that we have our Spring Boot application set up to work with GeoHash and Redis, we can start storing and querying locations effectively. By combining the power of GeoHash for spatial indexing and Redis for efficient data storage and retrieval, we have unlocked the magic of location-based services in Java.
Lessons Learned
In this blog post, we've explored how to leverage GeoHash with Redis and Spring Boot to enable powerful location-based services in Java. From storing geospatial data in Redis to performing spatial queries, we've demonstrated the potential of these technologies in creating location-aware applications. By understanding and harnessing the capabilities of GeoHash and Redis, developers can unlock the magic of location-based functionality in their Java applications.